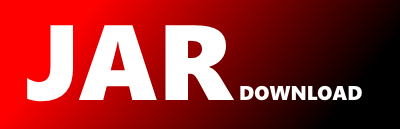
org.pkl.thirdparty.truffle.api.debug.SetThreadSuspensionEnabledNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-tools Show documentation
Show all versions of pkl-tools Show documentation
Fat Jar containing pkl-cli, pkl-codegen-java, pkl-codegen-kotlin, pkl-config-java, pkl-core, pkl-doc, and their shaded third-party dependencies.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.api.debug;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.debug.Breakpoint.SessionList;
import org.pkl.thirdparty.truffle.api.debug.DebuggerSession.ThreadSuspension;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.NeverDefault;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.ExplodeLoop;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
/**
* Debug Info:
* Specialization {@link SetThreadSuspensionEnabledNode#doCached}
* Activation probability: 0.65000
* With/without class size: 22/12 bytes
* Specialization {@link SetThreadSuspensionEnabledNode#doGeneric}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(SetThreadSuspensionEnabledNode.class)
@SuppressWarnings("javadoc")
final class SetThreadSuspensionEnabledNodeGen extends SetThreadSuspensionEnabledNode {
static final ReferenceField CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cached_cache", CachedData.class);
/**
* State Info:
* 0: SpecializationActive {@link SetThreadSuspensionEnabledNode#doCached}
* 1: SpecializationActive {@link SetThreadSuspensionEnabledNode#doGeneric}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private CachedData cached_cache;
private SetThreadSuspensionEnabledNodeGen() {
}
@ExplodeLoop
@Override
protected void execute(boolean arg0Value, SessionList arg1Value, long arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[SetThreadSuspensionEnabledNode.doCached(boolean, SessionList, long, long, ThreadSuspension)] || SpecializationActive[SetThreadSuspensionEnabledNode.doGeneric(boolean, SessionList, long)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SetThreadSuspensionEnabledNode.doCached(boolean, SessionList, long, long, ThreadSuspension)] */ && (arg1Value.next == null)) {
CachedData s0_ = this.cached_cache;
while (s0_ != null) {
if ((arg2Value == s0_.currentThreadId_)) {
doCached(arg0Value, arg1Value, arg2Value, s0_.currentThreadId_, s0_.threadSuspension_);
return;
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SetThreadSuspensionEnabledNode.doGeneric(boolean, SessionList, long)] */) {
doGeneric(arg0Value, arg1Value, arg2Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void executeAndSpecialize(boolean arg0Value, SessionList arg1Value, long arg2Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[SetThreadSuspensionEnabledNode.doGeneric(boolean, SessionList, long)] */ && (arg1Value.next == null)) {
while (true) {
int count0_ = 0;
CachedData s0_ = CACHED_CACHE_UPDATER.getVolatile(this);
CachedData s0_original = s0_;
while (s0_ != null) {
if ((arg2Value == s0_.currentThreadId_)) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
long currentThreadId__ = (SetThreadSuspensionEnabledNode.currentThreadId());
if ((arg2Value == currentThreadId__) && count0_ < (SetThreadSuspensionEnabledNode.CACHE_LIMIT)) {
s0_ = new CachedData(s0_original);
s0_.currentThreadId_ = currentThreadId__;
s0_.threadSuspension_ = (getThreadSuspension(arg1Value));
if (!CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[SetThreadSuspensionEnabledNode.doCached(boolean, SessionList, long, long, ThreadSuspension)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
doCached(arg0Value, arg1Value, arg2Value, s0_.currentThreadId_, s0_.threadSuspension_);
return;
}
break;
}
}
this.cached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[SetThreadSuspensionEnabledNode.doCached(boolean, SessionList, long, long, ThreadSuspension)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[SetThreadSuspensionEnabledNode.doGeneric(boolean, SessionList, long)] */;
this.state_0_ = state_0;
doGeneric(arg0Value, arg1Value, arg2Value);
return;
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
CachedData s0_ = this.cached_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static SetThreadSuspensionEnabledNode create() {
return new SetThreadSuspensionEnabledNodeGen();
}
@GeneratedBy(SetThreadSuspensionEnabledNode.class)
@DenyReplace
private static final class CachedData {
@CompilationFinal final CachedData next_;
/**
* Source Info:
* Specialization: {@link SetThreadSuspensionEnabledNode#doCached}
* Parameter: long currentThreadId
*/
@CompilationFinal long currentThreadId_;
/**
* Source Info:
* Specialization: {@link SetThreadSuspensionEnabledNode#doCached}
* Parameter: {@link ThreadSuspension} threadSuspension
*/
@CompilationFinal ThreadSuspension threadSuspension_;
CachedData(CachedData next_) {
this.next_ = next_;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy