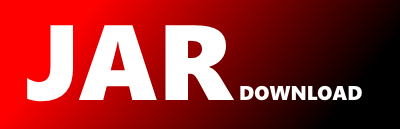
org.pkl.thirdparty.truffle.host.HostFunctionGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.host;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.TruffleLanguage;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.interop.ArityException;
import org.pkl.thirdparty.truffle.api.interop.InteropLibrary;
import org.pkl.thirdparty.truffle.api.interop.UnsupportedMessageException;
import org.pkl.thirdparty.truffle.api.interop.UnsupportedTypeException;
import org.pkl.thirdparty.truffle.api.library.DynamicDispatchLibrary;
import org.pkl.thirdparty.truffle.api.library.LibraryExport;
import org.pkl.thirdparty.truffle.api.library.LibraryFactory;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.utilities.TriState;
import org.pkl.thirdparty.truffle.host.HostFunction.IsIdenticalOrUndefined;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
@GeneratedBy(HostFunction.class)
@SuppressWarnings({"javadoc", "unused"})
final class HostFunctionGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(HostFunction.class, new InteropLibraryExports());
}
private HostFunctionGen() {
}
@GeneratedBy(HostFunction.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, HostFunction.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof HostFunction;
InteropLibrary uncached = new Uncached();
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof HostFunction;
return new Cached();
}
@GeneratedBy(HostFunction.class)
private static final class Cached extends InteropLibrary {
private static final StateField EXECUTE_EXECUTE_STATE_0_UPDATER = StateField.create(ExecuteNode_ExecuteData.lookup_(), "execute_state_0_");
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
*/
private static final HostExecuteNode INLINED_EXECUTE_NODE__EXECUTE_EXECUTE_ = HostExecuteNodeGen.inline(InlineTarget.create(HostExecuteNode.class, EXECUTE_EXECUTE_STATE_0_UPDATER.subUpdater(0, 17), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field1_", Node.class), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field2_", Object.class), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field3_", Object.class), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field4_", Node.class), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field5_", Node.class), ReferenceField.create(ExecuteNode_ExecuteData.lookup_(), "executeNode__execute_execute__field6_", Node.class)));
/**
* State Info:
* 0: SpecializationActive {@link IsIdenticalOrUndefined#doHostObject}
* 1: SpecializationActive {@link IsIdenticalOrUndefined#doOther}
*
*/
@CompilationFinal private int state_0_;
@Child private ExecuteNode_ExecuteData executeNode__execute_cache;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof HostFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof HostFunction;
}
@SuppressWarnings("static-method")
private boolean isIdenticalOrUndefinedFallbackGuard_(int state_0, HostFunction arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[HostFunction.IsIdenticalOrUndefined.doHostObject(HostFunction, HostFunction)] */) && arg1Value instanceof HostFunction) {
return false;
}
return true;
}
/**
* Debug Info:
* Specialization {@link IsIdenticalOrUndefined#doHostObject}
* Activation probability: 0.32500
* With/without class size: 7/0 bytes
* Specialization {@link IsIdenticalOrUndefined#doOther}
* Activation probability: 0.17500
* With/without class size: 6/0 bytes
*
*/
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
HostFunction arg0Value = ((HostFunction) arg0Value_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[HostFunction.IsIdenticalOrUndefined.doHostObject(HostFunction, HostFunction)] || SpecializationActive[HostFunction.IsIdenticalOrUndefined.doOther(HostFunction, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostFunction.IsIdenticalOrUndefined.doHostObject(HostFunction, HostFunction)] */ && arg1Value instanceof HostFunction) {
HostFunction arg1Value_ = (HostFunction) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostFunction.IsIdenticalOrUndefined.doOther(HostFunction, Object)] */) {
if (isIdenticalOrUndefinedFallbackGuard_(state_0, arg0Value, arg1Value)) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isIdenticalOrUndefinedAndSpecialize(arg0Value, arg1Value);
}
private TriState isIdenticalOrUndefinedAndSpecialize(HostFunction arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof HostFunction) {
HostFunction arg1Value_ = (HostFunction) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[HostFunction.IsIdenticalOrUndefined.doHostObject(HostFunction, HostFunction)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[HostFunction.IsIdenticalOrUndefined.doOther(HostFunction, Object)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@Override
public boolean isExecutable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((HostFunction) receiver)).isExecutable();
}
/**
* Debug Info:
* Specialization {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Activation probability: 0.50000
* With/without class size: 26/27 bytes
*
*/
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
HostFunction arg0Value = ((HostFunction) arg0Value_);
ExecuteNode_ExecuteData s0_ = this.executeNode__execute_cache;
if (s0_ != null) {
{
Node node__ = (s0_);
return arg0Value.execute(arg1Value, node__, INLINED_EXECUTE_NODE__EXECUTE_EXECUTE_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeNode_AndSpecialize(arg0Value, arg1Value);
}
private Object executeNode_AndSpecialize(HostFunction arg0Value, Object[] arg1Value) throws UnsupportedTypeException, ArityException {
{
Node node__ = null;
ExecuteNode_ExecuteData s0_ = this.insert(new ExecuteNode_ExecuteData());
node__ = (s0_);
VarHandle.storeStoreFence();
this.executeNode__execute_cache = s0_;
return arg0Value.execute(arg1Value, node__, INLINED_EXECUTE_NODE__EXECUTE_EXECUTE_);
}
}
@Override
public boolean hasLanguage(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((HostFunction) receiver)).hasLanguage();
}
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((HostFunction) receiver)).getLanguage();
}
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((HostFunction) receiver)).toDisplayString(allowSideEffects);
}
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return HostFunction.identityHashCode((((HostFunction) receiver)));
}
@GeneratedBy(HostFunction.class)
@DenyReplace
private static final class ExecuteNode_ExecuteData extends Node {
/**
* State Info:
* 0-16: InlinedCache
* Specialization: {@link HostFunction#execute}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int execute_state_0_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node executeNode__execute_execute__field1_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Object} field2
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object executeNode__execute_execute__field2_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Object} field3
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object executeNode__execute_execute__field3_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Node} field4
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node executeNode__execute_execute__field4_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Node} field5
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node executeNode__execute_execute__field5_;
/**
* Source Info:
* Specialization: {@link HostFunction#execute(HostFunction, Object[], Node, HostExecuteNode)}
* Parameter: {@link HostExecuteNode} execute
* Inline method: {@link HostExecuteNodeGen#inline}
* Inline field: {@link Node} field6
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node executeNode__execute_execute__field6_;
ExecuteNode_ExecuteData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
@GeneratedBy(HostFunction.class)
@DenyReplace
private static final class Uncached extends InteropLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof HostFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof HostFunction;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
HostFunction arg0Value = ((HostFunction) arg0Value_);
if (arg1Value instanceof HostFunction) {
HostFunction arg1Value_ = (HostFunction) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public boolean isExecutable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((HostFunction) receiver) .isExecutable();
}
@TruffleBoundary
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
HostFunction arg0Value = ((HostFunction) arg0Value_);
return arg0Value.execute(arg1Value, (this), (HostExecuteNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public boolean hasLanguage(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((HostFunction) receiver) .hasLanguage();
}
@TruffleBoundary
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((HostFunction) receiver) .getLanguage();
}
@TruffleBoundary
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((HostFunction) receiver) .toDisplayString(allowSideEffects);
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return HostFunction.identityHashCode(((HostFunction) receiver) );
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy