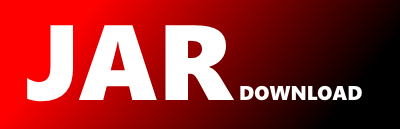
org.pkl.thirdparty.commonmark.node.DefinitionMap Maven / Gradle / Ivy
Show all versions of pkl-tools Show documentation
package org.pkl.thirdparty.commonmark.node;
import org.pkl.thirdparty.commonmark.internal.util.Escaping;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
/**
* A map that can be used to store and look up reference definitions by a label. The labels are case-insensitive and
* normalized, the same way as for {@link LinkReferenceDefinition} nodes.
*
* @param the type of value
*/
public class DefinitionMap {
private final Class type;
// LinkedHashMap for determinism and to preserve document order
private final Map definitions = new LinkedHashMap<>();
public DefinitionMap(Class type) {
this.type = type;
}
public Class getType() {
return type;
}
public void addAll(DefinitionMap that) {
for (var entry : that.definitions.entrySet()) {
// Note that keys are already normalized, so we can add them directly
definitions.putIfAbsent(entry.getKey(), entry.getValue());
}
}
/**
* Store a new definition unless one is already in the map. If there is no definition for that label yet, return null.
* Otherwise, return the existing definition.
*
* The label is normalized by the definition map before storing.
*/
public D putIfAbsent(String label, D definition) {
String normalizedLabel = Escaping.normalizeLabelContent(label);
// spec: When there are multiple matching link reference definitions, the first is used
return definitions.putIfAbsent(normalizedLabel, definition);
}
/**
* Look up a definition by label. The label is normalized by the definition map before lookup.
*
* @return the value or null
*/
public D get(String label) {
String normalizedLabel = Escaping.normalizeLabelContent(label);
return definitions.get(normalizedLabel);
}
public Set keySet() {
return definitions.keySet();
}
public Collection values() {
return definitions.values();
}
}