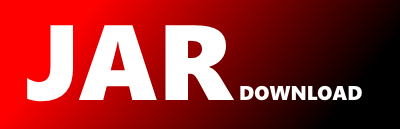
org.pkl.thirdparty.paguro.oneOf.None Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
package org.pkl.thirdparty.paguro.oneOf;
import org.pkl.thirdparty.jetbrains.annotations.NotNull;
import org.pkl.thirdparty.paguro.function.Fn0;
import org.pkl.thirdparty.paguro.function.Fn1;
/** Represents the absence of a value */
public final class None implements Option {
// For serializable. Make sure to change whenever internal data format changes.
private static final long serialVersionUID = 20170810211300L;
// ========================================== Static ==========================================
/**
* None is a singleton and this is its only instance.
* In general, you want to use {@link Option#none()} instead of
* accessing this directly so that the generic types work out.
*/
@SuppressWarnings("rawtypes")
static final Option NONE = new None();
/** Private constructor for singleton. */
private None() {}
/** {@inheritDoc} */
@Override public @NotNull T get() { throw new IllegalStateException("Called get on None"); }
/** {@inheritDoc} */
@Override public T getOrElse(T t) { return t; }
/** {@inheritDoc} */
@Override public boolean isSome() { return false; }
/** {@inheritDoc} */
@Override public U match(
@NotNull Fn1 has,
@NotNull Fn0 hasNot
) {
return hasNot.get();
}
/** {@inheritDoc} */
@Override public Option then(Fn1> f) { return Option.none(); }
/** Valid, but deprecated because it's usually an error to call this in client code. */
@Deprecated // Has no effect. Darn!
@Override public int hashCode() { return 0; }
/** Valid, but deprecated because it's usually an error to call this in client code. */
@Deprecated // Has no effect. Darn!
@Override public boolean equals(Object other) {
return other instanceof org.pkl.thirdparty.paguro.oneOf.None;
}
/** Defend our singleton property in the face of deserialization. */
private @NotNull Object readResolve() { return NONE; }
@Override
public @NotNull String toString() { return "None"; }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy