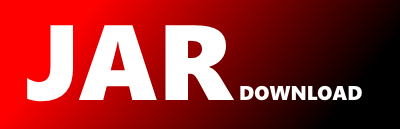
org.pitest.aggregate.DataLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pitest-aggregator Show documentation
Show all versions of pitest-aggregator Show documentation
Aggregates the output of report XML documents into a new combined HTML report
package org.pitest.aggregate;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamReader;
abstract class DataLoader {
private static final String CANNOT_CLOSE_ERR = "Unable to close input stream";
private final Set filesToLoad;
protected DataLoader(final Collection filesToLoad) {
if ((filesToLoad == null) || filesToLoad.isEmpty()) {
throw new IllegalArgumentException("Null or empty filesToLoad");
}
this.filesToLoad = Collections.unmodifiableSet(new HashSet<>(filesToLoad));
}
public Set loadData() throws ReportAggregationException {
final Set data = new HashSet<>();
for (final File file : this.filesToLoad) {
data.addAll(loadData(file));
}
return data;
}
protected abstract Set mapToData(XMLStreamReader xr) throws XMLStreamException;
Set loadData(final File dataLocation) throws ReportAggregationException {
if (!dataLocation.exists() || !dataLocation.isFile()) {
throw new ReportAggregationException(dataLocation.getAbsolutePath() + " does not exist or is not a file");
}
try {
return loadData(new BufferedInputStream(new FileInputStream(dataLocation)), dataLocation);
} catch (FileNotFoundException e) {
throw new ReportAggregationException("Could not read file: " + dataLocation.getAbsolutePath(), e);
}
}
Set loadData(final InputStream inputStream, final File dataLocation) throws ReportAggregationException {
try {
XMLInputFactory xif = XMLInputFactory.newInstance();
XMLStreamReader xr = xif.createXMLStreamReader(inputStream);
return mapToData(xr);
} catch (final XMLStreamException e) {
throw new ReportAggregationException("Could not parse file: " + dataLocation.getAbsolutePath(), e);
} finally {
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
throw new ReportAggregationException(CANNOT_CLOSE_ERR, e);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy