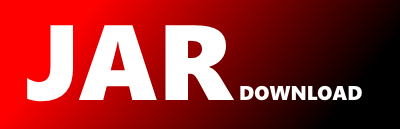
org.pkl.thirdparty.commonmark.parser.InlineParserContext Maven / Gradle / Ivy
Show all versions of pkl-tools Show documentation
package org.pkl.thirdparty.commonmark.parser;
import org.pkl.thirdparty.commonmark.node.LinkReferenceDefinition;
import org.pkl.thirdparty.commonmark.parser.beta.LinkProcessor;
import org.pkl.thirdparty.commonmark.parser.beta.InlineContentParserFactory;
import org.pkl.thirdparty.commonmark.parser.delimiter.DelimiterProcessor;
import java.util.List;
import java.util.Set;
/**
* Context for inline parsing.
*/
public interface InlineParserContext {
/**
* @return custom inline content parsers that have been configured with
* {@link Parser.Builder#customInlineContentParserFactory(InlineContentParserFactory)}
*/
List getCustomInlineContentParserFactories();
/**
* @return custom delimiter processors that have been configured with
* {@link Parser.Builder#customDelimiterProcessor(DelimiterProcessor)}
*/
List getCustomDelimiterProcessors();
/**
* @return custom link processors that have been configured with {@link Parser.Builder#linkProcessor}.
*/
List getCustomLinkProcessors();
/**
* @return custom link markers that have been configured with {@link Parser.Builder#linkMarker}.
*/
Set getCustomLinkMarkers();
/**
* Look up a {@link LinkReferenceDefinition} for a given label.
*
* Note that the passed in label does not need to be normalized; implementations are responsible for doing the
* normalization before lookup.
*
* @param label the link label to look up
* @return the definition if one exists, {@code null} otherwise
* @deprecated use {@link #getDefinition} with {@link LinkReferenceDefinition} instead
*/
@Deprecated
LinkReferenceDefinition getLinkReferenceDefinition(String label);
/**
* Look up a definition of a type for a given label.
*
* Note that the passed in label does not need to be normalized; implementations are responsible for doing the
* normalization before lookup.
*
* @return the definition if one exists, null otherwise
*/
D getDefinition(Class type, String label);
}