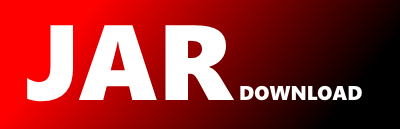
org.pkl.thirdparty.truffle.host.HostObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.host;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport;
import org.pkl.thirdparty.truffle.api.dsl.NeverDefault;
import org.pkl.thirdparty.truffle.api.dsl.UnsupportedSpecializationException;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.RequiredField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.interop.UnknownIdentifierException;
import org.pkl.thirdparty.truffle.api.interop.UnsupportedTypeException;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.ExplodeLoop;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.profiles.InlinedBranchProfile;
import org.pkl.thirdparty.truffle.host.HostContext.ToGuestValueNode;
import org.pkl.thirdparty.truffle.host.HostContextFactory.ToGuestValueNodeGen;
import org.pkl.thirdparty.truffle.host.HostObject.ArrayGet;
import org.pkl.thirdparty.truffle.host.HostObject.ArraySet;
import org.pkl.thirdparty.truffle.host.HostObject.ContainsKeyNode;
import org.pkl.thirdparty.truffle.host.HostObject.LookupConstructorNode;
import org.pkl.thirdparty.truffle.host.HostObject.LookupFieldNode;
import org.pkl.thirdparty.truffle.host.HostObject.LookupFunctionalMethodNode;
import org.pkl.thirdparty.truffle.host.HostObject.LookupInnerClassNode;
import org.pkl.thirdparty.truffle.host.HostObject.LookupMethodNode;
import org.pkl.thirdparty.truffle.host.HostObject.ReadFieldNode;
import org.pkl.thirdparty.truffle.host.HostObject.WriteFieldNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
@GeneratedBy(HostObject.class)
@SuppressWarnings("javadoc")
final class HostObjectFactory {
/**
* Debug Info:
* Specialization {@link ArraySet#doBoolean}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link ArraySet#doByte}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link ArraySet#doShort}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link ArraySet#doChar}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link ArraySet#doInt}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link ArraySet#doLong}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link ArraySet#doFloat}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link ArraySet#doDouble}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link ArraySet#doObject}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(ArraySet.class)
@SuppressWarnings("javadoc")
static final class ArraySetNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static ArraySet getUncached() {
return ArraySetNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static ArraySet inline(@RequiredField(bits = 9, value = StateField.class) InlineTarget target) {
return new ArraySetNodeGen.Inlined(target);
}
@GeneratedBy(ArraySet.class)
@DenyReplace
private static final class Inlined extends ArraySet {
/**
* State Info:
* 0: SpecializationActive {@link ArraySet#doBoolean}
* 1: SpecializationActive {@link ArraySet#doByte}
* 2: SpecializationActive {@link ArraySet#doShort}
* 3: SpecializationActive {@link ArraySet#doChar}
* 4: SpecializationActive {@link ArraySet#doInt}
* 5: SpecializationActive {@link ArraySet#doLong}
* 6: SpecializationActive {@link ArraySet#doFloat}
* 7: SpecializationActive {@link ArraySet#doDouble}
* 8: SpecializationActive {@link ArraySet#doObject}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ArraySet.class);
this.state_0_ = target.getState(0, 9);
}
@Override
protected void execute(Node arg0Value, Object arg1Value, int arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.ArraySet.doBoolean(boolean[], int, boolean)] || SpecializationActive[HostObject.ArraySet.doByte(byte[], int, byte)] || SpecializationActive[HostObject.ArraySet.doShort(short[], int, short)] || SpecializationActive[HostObject.ArraySet.doChar(char[], int, char)] || SpecializationActive[HostObject.ArraySet.doInt(int[], int, int)] || SpecializationActive[HostObject.ArraySet.doLong(long[], int, long)] || SpecializationActive[HostObject.ArraySet.doFloat(float[], int, float)] || SpecializationActive[HostObject.ArraySet.doDouble(double[], int, double)] || SpecializationActive[HostObject.ArraySet.doObject(Object[], int, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.ArraySet.doBoolean(boolean[], int, boolean)] */ && arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
if (arg3Value instanceof Boolean) {
boolean arg3Value_ = (boolean) arg3Value;
ArraySet.doBoolean(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.ArraySet.doByte(byte[], int, byte)] */ && arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
if (arg3Value instanceof Byte) {
byte arg3Value_ = (byte) arg3Value;
ArraySet.doByte(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[HostObject.ArraySet.doShort(short[], int, short)] */ && arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
if (arg3Value instanceof Short) {
short arg3Value_ = (short) arg3Value;
ArraySet.doShort(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[HostObject.ArraySet.doChar(char[], int, char)] */ && arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
if (arg3Value instanceof Character) {
char arg3Value_ = (char) arg3Value;
ArraySet.doChar(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[HostObject.ArraySet.doInt(int[], int, int)] */ && arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
ArraySet.doInt(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[HostObject.ArraySet.doLong(long[], int, long)] */ && arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
if (arg3Value instanceof Long) {
long arg3Value_ = (long) arg3Value;
ArraySet.doLong(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[HostObject.ArraySet.doFloat(float[], int, float)] */ && arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
if (arg3Value instanceof Float) {
float arg3Value_ = (float) arg3Value;
ArraySet.doFloat(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[HostObject.ArraySet.doDouble(double[], int, double)] */ && arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
if (arg3Value instanceof Double) {
double arg3Value_ = (double) arg3Value;
ArraySet.doDouble(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[HostObject.ArraySet.doObject(Object[], int, Object)] */ && arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
ArraySet.doObject(arg1Value_, arg2Value, arg3Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void executeAndSpecialize(Node arg0Value, Object arg1Value, int arg2Value, Object arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
if (arg3Value instanceof Boolean) {
boolean arg3Value_ = (boolean) arg3Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.ArraySet.doBoolean(boolean[], int, boolean)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doBoolean(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
if (arg3Value instanceof Byte) {
byte arg3Value_ = (byte) arg3Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.ArraySet.doByte(byte[], int, byte)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doByte(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
if (arg3Value instanceof Short) {
short arg3Value_ = (short) arg3Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[HostObject.ArraySet.doShort(short[], int, short)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doShort(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
if (arg3Value instanceof Character) {
char arg3Value_ = (char) arg3Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[HostObject.ArraySet.doChar(char[], int, char)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doChar(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[HostObject.ArraySet.doInt(int[], int, int)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doInt(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
if (arg3Value instanceof Long) {
long arg3Value_ = (long) arg3Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[HostObject.ArraySet.doLong(long[], int, long)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doLong(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
if (arg3Value instanceof Float) {
float arg3Value_ = (float) arg3Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[HostObject.ArraySet.doFloat(float[], int, float)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doFloat(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
if (arg3Value instanceof Double) {
double arg3Value_ = (double) arg3Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[HostObject.ArraySet.doDouble(double[], int, double)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doDouble(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[HostObject.ArraySet.doObject(Object[], int, Object)] */;
this.state_0_.set(arg0Value, state_0);
ArraySet.doObject(arg1Value_, arg2Value, arg3Value);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ArraySet.class)
@DenyReplace
private static final class Uncached extends ArraySet {
@TruffleBoundary
@Override
protected void execute(Node arg0Value, Object arg1Value, int arg2Value, Object arg3Value) {
if (arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
if (arg3Value instanceof Boolean) {
boolean arg3Value_ = (boolean) arg3Value;
ArraySet.doBoolean(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
if (arg3Value instanceof Byte) {
byte arg3Value_ = (byte) arg3Value;
ArraySet.doByte(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
if (arg3Value instanceof Short) {
short arg3Value_ = (short) arg3Value;
ArraySet.doShort(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
if (arg3Value instanceof Character) {
char arg3Value_ = (char) arg3Value;
ArraySet.doChar(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
if (arg3Value instanceof Integer) {
int arg3Value_ = (int) arg3Value;
ArraySet.doInt(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
if (arg3Value instanceof Long) {
long arg3Value_ = (long) arg3Value;
ArraySet.doLong(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
if (arg3Value instanceof Float) {
float arg3Value_ = (float) arg3Value;
ArraySet.doFloat(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
if (arg3Value instanceof Double) {
double arg3Value_ = (double) arg3Value;
ArraySet.doDouble(arg1Value_, arg2Value, arg3Value_);
return;
}
}
if (arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
ArraySet.doObject(arg1Value_, arg2Value, arg3Value);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ArrayGet#doBoolean}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link ArrayGet#doByte}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link ArrayGet#doShort}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link ArrayGet#doChar}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link ArrayGet#doInt}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link ArrayGet#doLong}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link ArrayGet#doFloat}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link ArrayGet#doDouble}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link ArrayGet#doObject}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(ArrayGet.class)
@SuppressWarnings("javadoc")
static final class ArrayGetNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static ArrayGet getUncached() {
return ArrayGetNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static ArrayGet inline(@RequiredField(bits = 9, value = StateField.class) InlineTarget target) {
return new ArrayGetNodeGen.Inlined(target);
}
@GeneratedBy(ArrayGet.class)
@DenyReplace
private static final class Inlined extends ArrayGet {
/**
* State Info:
* 0: SpecializationActive {@link ArrayGet#doBoolean}
* 1: SpecializationActive {@link ArrayGet#doByte}
* 2: SpecializationActive {@link ArrayGet#doShort}
* 3: SpecializationActive {@link ArrayGet#doChar}
* 4: SpecializationActive {@link ArrayGet#doInt}
* 5: SpecializationActive {@link ArrayGet#doLong}
* 6: SpecializationActive {@link ArrayGet#doFloat}
* 7: SpecializationActive {@link ArrayGet#doDouble}
* 8: SpecializationActive {@link ArrayGet#doObject}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ArrayGet.class);
this.state_0_ = target.getState(0, 9);
}
@Override
protected Object execute(Node arg0Value, Object arg1Value, int arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.ArrayGet.doBoolean(boolean[], int)] || SpecializationActive[HostObject.ArrayGet.doByte(byte[], int)] || SpecializationActive[HostObject.ArrayGet.doShort(short[], int)] || SpecializationActive[HostObject.ArrayGet.doChar(char[], int)] || SpecializationActive[HostObject.ArrayGet.doInt(int[], int)] || SpecializationActive[HostObject.ArrayGet.doLong(long[], int)] || SpecializationActive[HostObject.ArrayGet.doFloat(float[], int)] || SpecializationActive[HostObject.ArrayGet.doDouble(double[], int)] || SpecializationActive[HostObject.ArrayGet.doObject(Object[], int)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.ArrayGet.doBoolean(boolean[], int)] */ && arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
return ArrayGet.doBoolean(arg1Value_, arg2Value);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.ArrayGet.doByte(byte[], int)] */ && arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
return ArrayGet.doByte(arg1Value_, arg2Value);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[HostObject.ArrayGet.doShort(short[], int)] */ && arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
return ArrayGet.doShort(arg1Value_, arg2Value);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doChar(char[], int)] */ && arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
return ArrayGet.doChar(arg1Value_, arg2Value);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doInt(int[], int)] */ && arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
return ArrayGet.doInt(arg1Value_, arg2Value);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doLong(long[], int)] */ && arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
return ArrayGet.doLong(arg1Value_, arg2Value);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doFloat(float[], int)] */ && arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
return ArrayGet.doFloat(arg1Value_, arg2Value);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doDouble(double[], int)] */ && arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
return ArrayGet.doDouble(arg1Value_, arg2Value);
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[HostObject.ArrayGet.doObject(Object[], int)] */ && arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
return ArrayGet.doObject(arg1Value_, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Node arg0Value, Object arg1Value, int arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.ArrayGet.doBoolean(boolean[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doBoolean(arg1Value_, arg2Value);
}
if (arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.ArrayGet.doByte(byte[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doByte(arg1Value_, arg2Value);
}
if (arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[HostObject.ArrayGet.doShort(short[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doShort(arg1Value_, arg2Value);
}
if (arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[HostObject.ArrayGet.doChar(char[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doChar(arg1Value_, arg2Value);
}
if (arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[HostObject.ArrayGet.doInt(int[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doInt(arg1Value_, arg2Value);
}
if (arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[HostObject.ArrayGet.doLong(long[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doLong(arg1Value_, arg2Value);
}
if (arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[HostObject.ArrayGet.doFloat(float[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doFloat(arg1Value_, arg2Value);
}
if (arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[HostObject.ArrayGet.doDouble(double[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doDouble(arg1Value_, arg2Value);
}
if (arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[HostObject.ArrayGet.doObject(Object[], int)] */;
this.state_0_.set(arg0Value, state_0);
return ArrayGet.doObject(arg1Value_, arg2Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ArrayGet.class)
@DenyReplace
private static final class Uncached extends ArrayGet {
@TruffleBoundary
@Override
protected Object execute(Node arg0Value, Object arg1Value, int arg2Value) {
if (arg1Value instanceof boolean[]) {
boolean[] arg1Value_ = (boolean[]) arg1Value;
return ArrayGet.doBoolean(arg1Value_, arg2Value);
}
if (arg1Value instanceof byte[]) {
byte[] arg1Value_ = (byte[]) arg1Value;
return ArrayGet.doByte(arg1Value_, arg2Value);
}
if (arg1Value instanceof short[]) {
short[] arg1Value_ = (short[]) arg1Value;
return ArrayGet.doShort(arg1Value_, arg2Value);
}
if (arg1Value instanceof char[]) {
char[] arg1Value_ = (char[]) arg1Value;
return ArrayGet.doChar(arg1Value_, arg2Value);
}
if (arg1Value instanceof int[]) {
int[] arg1Value_ = (int[]) arg1Value;
return ArrayGet.doInt(arg1Value_, arg2Value);
}
if (arg1Value instanceof long[]) {
long[] arg1Value_ = (long[]) arg1Value;
return ArrayGet.doLong(arg1Value_, arg2Value);
}
if (arg1Value instanceof float[]) {
float[] arg1Value_ = (float[]) arg1Value;
return ArrayGet.doFloat(arg1Value_, arg2Value);
}
if (arg1Value instanceof double[]) {
double[] arg1Value_ = (double[]) arg1Value;
return ArrayGet.doDouble(arg1Value_, arg2Value);
}
if (arg1Value instanceof Object[]) {
Object[] arg1Value_ = (Object[]) arg1Value;
return ArrayGet.doObject(arg1Value_, arg2Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link LookupConstructorNode#doCached}
* Activation probability: 0.65000
* With/without class size: 19/8 bytes
* Specialization {@link LookupConstructorNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(LookupConstructorNode.class)
@SuppressWarnings("javadoc")
static final class LookupConstructorNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LookupConstructorNode getUncached() {
return LookupConstructorNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static LookupConstructorNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new LookupConstructorNodeGen.Inlined(target);
}
@GeneratedBy(LookupConstructorNode.class)
@DenyReplace
private static final class Inlined extends LookupConstructorNode {
/**
* State Info:
* 0: SpecializationActive {@link LookupConstructorNode#doCached}
* 1: SpecializationActive {@link LookupConstructorNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LookupConstructorNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.LookupConstructorNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] || SpecializationActive[HostObject.LookupConstructorNode.doUncached(HostObject, Class<>)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.LookupConstructorNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg2Value == s0_.cachedClazz_)) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedMethod_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.LookupConstructorNode.doUncached(HostObject, Class<>)] */) {
return doUncached(arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private HostMethodDesc executeAndSpecialize(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.LookupConstructorNode.doUncached(HostObject, Class<>)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg2Value == s0_.cachedClazz_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg2Value == s0_.cachedClazz_);
if (count0_ < (LookupConstructorNode.LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.cachedClazz_ = (arg2Value);
s0_.cachedMethod_ = (doUncached(arg1Value, arg2Value));
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.LookupConstructorNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedMethod_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.LookupConstructorNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.LookupConstructorNode.doUncached(HostObject, Class<>)] */;
this.state_0_.set(arg0Value, state_0);
return doUncached(arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LookupConstructorNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link LookupConstructorNode#doCached}
* Parameter: {@link Class} cachedClazz
*/
@CompilationFinal Class> cachedClazz_;
/**
* Source Info:
* Specialization: {@link LookupConstructorNode#doCached}
* Parameter: {@link HostMethodDesc} cachedMethod
*/
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupConstructorNode.class)
@DenyReplace
private static final class Uncached extends LookupConstructorNode {
@TruffleBoundary
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
return doUncached(arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link LookupFieldNode#doCached}
* Activation probability: 0.65000
* With/without class size: 22/13 bytes
* Specialization {@link LookupFieldNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(LookupFieldNode.class)
@SuppressWarnings("javadoc")
static final class LookupFieldNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LookupFieldNode getUncached() {
return LookupFieldNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static LookupFieldNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new LookupFieldNodeGen.Inlined(target);
}
@GeneratedBy(LookupFieldNode.class)
@DenyReplace
private static final class Inlined extends LookupFieldNode {
/**
* State Info:
* 0: SpecializationActive {@link LookupFieldNode#doCached}
* 1: SpecializationActive {@link LookupFieldNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LookupFieldNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
public HostFieldDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.LookupFieldNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc)] || SpecializationActive[HostObject.LookupFieldNode.doUncached(HostObject, Class<>, String, boolean)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.LookupFieldNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg4Value == s0_.cachedStatic_) && (arg2Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg3Value))) {
return doCached(arg1Value, arg2Value, arg3Value, arg4Value, s0_.cachedStatic_, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedField_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.LookupFieldNode.doUncached(HostObject, Class<>, String, boolean)] */) {
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private HostFieldDesc executeAndSpecialize(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.LookupFieldNode.doUncached(HostObject, Class<>, String, boolean)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg4Value == s0_.cachedStatic_) && (arg2Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg3Value))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg4Value == s0_.cachedStatic_);
// assert (arg2Value == s0_.cachedClazz_);
// assert (s0_.cachedName_.equals(arg3Value));
if (count0_ < (LookupFieldNode.LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.cachedStatic_ = (arg4Value);
s0_.cachedClazz_ = (arg2Value);
s0_.cachedName_ = (arg3Value);
s0_.cachedField_ = (doUncached(arg1Value, arg2Value, arg3Value, arg4Value));
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.LookupFieldNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, arg3Value, arg4Value, s0_.cachedStatic_, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedField_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.LookupFieldNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostFieldDesc)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.LookupFieldNode.doUncached(HostObject, Class<>, String, boolean)] */;
this.state_0_.set(arg0Value, state_0);
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LookupFieldNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link LookupFieldNode#doCached}
* Parameter: boolean cachedStatic
*/
@CompilationFinal boolean cachedStatic_;
/**
* Source Info:
* Specialization: {@link LookupFieldNode#doCached}
* Parameter: {@link Class} cachedClazz
*/
@CompilationFinal Class> cachedClazz_;
/**
* Source Info:
* Specialization: {@link LookupFieldNode#doCached}
* Parameter: {@link String} cachedName
*/
@CompilationFinal String cachedName_;
/**
* Source Info:
* Specialization: {@link LookupFieldNode#doCached}
* Parameter: {@link HostFieldDesc} cachedField
*/
@CompilationFinal HostFieldDesc cachedField_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupFieldNode.class)
@DenyReplace
private static final class Uncached extends LookupFieldNode {
@TruffleBoundary
@Override
public HostFieldDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link LookupFunctionalMethodNode#doCached}
* Activation probability: 0.65000
* With/without class size: 19/8 bytes
* Specialization {@link LookupFunctionalMethodNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(LookupFunctionalMethodNode.class)
@SuppressWarnings("javadoc")
static final class LookupFunctionalMethodNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LookupFunctionalMethodNode getUncached() {
return LookupFunctionalMethodNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static LookupFunctionalMethodNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new LookupFunctionalMethodNodeGen.Inlined(target);
}
@GeneratedBy(LookupFunctionalMethodNode.class)
@DenyReplace
private static final class Inlined extends LookupFunctionalMethodNode {
/**
* State Info:
* 0: SpecializationActive {@link LookupFunctionalMethodNode#doCached}
* 1: SpecializationActive {@link LookupFunctionalMethodNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LookupFunctionalMethodNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.LookupFunctionalMethodNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] || SpecializationActive[HostObject.LookupFunctionalMethodNode.doUncached(HostObject, Class<>)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.LookupFunctionalMethodNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg2Value == s0_.cachedClazz_)) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedMethod_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.LookupFunctionalMethodNode.doUncached(HostObject, Class<>)] */) {
return LookupFunctionalMethodNode.doUncached(arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private HostMethodDesc executeAndSpecialize(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.LookupFunctionalMethodNode.doUncached(HostObject, Class<>)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg2Value == s0_.cachedClazz_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg2Value == s0_.cachedClazz_);
if (count0_ < (LookupFunctionalMethodNode.LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.cachedClazz_ = (arg2Value);
s0_.cachedMethod_ = (LookupFunctionalMethodNode.doUncached(arg1Value, arg2Value));
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.LookupFunctionalMethodNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedMethod_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.LookupFunctionalMethodNode.doCached(HostObject, Class<>, Class<>, HostMethodDesc)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.LookupFunctionalMethodNode.doUncached(HostObject, Class<>)] */;
this.state_0_.set(arg0Value, state_0);
return LookupFunctionalMethodNode.doUncached(arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LookupFunctionalMethodNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link LookupFunctionalMethodNode#doCached}
* Parameter: {@link Class} cachedClazz
*/
@CompilationFinal Class> cachedClazz_;
/**
* Source Info:
* Specialization: {@link LookupFunctionalMethodNode#doCached}
* Parameter: {@link HostMethodDesc} cachedMethod
*/
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupFunctionalMethodNode.class)
@DenyReplace
private static final class Uncached extends LookupFunctionalMethodNode {
@TruffleBoundary
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value) {
return LookupFunctionalMethodNode.doUncached(arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link LookupInnerClassNode#doCached}
* Activation probability: 0.65000
* With/without class size: 22/12 bytes
* Specialization {@link LookupInnerClassNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(LookupInnerClassNode.class)
@SuppressWarnings("javadoc")
static final class LookupInnerClassNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LookupInnerClassNode getUncached() {
return LookupInnerClassNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static LookupInnerClassNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new LookupInnerClassNodeGen.Inlined(target);
}
@GeneratedBy(LookupInnerClassNode.class)
@DenyReplace
private static final class Inlined extends LookupInnerClassNode {
/**
* State Info:
* 0: SpecializationActive {@link LookupInnerClassNode#doCached}
* 1: SpecializationActive {@link LookupInnerClassNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LookupInnerClassNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
public Class> execute(Node arg0Value, Class> arg1Value, String arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.LookupInnerClassNode.doCached(Class<>, String, Class<>, String, Class<>)] || SpecializationActive[HostObject.LookupInnerClassNode.doUncached(Class<>, String)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.LookupInnerClassNode.doCached(Class<>, String, Class<>, String, Class<>)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg1Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg2Value))) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedInnerClass_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.LookupInnerClassNode.doUncached(Class<>, String)] */) {
return doUncached(arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Class> executeAndSpecialize(Node arg0Value, Class> arg1Value, String arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.LookupInnerClassNode.doUncached(Class<>, String)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg1Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg2Value))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg1Value == s0_.cachedClazz_);
// assert (s0_.cachedName_.equals(arg2Value));
if (count0_ < (LookupInnerClassNode.LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.cachedClazz_ = (arg1Value);
s0_.cachedName_ = (arg2Value);
s0_.cachedInnerClass_ = (doUncached(arg1Value, arg2Value));
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.LookupInnerClassNode.doCached(Class<>, String, Class<>, String, Class<>)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedInnerClass_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.LookupInnerClassNode.doCached(Class<>, String, Class<>, String, Class<>)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.LookupInnerClassNode.doUncached(Class<>, String)] */;
this.state_0_.set(arg0Value, state_0);
return doUncached(arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LookupInnerClassNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link LookupInnerClassNode#doCached}
* Parameter: {@link Class} cachedClazz
*/
@CompilationFinal Class> cachedClazz_;
/**
* Source Info:
* Specialization: {@link LookupInnerClassNode#doCached}
* Parameter: {@link String} cachedName
*/
@CompilationFinal String cachedName_;
/**
* Source Info:
* Specialization: {@link LookupInnerClassNode#doCached}
* Parameter: {@link Class} cachedInnerClass
*/
@CompilationFinal Class> cachedInnerClass_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupInnerClassNode.class)
@DenyReplace
private static final class Uncached extends LookupInnerClassNode {
@TruffleBoundary
@Override
public Class> execute(Node arg0Value, Class> arg1Value, String arg2Value) {
return doUncached(arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link LookupMethodNode#doCached}
* Activation probability: 0.65000
* With/without class size: 22/13 bytes
* Specialization {@link LookupMethodNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(LookupMethodNode.class)
@SuppressWarnings("javadoc")
static final class LookupMethodNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static LookupMethodNode getUncached() {
return LookupMethodNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static LookupMethodNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new LookupMethodNodeGen.Inlined(target);
}
@GeneratedBy(LookupMethodNode.class)
@DenyReplace
private static final class Inlined extends LookupMethodNode {
/**
* State Info:
* 0: SpecializationActive {@link LookupMethodNode#doCached}
* 1: SpecializationActive {@link LookupMethodNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(LookupMethodNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.LookupMethodNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc)] || SpecializationActive[HostObject.LookupMethodNode.doUncached(HostObject, Class<>, String, boolean)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.LookupMethodNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg4Value == s0_.cachedStatic_) && (arg2Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg3Value))) {
return doCached(arg1Value, arg2Value, arg3Value, arg4Value, s0_.cachedStatic_, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedMethod_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.LookupMethodNode.doUncached(HostObject, Class<>, String, boolean)] */) {
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private HostMethodDesc executeAndSpecialize(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.LookupMethodNode.doUncached(HostObject, Class<>, String, boolean)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg4Value == s0_.cachedStatic_) && (arg2Value == s0_.cachedClazz_) && (s0_.cachedName_.equals(arg3Value))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg4Value == s0_.cachedStatic_);
// assert (arg2Value == s0_.cachedClazz_);
// assert (s0_.cachedName_.equals(arg3Value));
if (count0_ < (LookupMethodNode.LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.cachedStatic_ = (arg4Value);
s0_.cachedClazz_ = (arg2Value);
s0_.cachedName_ = (arg3Value);
s0_.cachedMethod_ = (doUncached(arg1Value, arg2Value, arg3Value, arg4Value));
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.LookupMethodNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, arg3Value, arg4Value, s0_.cachedStatic_, s0_.cachedClazz_, s0_.cachedName_, s0_.cachedMethod_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.LookupMethodNode.doCached(HostObject, Class<>, String, boolean, boolean, Class<>, String, HostMethodDesc)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.LookupMethodNode.doUncached(HostObject, Class<>, String, boolean)] */;
this.state_0_.set(arg0Value, state_0);
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(LookupMethodNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link LookupMethodNode#doCached}
* Parameter: boolean cachedStatic
*/
@CompilationFinal boolean cachedStatic_;
/**
* Source Info:
* Specialization: {@link LookupMethodNode#doCached}
* Parameter: {@link Class} cachedClazz
*/
@CompilationFinal Class> cachedClazz_;
/**
* Source Info:
* Specialization: {@link LookupMethodNode#doCached}
* Parameter: {@link String} cachedName
*/
@CompilationFinal String cachedName_;
/**
* Source Info:
* Specialization: {@link LookupMethodNode#doCached}
* Parameter: {@link HostMethodDesc} cachedMethod
*/
@CompilationFinal HostMethodDesc cachedMethod_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(LookupMethodNode.class)
@DenyReplace
private static final class Uncached extends LookupMethodNode {
@TruffleBoundary
@Override
public HostMethodDesc execute(Node arg0Value, HostObject arg1Value, Class> arg2Value, String arg3Value, boolean arg4Value) {
return doUncached(arg1Value, arg2Value, arg3Value, arg4Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ReadFieldNode#doCached}
* Activation probability: 0.65000
* With/without class size: 24/9 bytes
* Specialization {@link ReadFieldNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(ReadFieldNode.class)
@SuppressWarnings("javadoc")
static final class ReadFieldNodeGen {
private static final StateField CACHED_CACHED_STATE_0_UPDATER = StateField.create(CachedData.lookup_(), "cached_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static ReadFieldNode getUncached() {
return ReadFieldNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static ReadFieldNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new ReadFieldNodeGen.Inlined(target);
}
@GeneratedBy(ReadFieldNode.class)
@DenyReplace
private static final class Inlined extends ReadFieldNode {
/**
* State Info:
* 0: SpecializationActive {@link ReadFieldNode#doCached}
* 1: SpecializationActive {@link ReadFieldNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
/**
* Source Info:
* Specialization: {@link ReadFieldNode#doCached}
* Parameter: {@link ToGuestValueNode} toGuest
* Inline method: {@link ToGuestValueNodeGen#inline}
*/
private final ToGuestValueNode cached_toGuest_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ReadFieldNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
this.cached_toGuest_ = ToGuestValueNodeGen.inline(InlineTarget.create(ToGuestValueNode.class, CACHED_CACHED_STATE_0_UPDATER.subUpdater(0, 3), ReferenceField.create(CachedData.lookup_(), "cached_toGuest__field1_", Object.class)));
}
@ExplodeLoop
@Override
public Object execute(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.ReadFieldNode.doCached(Node, HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode)] || SpecializationActive[HostObject.ReadFieldNode.doUncached(HostFieldDesc, HostObject)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.ReadFieldNode.doCached(Node, HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg1Value == s0_.cachedField_)) {
return ReadFieldNode.doCached(s0_, arg1Value, arg2Value, s0_.cachedField_, this.cached_toGuest_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.ReadFieldNode.doUncached(HostFieldDesc, HostObject)] */) {
return ReadFieldNode.doUncached(arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.ReadFieldNode.doUncached(HostFieldDesc, HostObject)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg1Value == s0_.cachedField_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg1Value == s0_.cachedField_);
if (count0_ < (ReadFieldNode.LIMIT)) {
s0_ = arg0Value.insert(new CachedData(s0_original));
s0_.cachedField_ = (arg1Value);
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.ReadFieldNode.doCached(Node, HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return ReadFieldNode.doCached(s0_, arg1Value, arg2Value, s0_.cachedField_, this.cached_toGuest_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.ReadFieldNode.doCached(Node, HostFieldDesc, HostObject, HostFieldDesc, ToGuestValueNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.ReadFieldNode.doUncached(HostFieldDesc, HostObject)] */;
this.state_0_.set(arg0Value, state_0);
return ReadFieldNode.doUncached(arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ReadFieldNode.class)
@DenyReplace
private static final class CachedData extends Node {
@Child CachedData next_;
/**
* State Info:
* 0-2: InlinedCache
* Specialization: {@link ReadFieldNode#doCached}
* Parameter: {@link ToGuestValueNode} toGuest
* Inline method: {@link ToGuestValueNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cached_state_0_;
/**
* Source Info:
* Specialization: {@link ReadFieldNode#doCached}
* Parameter: {@link HostFieldDesc} cachedField
*/
@CompilationFinal HostFieldDesc cachedField_;
/**
* Source Info:
* Specialization: {@link ReadFieldNode#doCached}
* Parameter: {@link ToGuestValueNode} toGuest
* Inline method: {@link ToGuestValueNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object cached_toGuest__field1_;
CachedData(CachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(ReadFieldNode.class)
@DenyReplace
private static final class Uncached extends ReadFieldNode {
@TruffleBoundary
@Override
public Object execute(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value) {
return ReadFieldNode.doUncached(arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link WriteFieldNode#doCached}
* Activation probability: 0.65000
* With/without class size: 24/9 bytes
* Specialization {@link WriteFieldNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(WriteFieldNode.class)
@SuppressWarnings("javadoc")
static final class WriteFieldNodeGen {
private static final StateField CACHED_CACHED_STATE_0_UPDATER = StateField.create(CachedData.lookup_(), "cached_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static WriteFieldNode getUncached() {
return WriteFieldNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static WriteFieldNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new WriteFieldNodeGen.Inlined(target);
}
@GeneratedBy(WriteFieldNode.class)
@DenyReplace
private static final class Inlined extends WriteFieldNode {
/**
* State Info:
* 0: SpecializationActive {@link WriteFieldNode#doCached}
* 1: SpecializationActive {@link WriteFieldNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
/**
* Source Info:
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link HostToTypeNode} toHost
* Inline method: {@link HostToTypeNodeGen#inline}
*/
private final HostToTypeNode cached_toHost_;
/**
* Source Info:
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile cached_error_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(WriteFieldNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
this.cached_toHost_ = HostToTypeNodeGen.inline(InlineTarget.create(HostToTypeNode.class, CACHED_CACHED_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(CachedData.lookup_(), "cached_toHost__field1_", Node.class)));
this.cached_error_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED_CACHED_STATE_0_UPDATER.subUpdater(2, 1)));
}
@ExplodeLoop
@Override
public void execute(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value, Object arg3Value) throws UnsupportedTypeException, UnknownIdentifierException {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[HostObject.WriteFieldNode.doCached(Node, HostFieldDesc, HostObject, Object, HostFieldDesc, HostToTypeNode, InlinedBranchProfile)] || SpecializationActive[HostObject.WriteFieldNode.doUncached(HostFieldDesc, HostObject, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.WriteFieldNode.doCached(Node, HostFieldDesc, HostObject, Object, HostFieldDesc, HostToTypeNode, InlinedBranchProfile)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg1Value == s0_.cachedField_)) {
WriteFieldNode.doCached(s0_, arg1Value, arg2Value, arg3Value, s0_.cachedField_, this.cached_toHost_, this.cached_error_);
return;
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.WriteFieldNode.doUncached(HostFieldDesc, HostObject, Object)] */) {
WriteFieldNode.doUncached(arg1Value, arg2Value, arg3Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void executeAndSpecialize(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value, Object arg3Value) throws UnsupportedTypeException, UnknownIdentifierException {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[HostObject.WriteFieldNode.doUncached(HostFieldDesc, HostObject, Object)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg1Value == s0_.cachedField_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (arg1Value == s0_.cachedField_);
if (count0_ < (WriteFieldNode.LIMIT)) {
s0_ = arg0Value.insert(new CachedData(s0_original));
s0_.cachedField_ = (arg1Value);
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.WriteFieldNode.doCached(Node, HostFieldDesc, HostObject, Object, HostFieldDesc, HostToTypeNode, InlinedBranchProfile)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
WriteFieldNode.doCached(s0_, arg1Value, arg2Value, arg3Value, s0_.cachedField_, this.cached_toHost_, this.cached_error_);
return;
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[HostObject.WriteFieldNode.doCached(Node, HostFieldDesc, HostObject, Object, HostFieldDesc, HostToTypeNode, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.WriteFieldNode.doUncached(HostFieldDesc, HostObject, Object)] */;
this.state_0_.set(arg0Value, state_0);
WriteFieldNode.doUncached(arg1Value, arg2Value, arg3Value);
return;
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(WriteFieldNode.class)
@DenyReplace
private static final class CachedData extends Node {
@Child CachedData next_;
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link HostToTypeNode} toHost
* Inline method: {@link HostToTypeNodeGen#inline}
* 2: InlinedCache
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cached_state_0_;
/**
* Source Info:
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link HostFieldDesc} cachedField
*/
@CompilationFinal HostFieldDesc cachedField_;
/**
* Source Info:
* Specialization: {@link WriteFieldNode#doCached}
* Parameter: {@link HostToTypeNode} toHost
* Inline method: {@link HostToTypeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node cached_toHost__field1_;
CachedData(CachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(WriteFieldNode.class)
@DenyReplace
private static final class Uncached extends WriteFieldNode {
@TruffleBoundary
@Override
public void execute(Node arg0Value, HostFieldDesc arg1Value, HostObject arg2Value, Object arg3Value) throws UnsupportedTypeException, UnknownIdentifierException {
WriteFieldNode.doUncached(arg1Value, arg2Value, arg3Value);
return;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ContainsKeyNode#doMap}
* Activation probability: 0.65000
* With/without class size: 19/5 bytes
* Specialization {@link ContainsKeyNode#doNotMap}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(ContainsKeyNode.class)
@SuppressWarnings("javadoc")
static final class ContainsKeyNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static ContainsKeyNode getUncached() {
return ContainsKeyNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#map_toHost__field1_}
*
*/
@NeverDefault
public static ContainsKeyNode inline(@RequiredField(bits = 5, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new ContainsKeyNodeGen.Inlined(target);
}
@GeneratedBy(ContainsKeyNode.class)
@DenyReplace
private static final class Inlined extends ContainsKeyNode {
/**
* State Info:
* 0: SpecializationActive {@link ContainsKeyNode#doMap}
* 1: SpecializationActive {@link ContainsKeyNode#doNotMap}
* 2-3: InlinedCache
* Specialization: {@link ContainsKeyNode#doMap}
* Parameter: {@link HostToTypeNode} toHost
* Inline method: {@link HostToTypeNodeGen#inline}
* 4: InlinedCache
* Specialization: {@link ContainsKeyNode#doMap}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
private final StateField state_0_;
private final ReferenceField map_toHost__field1_;
/**
* Source Info:
* Specialization: {@link ContainsKeyNode#doMap}
* Parameter: {@link HostToTypeNode} toHost
* Inline method: {@link HostToTypeNodeGen#inline}
*/
private final HostToTypeNode map_toHost_;
/**
* Source Info:
* Specialization: {@link ContainsKeyNode#doMap}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile map_error_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ContainsKeyNode.class);
this.state_0_ = target.getState(0, 5);
this.map_toHost__field1_ = target.getReference(1, Node.class);
this.map_toHost_ = HostToTypeNodeGen.inline(InlineTarget.create(HostToTypeNode.class, state_0_.subUpdater(2, 2), map_toHost__field1_));
this.map_error_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, state_0_.subUpdater(4, 1)));
}
@Override
public boolean execute(Node arg0Value, HostObject arg1Value, Object arg2Value, HostClassCache arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b11) != 0 /* is SpecializationActive[HostObject.ContainsKeyNode.doMap(Node, HostObject, Object, HostClassCache, HostToTypeNode, InlinedBranchProfile)] || SpecializationActive[HostObject.ContainsKeyNode.doNotMap(Node, HostObject, Object, HostClassCache)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[HostObject.ContainsKeyNode.doMap(Node, HostObject, Object, HostClassCache, HostToTypeNode, InlinedBranchProfile)] */) {
if ((!(arg1Value.isNull())) && (arg1Value.isMap(arg3Value))) {
assert InlineSupport.validate(arg0Value, this.state_0_, this.map_toHost__field1_, this.state_0_);
return ContainsKeyNode.doMap(arg0Value, arg1Value, arg2Value, arg3Value, this.map_toHost_, this.map_error_);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[HostObject.ContainsKeyNode.doNotMap(Node, HostObject, Object, HostClassCache)] */) {
if ((!(arg1Value.isNull())) && (!(arg1Value.isMap(arg3Value)))) {
return ContainsKeyNode.doNotMap(arg0Value, arg1Value, arg2Value, arg3Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private boolean executeAndSpecialize(Node arg0Value, HostObject arg1Value, Object arg2Value, HostClassCache arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((!(arg1Value.isNull())) && (arg1Value.isMap(arg3Value))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[HostObject.ContainsKeyNode.doMap(Node, HostObject, Object, HostClassCache, HostToTypeNode, InlinedBranchProfile)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.map_toHost__field1_, this.state_0_);
return ContainsKeyNode.doMap(arg0Value, arg1Value, arg2Value, arg3Value, this.map_toHost_, this.map_error_);
}
if ((!(arg1Value.isNull())) && (!(arg1Value.isMap(arg3Value)))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[HostObject.ContainsKeyNode.doNotMap(Node, HostObject, Object, HostClassCache)] */;
this.state_0_.set(arg0Value, state_0);
return ContainsKeyNode.doNotMap(arg0Value, arg1Value, arg2Value, arg3Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ContainsKeyNode.class)
@DenyReplace
private static final class Uncached extends ContainsKeyNode {
@TruffleBoundary
@Override
public boolean execute(Node arg0Value, HostObject arg1Value, Object arg2Value, HostClassCache arg3Value) {
if ((!(arg1Value.isNull())) && (arg1Value.isMap(arg3Value))) {
return ContainsKeyNode.doMap(arg0Value, arg1Value, arg2Value, arg3Value, (HostToTypeNodeGen.getUncached()), (InlinedBranchProfile.getUncached()));
}
if ((!(arg1Value.isNull())) && (!(arg1Value.isMap(arg3Value)))) {
return ContainsKeyNode.doNotMap(arg0Value, arg1Value, arg2Value, arg3Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy