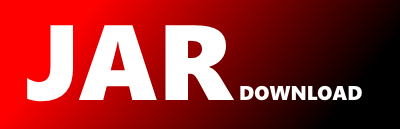
org.pkl.thirdparty.commonmark.internal.BlockQuoteParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-tools Show documentation
Show all versions of pkl-tools Show documentation
Fat Jar containing pkl-cli, pkl-codegen-java, pkl-codegen-kotlin, pkl-config-java, pkl-core, pkl-doc, and their shaded third-party dependencies.
package org.pkl.thirdparty.commonmark.internal;
import org.pkl.thirdparty.commonmark.internal.util.Parsing;
import org.pkl.thirdparty.commonmark.node.Block;
import org.pkl.thirdparty.commonmark.node.BlockQuote;
import org.pkl.thirdparty.commonmark.parser.block.*;
import org.pkl.thirdparty.commonmark.text.Characters;
public class BlockQuoteParser extends AbstractBlockParser {
private final BlockQuote block = new BlockQuote();
@Override
public boolean isContainer() {
return true;
}
@Override
public boolean canContain(Block block) {
return true;
}
@Override
public BlockQuote getBlock() {
return block;
}
@Override
public BlockContinue tryContinue(ParserState state) {
int nextNonSpace = state.getNextNonSpaceIndex();
if (isMarker(state, nextNonSpace)) {
int newColumn = state.getColumn() + state.getIndent() + 1;
// optional following space or tab
if (Characters.isSpaceOrTab(state.getLine().getContent(), nextNonSpace + 1)) {
newColumn++;
}
return BlockContinue.atColumn(newColumn);
} else {
return BlockContinue.none();
}
}
private static boolean isMarker(ParserState state, int index) {
CharSequence line = state.getLine().getContent();
return state.getIndent() < Parsing.CODE_BLOCK_INDENT && index < line.length() && line.charAt(index) == '>';
}
public static class Factory extends AbstractBlockParserFactory {
public BlockStart tryStart(ParserState state, MatchedBlockParser matchedBlockParser) {
int nextNonSpace = state.getNextNonSpaceIndex();
if (isMarker(state, nextNonSpace)) {
int newColumn = state.getColumn() + state.getIndent() + 1;
// optional following space or tab
if (Characters.isSpaceOrTab(state.getLine().getContent(), nextNonSpace + 1)) {
newColumn++;
}
return BlockStart.of(new BlockQuoteParser()).atColumn(newColumn);
} else {
return BlockStart.none();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy