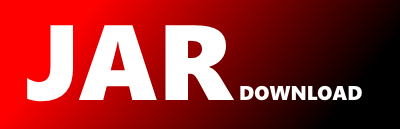
org.pkl.thirdparty.truffle.polyglot.OtherContextGuestObjectGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.polyglot;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.library.DynamicDispatchLibrary;
import org.pkl.thirdparty.truffle.api.library.LibraryExport;
import org.pkl.thirdparty.truffle.api.library.LibraryFactory;
import org.pkl.thirdparty.truffle.api.library.Message;
import org.pkl.thirdparty.truffle.api.library.ReflectionLibrary;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.profiles.InlinedBranchProfile;
import org.pkl.thirdparty.truffle.polyglot.OtherContextGuestObject.Send;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
@GeneratedBy(OtherContextGuestObject.class)
@SuppressWarnings("javadoc")
final class OtherContextGuestObjectGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
private static final LibraryFactory REFLECTION_LIBRARY_ = LibraryFactory.resolve(ReflectionLibrary.class);
static {
LibraryExport.register(OtherContextGuestObject.class, new ReflectionLibraryExports());
}
private OtherContextGuestObjectGen() {
}
@GeneratedBy(OtherContextGuestObject.class)
private static final class ReflectionLibraryExports extends LibraryExport {
private ReflectionLibraryExports() {
super(ReflectionLibrary.class, OtherContextGuestObject.class, false, false, 0);
}
@Override
protected ReflectionLibrary createUncached(Object receiver) {
assert receiver instanceof OtherContextGuestObject;
ReflectionLibrary uncached = new Uncached();
return uncached;
}
@Override
protected ReflectionLibrary createCached(Object receiver) {
assert receiver instanceof OtherContextGuestObject;
return new Cached();
}
@GeneratedBy(OtherContextGuestObject.class)
private static final class Cached extends ReflectionLibrary {
private static final StateField CACHED_CACHED_STATE_0_UPDATER = StateField.create(CachedData.lookup_(), "cached_state_0_");
static final ReferenceField CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cached_cache", CachedData.class);
/**
* Source Info:
* Specialization: {@link Send#doCached}
* Parameter: {@link InlinedBranchProfile} seenOther
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED_SEEN_OTHER_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED_CACHED_STATE_0_UPDATER.subUpdater(0, 1)));
/**
* Source Info:
* Specialization: {@link Send#doCached}
* Parameter: {@link InlinedBranchProfile} seenError
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED_SEEN_ERROR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED_CACHED_STATE_0_UPDATER.subUpdater(1, 1)));
/**
* State Info:
* 0: SpecializationActive {@link Send#doCached}
* 1: SpecializationActive {@link Send#doSlowPath}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private CachedData cached_cache;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof OtherContextGuestObject) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof OtherContextGuestObject;
}
/**
* Debug Info:
* Specialization {@link Send#doCached}
* Activation probability: 0.65000
* With/without class size: 22/9 bytes
* Specialization {@link Send#doSlowPath}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@Override
public Object send(Object arg0Value_, Message arg1Value, Object... arg2Value) throws Exception {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
OtherContextGuestObject arg0Value = ((OtherContextGuestObject) arg0Value_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[OtherContextGuestObject.Send.doCached(OtherContextGuestObject, Message, Object[], Node, ReflectionLibrary, PolyglotSharingLayer, ReflectionLibrary, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[OtherContextGuestObject.Send.doSlowPath(OtherContextGuestObject, Message, Object[], Node)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[OtherContextGuestObject.Send.doCached(OtherContextGuestObject, Message, Object[], Node, ReflectionLibrary, PolyglotSharingLayer, ReflectionLibrary, InlinedBranchProfile, InlinedBranchProfile)] */) {
CachedData s0_ = this.cached_cache;
if (s0_ != null) {
{
ReflectionLibrary receiverLibrary__ = (this);
if ((OtherContextGuestObject.canCache(s0_.cachedLayer_, arg0Value.receiverContext, arg0Value.delegateContext))) {
Node node__ = (s0_);
return Send.doCached(arg0Value, arg1Value, arg2Value, node__, receiverLibrary__, s0_.cachedLayer_, s0_.delegateLibrary_, INLINED_CACHED_SEEN_OTHER_, INLINED_CACHED_SEEN_ERROR_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[OtherContextGuestObject.Send.doSlowPath(OtherContextGuestObject, Message, Object[], Node)] */) {
{
Node node__1 = (this);
return Send.doSlowPath(arg0Value, arg1Value, arg2Value, node__1);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(OtherContextGuestObject arg0Value, Message arg1Value, Object[] arg2Value) throws Exception {
int state_0 = this.state_0_;
{
ReflectionLibrary receiverLibrary__ = null;
Node node__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[OtherContextGuestObject.Send.doSlowPath(OtherContextGuestObject, Message, Object[], Node)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = CACHED_CACHE_UPDATER.getVolatile(this);
CachedData s0_original = s0_;
while (s0_ != null) {
{
receiverLibrary__ = (this);
if ((OtherContextGuestObject.canCache(s0_.cachedLayer_, arg0Value.receiverContext, arg0Value.delegateContext))) {
node__ = (s0_);
break;
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
receiverLibrary__ = (this);
PolyglotSharingLayer cachedLayer__ = (OtherContextGuestObject.getCachedLayer(receiverLibrary__));
if ((OtherContextGuestObject.canCache(cachedLayer__, arg0Value.receiverContext, arg0Value.delegateContext))) {
s0_ = this.insert(new CachedData());
node__ = (s0_);
s0_.cachedLayer_ = cachedLayer__;
ReflectionLibrary delegateLibrary__ = s0_.insert((REFLECTION_LIBRARY_.createDispatched(OtherContextGuestObject.CACHE_LIMIT)));
Objects.requireNonNull(delegateLibrary__, "Specialization 'doCached(OtherContextGuestObject, Message, Object[], Node, ReflectionLibrary, PolyglotSharingLayer, ReflectionLibrary, InlinedBranchProfile, InlinedBranchProfile)' cache 'delegateLibrary' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.delegateLibrary_ = delegateLibrary__;
if (!CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[OtherContextGuestObject.Send.doCached(OtherContextGuestObject, Message, Object[], Node, ReflectionLibrary, PolyglotSharingLayer, ReflectionLibrary, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return Send.doCached(arg0Value, arg1Value, arg2Value, node__, receiverLibrary__, s0_.cachedLayer_, s0_.delegateLibrary_, INLINED_CACHED_SEEN_OTHER_, INLINED_CACHED_SEEN_ERROR_);
}
break;
}
}
}
{
Node node__1 = null;
node__1 = (this);
this.cached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[OtherContextGuestObject.Send.doCached(OtherContextGuestObject, Message, Object[], Node, ReflectionLibrary, PolyglotSharingLayer, ReflectionLibrary, InlinedBranchProfile, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[OtherContextGuestObject.Send.doSlowPath(OtherContextGuestObject, Message, Object[], Node)] */;
this.state_0_ = state_0;
return Send.doSlowPath(arg0Value, arg1Value, arg2Value, node__1);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@GeneratedBy(OtherContextGuestObject.class)
@DenyReplace
private static final class CachedData extends Node {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link Send#doCached}
* Parameter: {@link InlinedBranchProfile} seenOther
* Inline method: {@link InlinedBranchProfile#inline}
* 1: InlinedCache
* Specialization: {@link Send#doCached}
* Parameter: {@link InlinedBranchProfile} seenError
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cached_state_0_;
/**
* Source Info:
* Specialization: {@link Send#doCached}
* Parameter: {@link PolyglotSharingLayer} cachedLayer
*/
@CompilationFinal PolyglotSharingLayer cachedLayer_;
/**
* Source Info:
* Specialization: {@link Send#doCached}
* Parameter: {@link ReflectionLibrary} delegateLibrary
*/
@Child ReflectionLibrary delegateLibrary_;
CachedData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
@GeneratedBy(OtherContextGuestObject.class)
@DenyReplace
private static final class Uncached extends ReflectionLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof OtherContextGuestObject) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof OtherContextGuestObject;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public Object send(Object arg0Value_, Message arg1Value, Object... arg2Value) throws Exception {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
OtherContextGuestObject arg0Value = ((OtherContextGuestObject) arg0Value_);
return Send.doSlowPath(arg0Value, arg1Value, arg2Value, (this));
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy