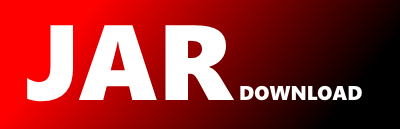
org.pkl.thirdparty.truffle.api.library.ReflectionLibraryDefaultGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-tools Show documentation
Show all versions of pkl-tools Show documentation
Fat Jar containing pkl-cli, pkl-codegen-java, pkl-codegen-kotlin, pkl-config-java, pkl-core, pkl-doc, and their shaded third-party dependencies.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.api.library;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.library.ReflectionLibraryDefault.Send;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.ExplodeLoop;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import java.lang.invoke.MethodHandles;
@GeneratedBy(ReflectionLibraryDefault.class)
@SuppressWarnings("javadoc")
final class ReflectionLibraryDefaultGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(ReflectionLibraryDefault.class, new ReflectionLibraryExports());
}
private ReflectionLibraryDefaultGen() {
}
@GeneratedBy(ReflectionLibraryDefault.class)
private static final class ReflectionLibraryExports extends LibraryExport {
private ReflectionLibraryExports() {
super(ReflectionLibrary.class, Object.class, true, false, 0);
}
@Override
protected ReflectionLibrary createUncached(Object receiver) {
ReflectionLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected ReflectionLibrary createCached(Object receiver) {
return new Cached(receiver);
}
@GeneratedBy(ReflectionLibraryDefault.class)
private static final class Cached extends ReflectionLibrary {
static final ReferenceField SEND_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "sendCached_cache", SendCachedData.class);
@Child private DynamicDispatchLibrary dynamicDispatch_;
private final Class> dynamicDispatchTarget_;
/**
* State Info:
* 0: SpecializationActive {@link Send#doSendCached}
* 1: SpecializationActive {@link Send#doSendGeneric}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private SendCachedData sendCached_cache;
protected Cached(Object receiver) {
this.dynamicDispatch_ = insert(DYNAMIC_DISPATCH_LIBRARY_.create(receiver));
this.dynamicDispatchTarget_ = DYNAMIC_DISPATCH_LIBRARY_.getUncached(receiver).dispatch(receiver);
}
@Override
public boolean accepts(Object receiver) {
return dynamicDispatch_.accepts(receiver) && dynamicDispatch_.dispatch(receiver) == dynamicDispatchTarget_;
}
/**
* Debug Info:
* Specialization {@link Send#doSendCached}
* Activation probability: 0.65000
* With/without class size: 22/8 bytes
* Specialization {@link Send#doSendGeneric}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@ExplodeLoop
@Override
public Object send(Object arg0Value, Message arg1Value, Object... arg2Value) throws Exception {
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[ReflectionLibraryDefault.Send.doSendCached(Object, Message, Object[], Message, Library)] || SpecializationActive[ReflectionLibraryDefault.Send.doSendGeneric(Object, Message, Object[])] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectionLibraryDefault.Send.doSendCached(Object, Message, Object[], Message, Library)] */) {
SendCachedData s0_ = this.sendCached_cache;
while (s0_ != null) {
if ((arg1Value == s0_.cachedMessage_) && (s0_.cachedLibrary_.accepts(arg0Value))) {
return Send.doSendCached(arg0Value, arg1Value, arg2Value, s0_.cachedMessage_, s0_.cachedLibrary_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectionLibraryDefault.Send.doSendGeneric(Object, Message, Object[])] */) {
return Send.doSendGeneric(arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object executeAndSpecialize(Object arg0Value, Message arg1Value, Object[] arg2Value) throws Exception {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ReflectionLibraryDefault.Send.doSendGeneric(Object, Message, Object[])] */) {
while (true) {
int count0_ = 0;
SendCachedData s0_ = SEND_CACHED_CACHE_UPDATER.getVolatile(this);
SendCachedData s0_original = s0_;
while (s0_ != null) {
if ((arg1Value == s0_.cachedMessage_) && (s0_.cachedLibrary_.accepts(arg0Value))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
Library cachedLibrary__ = this.insert((Send.createLibrary(arg1Value, arg0Value)));
// assert (arg1Value == s0_.cachedMessage_);
if ((cachedLibrary__.accepts(arg0Value)) && count0_ < (ReflectionLibraryDefault.LIMIT)) {
s0_ = this.insert(new SendCachedData(s0_original));
s0_.cachedMessage_ = (arg1Value);
s0_.cachedLibrary_ = s0_.insert(cachedLibrary__);
if (!SEND_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectionLibraryDefault.Send.doSendCached(Object, Message, Object[], Message, Library)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return Send.doSendCached(arg0Value, arg1Value, arg2Value, s0_.cachedMessage_, s0_.cachedLibrary_);
}
break;
}
}
this.sendCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[ReflectionLibraryDefault.Send.doSendCached(Object, Message, Object[], Message, Library)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectionLibraryDefault.Send.doSendGeneric(Object, Message, Object[])] */;
this.state_0_ = state_0;
return Send.doSendGeneric(arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
SendCachedData s0_ = this.sendCached_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@GeneratedBy(ReflectionLibraryDefault.class)
@DenyReplace
private static final class SendCachedData extends Node {
@Child SendCachedData next_;
/**
* Source Info:
* Specialization: {@link Send#doSendCached}
* Parameter: {@link Message} cachedMessage
*/
@CompilationFinal Message cachedMessage_;
/**
* Source Info:
* Specialization: {@link Send#doSendCached}
* Parameter: {@link Library} cachedLibrary
*/
@Child Library cachedLibrary_;
SendCachedData(SendCachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
}
@GeneratedBy(ReflectionLibraryDefault.class)
@DenyReplace
private static final class Uncached extends ReflectionLibrary {
@Child private DynamicDispatchLibrary dynamicDispatch_;
private final Class> dynamicDispatchTarget_;
protected Uncached(Object receiver) {
this.dynamicDispatch_ = DYNAMIC_DISPATCH_LIBRARY_.getUncached(receiver);
this.dynamicDispatchTarget_ = dynamicDispatch_.dispatch(receiver);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return dynamicDispatch_.accepts(receiver) && dynamicDispatch_.dispatch(receiver) == dynamicDispatchTarget_;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public Object send(Object arg0Value, Message arg1Value, Object... arg2Value) throws Exception {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return Send.doSendGeneric(arg0Value, arg1Value, arg2Value);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy