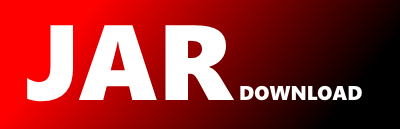
org.pkl.config.java.mapper.PMapToMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
/*
* Copyright © 2024 Apple Inc. and the Pkl project authors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.pkl.config.java.mapper;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
import java.lang.invoke.MethodType;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Map;
import java.util.Optional;
import java.util.Properties;
import java.util.function.Function;
import org.pkl.core.PClassInfo;
import org.pkl.core.util.Nullable;
class PMapToMap implements ConverterFactory {
private static final Lookup lookup = MethodHandles.lookup();
@Override
public Optional> create(PClassInfo> sourceType, Type targetType) {
var targetClass = Reflection.toRawType(targetType);
if (!(sourceType == PClassInfo.Map && Map.class.isAssignableFrom(targetClass))) {
return Optional.empty();
}
ParameterizedType mapType;
if (Properties.class.isAssignableFrom(targetClass)) {
// Properties is-a Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy