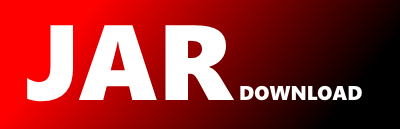
org.pkl.thirdparty.truffle.polyglot.PolyglotLanguageContextFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-config-java-all Show documentation
Show all versions of pkl-config-java-all Show documentation
Shaded fat Jar for pkl-config-java, a Java config library based on the Pkl config language.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.polyglot;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport;
import org.pkl.thirdparty.truffle.api.dsl.NeverDefault;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.RequiredField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.ExplodeLoop;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.profiles.InlinedBranchProfile;
import org.pkl.thirdparty.truffle.polyglot.PolyglotLanguageContext.ToGuestValueNode;
import org.pkl.thirdparty.truffle.polyglot.PolyglotLanguageContext.ToGuestValuesNode;
import org.pkl.thirdparty.truffle.polyglot.PolyglotLanguageContext.ToHostValueNode;
import java.lang.invoke.MethodHandles;
import java.util.Objects;
import org.pkl.thirdparty.graalvm.polyglot.Value;
@GeneratedBy(PolyglotLanguageContext.class)
@SuppressWarnings({"javadoc", "unused"})
final class PolyglotLanguageContextFactory {
/**
* Debug Info:
* Specialization {@link ToHostValueNode#doCached}
* Activation probability: 0.65000
* With/without class size: 19/8 bytes
* Specialization {@link ToHostValueNode#doGeneric}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(ToHostValueNode.class)
@SuppressWarnings("javadoc")
static final class ToHostValueNodeGen {
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static ToHostValueNode inline(@RequiredField(bits = 2, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new ToHostValueNodeGen.Inlined(target);
}
@GeneratedBy(ToHostValueNode.class)
@DenyReplace
private static final class Inlined extends ToHostValueNode {
/**
* State Info:
* 0: SpecializationActive {@link ToHostValueNode#doCached}
* 1: SpecializationActive {@link ToHostValueNode#doGeneric}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ToHostValueNode.class);
this.state_0_ = target.getState(0, 2);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
Value execute(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doCached(PolyglotLanguageContext, Object, Class<>, PolyglotValueDispatch)] || SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doGeneric(PolyglotLanguageContext, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doCached(PolyglotLanguageContext, Object, Class<>, PolyglotValueDispatch)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
if ((arg2Value.getClass() == s0_.cachedClass_)) {
return doCached(arg1Value, arg2Value, s0_.cachedClass_, s0_.cachedValue_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doGeneric(PolyglotLanguageContext, Object)] */) {
return doGeneric(arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Value executeAndSpecialize(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doGeneric(PolyglotLanguageContext, Object)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg2Value.getClass() == s0_.cachedClass_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
Class> cachedClass__ = (arg2Value.getClass());
if ((arg2Value.getClass() == cachedClass__) && count0_ < (3)) {
s0_ = new CachedData(s0_original);
s0_.cachedClass_ = cachedClass__;
PolyglotValueDispatch cachedValue__ = (ToHostValueNode.lookupDispatch(arg1Value, arg2Value));
Objects.requireNonNull(cachedValue__, "Specialization 'doCached(PolyglotLanguageContext, Object, Class<>, PolyglotValueDispatch)' cache 'cachedValue' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.cachedValue_ = cachedValue__;
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doCached(PolyglotLanguageContext, Object, Class<>, PolyglotValueDispatch)] */;
this.state_0_.set(arg0Value, state_0);
}
}
}
if (s0_ != null) {
return doCached(arg1Value, arg2Value, s0_.cachedClass_, s0_.cachedValue_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doCached(PolyglotLanguageContext, Object, Class<>, PolyglotValueDispatch)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[PolyglotLanguageContext.ToHostValueNode.doGeneric(PolyglotLanguageContext, Object)] */;
this.state_0_.set(arg0Value, state_0);
return doGeneric(arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ToHostValueNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link ToHostValueNode#doCached}
* Parameter: {@link Class} cachedClass
*/
@CompilationFinal Class> cachedClass_;
/**
* Source Info:
* Specialization: {@link ToHostValueNode#doCached}
* Parameter: {@link PolyglotValueDispatch} cachedValue
*/
@CompilationFinal PolyglotValueDispatch cachedValue_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
/**
* Debug Info:
* Specialization {@link ToGuestValueNode#doNull}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link ToGuestValueNode#doCached}
* Activation probability: 0.33333
* With/without class size: 10/4 bytes
* Specialization {@link ToGuestValueNode#doUncached}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(ToGuestValueNode.class)
@SuppressWarnings("javadoc")
static final class ToGuestValueNodeGen extends ToGuestValueNode {
static final ReferenceField CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cached_cache", CachedData.class);
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link ToGuestValueNode#doNull}
* 1: SpecializationActive {@link ToGuestValueNode#doCached}
* 2: SpecializationActive {@link ToGuestValueNode#doUncached}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private CachedData cached_cache;
private ToGuestValueNodeGen() {
}
@ExplodeLoop
@Override
Object execute(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] || SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] || SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] */) {
if ((arg2Value == null)) {
return ToGuestValueNode.doNull(this, arg1Value, arg2Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */ && (arg2Value != null)) {
CachedData s1_ = this.cached_cache;
while (s1_ != null) {
if ((arg2Value.getClass() == s1_.cachedReceiver_)) {
return ToGuestValueNode.doCached(this, arg1Value, arg2Value, s1_.cachedReceiver_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */) {
return ToGuestValueNode.doUncached(this, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if ((arg2Value == null)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] */;
this.state_0_ = state_0;
return ToGuestValueNode.doNull(this, arg1Value, arg2Value);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */ && (arg2Value != null)) {
while (true) {
int count1_ = 0;
CachedData s1_ = CACHED_CACHE_UPDATER.getVolatile(this);
CachedData s1_original = s1_;
while (s1_ != null) {
if ((arg2Value.getClass() == s1_.cachedReceiver_)) {
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
Class> cachedReceiver__ = (arg2Value.getClass());
if ((arg2Value.getClass() == cachedReceiver__) && count1_ < (3)) {
s1_ = new CachedData(s1_original);
s1_.cachedReceiver_ = cachedReceiver__;
if (!CACHED_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return ToGuestValueNode.doCached(this, arg1Value, arg2Value, s1_.cachedReceiver_);
}
break;
}
}
this.cached_cache = null;
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */;
this.state_0_ = state_0;
return ToGuestValueNode.doUncached(this, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
CachedData s1_ = this.cached_cache;
if ((s1_ == null || s1_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static ToGuestValueNode create() {
return new ToGuestValueNodeGen();
}
@NeverDefault
public static ToGuestValueNode getUncached() {
return ToGuestValueNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static ToGuestValueNode inline(@RequiredField(bits = 3, value = StateField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new ToGuestValueNodeGen.Inlined(target);
}
@GeneratedBy(ToGuestValueNode.class)
@DenyReplace
private static final class Inlined extends ToGuestValueNode {
/**
* State Info:
* 0: SpecializationActive {@link ToGuestValueNode#doNull}
* 1: SpecializationActive {@link ToGuestValueNode#doCached}
* 2: SpecializationActive {@link ToGuestValueNode#doUncached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ToGuestValueNode.class);
this.state_0_ = target.getState(0, 3);
this.cached_cache = target.getReference(1, CachedData.class);
}
@ExplodeLoop
@Override
Object execute(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] || SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] || SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] */) {
if ((arg2Value == null)) {
return ToGuestValueNode.doNull(arg0Value, arg1Value, arg2Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */ && (arg2Value != null)) {
CachedData s1_ = this.cached_cache.get(arg0Value);
while (s1_ != null) {
if ((arg2Value.getClass() == s1_.cachedReceiver_)) {
return ToGuestValueNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedReceiver_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */) {
return ToGuestValueNode.doUncached(arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((arg2Value == null)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doNull(Node, PolyglotLanguageContext, Object)] */;
this.state_0_.set(arg0Value, state_0);
return ToGuestValueNode.doNull(arg0Value, arg1Value, arg2Value);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */ && (arg2Value != null)) {
while (true) {
int count1_ = 0;
CachedData s1_ = this.cached_cache.getVolatile(arg0Value);
CachedData s1_original = s1_;
while (s1_ != null) {
if ((arg2Value.getClass() == s1_.cachedReceiver_)) {
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
Class> cachedReceiver__ = (arg2Value.getClass());
if ((arg2Value.getClass() == cachedReceiver__) && count1_ < (3)) {
s1_ = new CachedData(s1_original);
s1_.cachedReceiver_ = cachedReceiver__;
if (!this.cached_cache.compareAndSet(arg0Value, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */;
this.state_0_.set(arg0Value, state_0);
}
}
}
if (s1_ != null) {
return ToGuestValueNode.doCached(arg0Value, arg1Value, arg2Value, s1_.cachedReceiver_);
}
break;
}
}
this.cached_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doCached(Node, PolyglotLanguageContext, Object, Class<>)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[PolyglotLanguageContext.ToGuestValueNode.doUncached(Node, PolyglotLanguageContext, Object)] */;
this.state_0_.set(arg0Value, state_0);
return ToGuestValueNode.doUncached(arg0Value, arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(ToGuestValueNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link ToGuestValueNode#doCached}
* Parameter: {@link Class} cachedReceiver
*/
@CompilationFinal Class> cachedReceiver_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(ToGuestValueNode.class)
@DenyReplace
private static final class Uncached extends ToGuestValueNode {
@TruffleBoundary
@Override
Object execute(Node arg0Value, PolyglotLanguageContext arg1Value, Object arg2Value) {
if ((arg2Value == null)) {
return ToGuestValueNode.doNull(arg0Value, arg1Value, arg2Value);
}
return ToGuestValueNode.doUncached(arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link ToGuestValuesNode#doZero}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link ToGuestValuesNode#doCached}
* Activation probability: 0.33333
* With/without class size: 10/4 bytes
* Specialization {@link ToGuestValuesNode#doGeneric}
* Activation probability: 0.18333
* With/without class size: 8/5 bytes
*
*/
@GeneratedBy(ToGuestValuesNode.class)
@SuppressWarnings("javadoc")
static final class ToGuestValuesNodeGen {
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
- {@link Inlined#generic_toGuest__field1_}
*
*/
@NeverDefault
public static ToGuestValuesNode inline(@RequiredField(bits = 7, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Object.class, value = ReferenceField.class) InlineTarget target) {
return new ToGuestValuesNodeGen.Inlined(target);
}
@GeneratedBy(ToGuestValuesNode.class)
@DenyReplace
private static final class Inlined extends ToGuestValuesNode {
/**
* State Info:
* 0: SpecializationActive {@link ToGuestValuesNode#doZero}
* 1: SpecializationActive {@link ToGuestValuesNode#doCached}
* 2: SpecializationActive {@link ToGuestValuesNode#doGeneric}
* 3: InlinedCache
* Specialization: {@link ToGuestValuesNode#doCached}
* Parameter: {@link InlinedBranchProfile} needsCopyProfile
* Inline method: {@link InlinedBranchProfile#inline}
* 4-6: InlinedCache
* Specialization: {@link ToGuestValuesNode#doGeneric}
* Parameter: {@link ToGuestValueNode} toGuest
* Inline method: {@link ToGuestValueNodeGen#inline}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
private final ReferenceField
© 2015 - 2024 Weber Informatics LLC | Privacy Policy