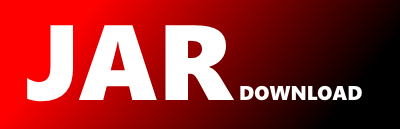
org.pkl.thirdparty.paguro.tuple.Tuple5 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
// Copyright 2016 PlanBase Inc. & Glen Peterson
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package org.pkl.thirdparty.paguro.tuple;
import org.pkl.thirdparty.jetbrains.annotations.NotNull;
import java.io.Serializable;
import java.util.Objects;
import static org.pkl.thirdparty.paguro.FunctionUtils.stringify;
// ======================================================================================
// THIS CLASS IS GENERATED BY /tupleGenerator/TupleGenerator.java. DO NOT EDIT MANUALLY!
// ======================================================================================
/**
* Holds 5 items of potentially different types. Designed to let you easily create immutable
* subclasses (to give your data structures meaningful names) with correct equals(), hashCode(), and
* toString() methods.
*/
public class Tuple5 implements Serializable {
// For serializable. Make sure to change whenever internal data format changes.
// Implemented because implementing serializable only on a subclass of an
// immutable class requires a serialization proxy. That's probably worse than
// the conceptual burden of all tuples being Serializable.
private static final long serialVersionUID = 20211228180200L;
// Fields are protected so that subclasses can make accessor methods with meaningful names.
protected final A _1;
protected final B _2;
protected final C _3;
protected final D _4;
protected final E _5;
/**
* Constructor is protected (not public) for easy inheritance. Josh Bloch's "Item 1" says public
* static factory methods are better than constructors because they have names, they can return
* an existing object instead of a new one, and they can return a subtype. Therefore, you
* have more flexibility with a static factory as part of your public API then with a public
* constructor.
*/
protected Tuple5(A a, B b, C c, D d, E e) {
_1 = a; _2 = b; _3 = c; _4 = d; _5 = e;
}
/** Public static factory method */
public static @NotNull Tuple5 of(A a, B b, C c, D d, E e) {
return new Tuple5<>(a, b, c, d, e);
}
/** Returns the 1st field */
public A _1() { return _1; }
/** Returns the 2nd field */
public B _2() { return _2; }
/** Returns the 3rd field */
public C _3() { return _3; }
/** Returns the 4th field */
public D _4() { return _4; }
/** Returns the 5th field */
public E _5() { return _5; }
@Override
public @NotNull String toString() {
return getClass().getSimpleName() + "(" +
stringify(_1) + "," + stringify(_2) + "," +
stringify(_3) + "," + stringify(_4) + "," + stringify(_5) + ")";
}
@Override
public boolean equals(Object other) {
// Cheapest operation first...
if (this == other) { return true; }
if (!(other instanceof Tuple5)) { return false; }
// Details...
@SuppressWarnings("rawtypes") final Tuple5 that = (Tuple5) other;
return Objects.equals(this._1, that._1()) &&
Objects.equals(this._2, that._2()) &&
Objects.equals(this._3, that._3()) &&
Objects.equals(this._4, that._4()) &&
Objects.equals(this._5, that._5());
}
@Override
public int hashCode() {
// First 2 fields match Tuple2 which implements java.util.Map.Entry as part of the map
// contract and therefore must match java.util.HashMap.Node.hashCode().
int ret = 0;
if (_1 != null) { ret = _1.hashCode(); }
if (_2 != null) { ret = ret ^ _2.hashCode(); }
if (_3 != null) { ret = ret + _3.hashCode(); }
if (_4 != null) { ret = ret + _4.hashCode(); }
if (_5 != null) { ret = ret + _5.hashCode(); }
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy