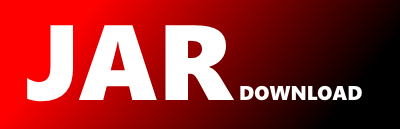
org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImplGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-tools Show documentation
Show all versions of pkl-tools Show documentation
Fat Jar containing pkl-cli, pkl-codegen-java, pkl-codegen-kotlin, pkl-config-java, pkl-core, pkl-doc, and their shaded third-party dependencies.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.object;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.library.DefaultExportProvider;
import org.pkl.thirdparty.truffle.api.library.LibraryExport;
import org.pkl.thirdparty.truffle.api.library.LibraryFactory;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.nodes.UnexpectedResultException;
import org.pkl.thirdparty.truffle.api.object.DynamicObject;
import org.pkl.thirdparty.truffle.api.object.DynamicObjectLibrary;
import org.pkl.thirdparty.truffle.api.object.Property;
import org.pkl.thirdparty.truffle.api.object.Shape;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImpl.KeyCacheNode;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImpl.MakeSharedNode;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImpl.ResetShapeNode;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImpl.SetDynamicTypeNode;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImpl.SetFlagsNode;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImplFactory.MakeSharedNodeGen;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImplFactory.ResetShapeNodeGen;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImplFactory.SetDynamicTypeNodeGen;
import org.pkl.thirdparty.truffle.object.DynamicObjectLibraryImplFactory.SetFlagsNodeGen;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
@GeneratedBy(DynamicObjectLibraryImpl.class)
@SuppressWarnings({"javadoc", "unused"})
public final class DynamicObjectLibraryImplGen {
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
static {
LibraryExport.register(DynamicObjectLibraryImpl.class, new DynamicObjectLibraryExports());
}
private DynamicObjectLibraryImplGen() {
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
public static final class DynamicObjectLibraryProvider implements DefaultExportProvider {
@Override
public String getLibraryClassName() {
return "org.pkl.thirdparty.truffle.api.object.DynamicObjectLibrary";
}
@Override
public Class> getDefaultExport() {
return DynamicObjectLibraryImpl.class;
}
@Override
public Class> getReceiverClass() {
return DynamicObject.class;
}
@Override
public int getPriority() {
return 10;
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
private static final class DynamicObjectLibraryExports extends LibraryExport {
private DynamicObjectLibraryExports() {
super(DynamicObjectLibrary.class, DynamicObject.class, false, false, 0);
}
@Override
protected DynamicObjectLibrary createUncached(Object receiver) {
assert receiver instanceof DynamicObject;
DynamicObjectLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected DynamicObjectLibrary createCached(Object receiver) {
assert receiver instanceof DynamicObject;
return new Cached(receiver);
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
private static final class Cached extends DynamicObjectLibrary {
private static final StateField SET_DYNAMIC_TYPE_SET_DYNAMIC_TYPE_STATE_0_UPDATER = StateField.create(SetDynamicTypeNode_SetDynamicTypeData.lookup_(), "setDynamicType_state_0_");
private static final StateField SET_SHAPE_FLAGS_SET_SHAPE_FLAGS_STATE_0_UPDATER = StateField.create(SetShapeFlagsNode_SetShapeFlagsData.lookup_(), "setShapeFlags_state_0_");
private static final StateField MARK_SHARED_MARK_SHARED_STATE_0_UPDATER = StateField.create(MarkSharedNode_MarkSharedData.lookup_(), "markShared_state_0_");
private static final StateField RESET_SHAPE_RESET_SHAPE_STATE_0_UPDATER = StateField.create(ResetShapeNode_ResetShapeData.lookup_(), "resetShape_state_0_");
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#setDynamicType}
* Parameter: {@link SetDynamicTypeNode} setCache
* Inline method: {@link SetDynamicTypeNodeGen#inline}
*/
private static final SetDynamicTypeNode INLINED_SET_DYNAMIC_TYPE_NODE__SET_DYNAMIC_TYPE_SET_CACHE_ = SetDynamicTypeNodeGen.inline(InlineTarget.create(SetDynamicTypeNode.class, SET_DYNAMIC_TYPE_SET_DYNAMIC_TYPE_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(SetDynamicTypeNode_SetDynamicTypeData.lookup_(), "setDynamicTypeNode__setDynamicType_setCache__field1_", Object.class)));
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#setShapeFlags}
* Parameter: {@link SetFlagsNode} setCache
* Inline method: {@link SetFlagsNodeGen#inline}
*/
private static final SetFlagsNode INLINED_SET_SHAPE_FLAGS_NODE__SET_SHAPE_FLAGS_SET_CACHE_ = SetFlagsNodeGen.inline(InlineTarget.create(SetFlagsNode.class, SET_SHAPE_FLAGS_SET_SHAPE_FLAGS_STATE_0_UPDATER.subUpdater(0, 2), ReferenceField.create(SetShapeFlagsNode_SetShapeFlagsData.lookup_(), "setShapeFlagsNode__setShapeFlags_setCache__field1_", Object.class)));
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#markShared}
* Parameter: {@link MakeSharedNode} setCache
* Inline method: {@link MakeSharedNodeGen#inline}
*/
private static final MakeSharedNode INLINED_MARK_SHARED_NODE__MARK_SHARED_SET_CACHE_ = MakeSharedNodeGen.inline(InlineTarget.create(MakeSharedNode.class, MARK_SHARED_MARK_SHARED_STATE_0_UPDATER.subUpdater(0, 1), ReferenceField.create(MarkSharedNode_MarkSharedData.lookup_(), "markSharedNode__markShared_setCache__field1_", Object.class)));
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#resetShape}
* Parameter: {@link ResetShapeNode} setCache
* Inline method: {@link ResetShapeNodeGen#inline}
*/
private static final ResetShapeNode INLINED_RESET_SHAPE_NODE__RESET_SHAPE_SET_CACHE_ = ResetShapeNodeGen.inline(InlineTarget.create(ResetShapeNode.class, RESET_SHAPE_RESET_SHAPE_STATE_0_UPDATER.subUpdater(0, 1), ReferenceField.create(ResetShapeNode_ResetShapeData.lookup_(), "resetShapeNode__resetShape_setCache__field1_", Object.class)));
private final Class extends DynamicObject> receiverClass_;
@Child private DynamicObjectLibrary fallback_;
/**
* State Info:
* 0: SpecializationActive {@link DynamicObjectLibraryImpl#getOrDefault}
* 1: SpecializationActive {@link DynamicObjectLibraryImpl#getIntOrDefault}
* 2: SpecializationActive {@link DynamicObjectLibraryImpl#getDoubleOrDefault}
* 3: SpecializationActive {@link DynamicObjectLibraryImpl#getLongOrDefault}
* 4: SpecializationActive {@link DynamicObjectLibraryImpl#containsKey}
* 5: SpecializationActive {@link DynamicObjectLibraryImpl#put}
* 6: SpecializationActive {@link DynamicObjectLibraryImpl#putInt}
* 7: SpecializationActive {@link DynamicObjectLibraryImpl#putLong}
* 8: SpecializationActive {@link DynamicObjectLibraryImpl#putDouble}
* 9: SpecializationActive {@link DynamicObjectLibraryImpl#putIfPresent}
* 10: SpecializationActive {@link DynamicObjectLibraryImpl#putWithFlags}
* 11: SpecializationActive {@link DynamicObjectLibraryImpl#putConstant}
* 12: SpecializationActive {@link DynamicObjectLibraryImpl#getProperty}
* 13: SpecializationActive {@link DynamicObjectLibraryImpl#setPropertyFlags}
* 14: SpecializationActive {@link DynamicObjectLibraryImpl#removeKey}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#accepts}
* Parameter: {@link Shape} cachedShape
*/
@CompilationFinal private Shape cachedShape;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#getOrDefault}
* Parameter: {@link KeyCacheNode} keyCache
*/
@Child private KeyCacheNode keyCache;
@Child private SetDynamicTypeNode_SetDynamicTypeData setDynamicTypeNode__setDynamicType_cache;
@Child private SetShapeFlagsNode_SetShapeFlagsData setShapeFlagsNode__setShapeFlags_cache;
@Child private MarkSharedNode_MarkSharedData markSharedNode__markShared_cache;
@Child private ResetShapeNode_ResetShapeData resetShapeNode__resetShape_cache;
protected Cached(Object receiver) {
DynamicObject castReceiver = ((DynamicObject) receiver) ;
Shape cachedShape_ = (castReceiver.getShape());
VarHandle.storeStoreFence();
this.cachedShape = cachedShape_;
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
return CompilerDirectives.isExact(receiver, this.receiverClass_) && accepts_(receiver);
}
private DynamicObjectLibrary getFallback_(DynamicObject receiver) {
DynamicObjectLibrary localFallback = this.fallback_;
if (localFallback == null) {
CompilerDirectives.transferToInterpreterAndInvalidate();
this.fallback_ = localFallback = insert(DYNAMIC_OBJECT_LIBRARY_.createDispatched((5)));
}
return localFallback;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#accepts}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
private boolean accepts_(Object arg0Value_) {
DynamicObject arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
return DynamicObjectLibraryImpl.accepts(arg0Value, this.cachedShape);
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getShape}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Shape getShape(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.getShape(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).getShape(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getOrDefault}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Object getOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.getOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.getOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getOrDefaultNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).getOrDefault(arg0Value, arg1Value, arg2Value);
}
}
private Object getOrDefaultNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'getOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[DynamicObjectLibraryImpl.getOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.getOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getIntOrDefault}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public int getIntOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.getIntOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.getIntOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getIntOrDefaultNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).getIntOrDefault(arg0Value, arg1Value, arg2Value);
}
}
private int getIntOrDefaultNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'getIntOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[DynamicObjectLibraryImpl.getIntOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.getIntOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getDoubleOrDefault}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public double getDoubleOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.getDoubleOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.getDoubleOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getDoubleOrDefaultNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).getDoubleOrDefault(arg0Value, arg1Value, arg2Value);
}
}
private double getDoubleOrDefaultNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'getDoubleOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[DynamicObjectLibraryImpl.getDoubleOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.getDoubleOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getLongOrDefault}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public long getLongOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.getLongOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.getLongOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getLongOrDefaultNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).getLongOrDefault(arg0Value, arg1Value, arg2Value);
}
}
private long getLongOrDefaultNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'getLongOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[DynamicObjectLibraryImpl.getLongOrDefault(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.getLongOrDefault(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#containsKey}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean containsKey(DynamicObject arg0Value, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.containsKey(DynamicObject, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.containsKey(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return containsKeyNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).containsKey(arg0Value, arg1Value);
}
}
private boolean containsKeyNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'containsKey(DynamicObject, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[DynamicObjectLibraryImpl.containsKey(DynamicObject, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.containsKey(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#put}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void put(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.put(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.put(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
} else {
getFallback_(arg0Value).put(arg0Value, arg1Value, arg2Value);
return;
}
}
private void putNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'put(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[DynamicObjectLibraryImpl.put(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.put(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putInt}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void putInt(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putInt(DynamicObject, Object, int, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.putInt(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putIntNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
} else {
getFallback_(arg0Value).putInt(arg0Value, arg1Value, arg2Value);
return;
}
}
private void putIntNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putInt(DynamicObject, Object, int, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putInt(DynamicObject, Object, int, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.putInt(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putLong}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void putLong(DynamicObject arg0Value, Object arg1Value, long arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putLong(DynamicObject, Object, long, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.putLong(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putLongNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
} else {
getFallback_(arg0Value).putLong(arg0Value, arg1Value, arg2Value);
return;
}
}
private void putLongNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, long arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putLong(DynamicObject, Object, long, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putLong(DynamicObject, Object, long, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.putLong(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putDouble}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void putDouble(DynamicObject arg0Value, Object arg1Value, double arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putDouble(DynamicObject, Object, double, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.putDouble(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putDoubleNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
} else {
getFallback_(arg0Value).putDouble(arg0Value, arg1Value, arg2Value);
return;
}
}
private void putDoubleNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, double arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putDouble(DynamicObject, Object, double, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putDouble(DynamicObject, Object, double, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.putDouble(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putIfPresent}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean putIfPresent(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putIfPresent(DynamicObject, Object, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.putIfPresent(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return putIfPresentNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).putIfPresent(arg0Value, arg1Value, arg2Value);
}
}
private boolean putIfPresentNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putIfPresent(DynamicObject, Object, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putIfPresent(DynamicObject, Object, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.putIfPresent(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putWithFlags}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void putWithFlags(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putWithFlags(DynamicObject, Object, Object, int, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.putWithFlags(arg0Value, arg1Value, arg2Value, arg3Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putWithFlagsNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
} else {
getFallback_(arg0Value).putWithFlags(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
}
private void putWithFlagsNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putWithFlags(DynamicObject, Object, Object, int, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putWithFlags(DynamicObject, Object, Object, int, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.putWithFlags(arg0Value, arg1Value, arg2Value, arg3Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#putConstant}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public void putConstant(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.putConstant(DynamicObject, Object, Object, int, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
DynamicObjectLibraryImpl.putConstant(arg0Value, arg1Value, arg2Value, arg3Value, this.cachedShape, keyCache_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
putConstantNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
} else {
getFallback_(arg0Value).putConstant(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
}
private void putConstantNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'putConstant(DynamicObject, Object, Object, int, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.putConstant(DynamicObject, Object, Object, int, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
DynamicObjectLibraryImpl.putConstant(arg0Value, arg1Value, arg2Value, arg3Value, this.cachedShape, keyCache_);
return;
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getProperty}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Property getProperty(DynamicObject arg0Value, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.getProperty(DynamicObject, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.getProperty(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getPropertyNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).getProperty(arg0Value, arg1Value);
}
}
private Property getPropertyNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'getProperty(DynamicObject, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.getProperty(DynamicObject, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.getProperty(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#setPropertyFlags}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean setPropertyFlags(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.setPropertyFlags(DynamicObject, Object, int, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.setPropertyFlags(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return setPropertyFlagsNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
} else {
return getFallback_(arg0Value).setPropertyFlags(arg0Value, arg1Value, arg2Value);
}
}
private boolean setPropertyFlagsNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'setPropertyFlags(DynamicObject, Object, int, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.setPropertyFlags(DynamicObject, Object, int, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.setPropertyFlags(arg0Value, arg1Value, arg2Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#removeKey}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean removeKey(DynamicObject arg0Value, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[DynamicObjectLibraryImpl.removeKey(DynamicObject, Object, Shape, KeyCacheNode)] */) {
{
KeyCacheNode keyCache_ = this.keyCache;
if (keyCache_ != null) {
return DynamicObjectLibraryImpl.removeKey(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return removeKeyNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).removeKey(arg0Value, arg1Value);
}
}
private boolean removeKeyNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
KeyCacheNode keyCache_;
KeyCacheNode keyCache__shared = this.keyCache;
if (keyCache__shared != null) {
keyCache_ = keyCache__shared;
} else {
keyCache_ = this.insert((KeyCacheNode.create(arg0Value.getShape(), arg1Value)));
if (keyCache_ == null) {
throw new IllegalStateException("Specialization 'removeKey(DynamicObject, Object, Shape, KeyCacheNode)' contains a shared cache with name 'keyCache' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.keyCache == null) {
VarHandle.storeStoreFence();
this.keyCache = keyCache_;
}
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[DynamicObjectLibraryImpl.removeKey(DynamicObject, Object, Shape, KeyCacheNode)] */;
this.state_0_ = state_0;
return DynamicObjectLibraryImpl.removeKey(arg0Value, arg1Value, this.cachedShape, keyCache_);
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getDynamicType}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Object getDynamicType(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.getDynamicType(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).getDynamicType(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#setDynamicType}
* Activation probability: 0.03704
* With/without class size: 4/5 bytes
*
*/
@Override
public boolean setDynamicType(DynamicObject arg0Value, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
SetDynamicTypeNode_SetDynamicTypeData s0_ = this.setDynamicTypeNode__setDynamicType_cache;
if (s0_ != null) {
{
Node node__ = (s0_);
return DynamicObjectLibraryImpl.setDynamicType(arg0Value, arg1Value, node__, this.cachedShape, INLINED_SET_DYNAMIC_TYPE_NODE__SET_DYNAMIC_TYPE_SET_CACHE_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return setDynamicTypeNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).setDynamicType(arg0Value, arg1Value);
}
}
private boolean setDynamicTypeNode_AndSpecialize(DynamicObject arg0Value, Object arg1Value) {
{
Node node__ = null;
SetDynamicTypeNode_SetDynamicTypeData s0_ = this.insert(new SetDynamicTypeNode_SetDynamicTypeData());
node__ = (s0_);
VarHandle.storeStoreFence();
this.setDynamicTypeNode__setDynamicType_cache = s0_;
return DynamicObjectLibraryImpl.setDynamicType(arg0Value, arg1Value, node__, this.cachedShape, INLINED_SET_DYNAMIC_TYPE_NODE__SET_DYNAMIC_TYPE_SET_CACHE_);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getShapeFlags}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public int getShapeFlags(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.getShapeFlags(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).getShapeFlags(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#setShapeFlags}
* Activation probability: 0.03704
* With/without class size: 4/5 bytes
*
*/
@Override
public boolean setShapeFlags(DynamicObject arg0Value, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
SetShapeFlagsNode_SetShapeFlagsData s0_ = this.setShapeFlagsNode__setShapeFlags_cache;
if (s0_ != null) {
{
Node node__ = (s0_);
return DynamicObjectLibraryImpl.setShapeFlags(arg0Value, arg1Value, node__, this.cachedShape, INLINED_SET_SHAPE_FLAGS_NODE__SET_SHAPE_FLAGS_SET_CACHE_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return setShapeFlagsNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).setShapeFlags(arg0Value, arg1Value);
}
}
private boolean setShapeFlagsNode_AndSpecialize(DynamicObject arg0Value, int arg1Value) {
{
Node node__ = null;
SetShapeFlagsNode_SetShapeFlagsData s0_ = this.insert(new SetShapeFlagsNode_SetShapeFlagsData());
node__ = (s0_);
VarHandle.storeStoreFence();
this.setShapeFlagsNode__setShapeFlags_cache = s0_;
return DynamicObjectLibraryImpl.setShapeFlags(arg0Value, arg1Value, node__, this.cachedShape, INLINED_SET_SHAPE_FLAGS_NODE__SET_SHAPE_FLAGS_SET_CACHE_);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#isShared}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isShared(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.isShared(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).isShared(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#markShared}
* Activation probability: 0.03704
* With/without class size: 4/5 bytes
*
*/
@Override
public void markShared(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
MarkSharedNode_MarkSharedData s0_ = this.markSharedNode__markShared_cache;
if (s0_ != null) {
{
Node node__ = (s0_);
DynamicObjectLibraryImpl.markShared(arg0Value, node__, this.cachedShape, INLINED_MARK_SHARED_NODE__MARK_SHARED_SET_CACHE_);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
markSharedNode_AndSpecialize(arg0Value);
return;
} else {
getFallback_(arg0Value).markShared(arg0Value);
return;
}
}
private void markSharedNode_AndSpecialize(DynamicObject arg0Value) {
{
Node node__ = null;
MarkSharedNode_MarkSharedData s0_ = this.insert(new MarkSharedNode_MarkSharedData());
node__ = (s0_);
VarHandle.storeStoreFence();
this.markSharedNode__markShared_cache = s0_;
DynamicObjectLibraryImpl.markShared(arg0Value, node__, this.cachedShape, INLINED_MARK_SHARED_NODE__MARK_SHARED_SET_CACHE_);
return;
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#updateShape}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean updateShape(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.updateShape(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).updateShape(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#resetShape}
* Activation probability: 0.03704
* With/without class size: 4/5 bytes
*
*/
@Override
public boolean resetShape(DynamicObject arg0Value, Shape arg1Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
ResetShapeNode_ResetShapeData s0_ = this.resetShapeNode__resetShape_cache;
if (s0_ != null) {
{
Node node__ = (s0_);
return DynamicObjectLibraryImpl.resetShape(arg0Value, arg1Value, node__, this.cachedShape, INLINED_RESET_SHAPE_NODE__RESET_SHAPE_SET_CACHE_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return resetShapeNode_AndSpecialize(arg0Value, arg1Value);
} else {
return getFallback_(arg0Value).resetShape(arg0Value, arg1Value);
}
}
private boolean resetShapeNode_AndSpecialize(DynamicObject arg0Value, Shape arg1Value) {
{
Node node__ = null;
ResetShapeNode_ResetShapeData s0_ = this.insert(new ResetShapeNode_ResetShapeData());
node__ = (s0_);
VarHandle.storeStoreFence();
this.resetShapeNode__resetShape_cache = s0_;
return DynamicObjectLibraryImpl.resetShape(arg0Value, arg1Value, node__, this.cachedShape, INLINED_RESET_SHAPE_NODE__RESET_SHAPE_SET_CACHE_);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getKeyArray}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] getKeyArray(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.getKeyArray(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).getKeyArray(arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link DynamicObjectLibraryImpl#getPropertyArray}
* Activation probability: 0.03704
* With/without class size: 4/0 bytes
*
*/
@Override
public Property[] getPropertyArray(DynamicObject arg0Value) {
assert CompilerDirectives.isExact(arg0Value, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
if (this.accepts(arg0Value)) {
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return DynamicObjectLibraryImpl.getPropertyArray(arg0Value, this.cachedShape);
} else {
return getFallback_(arg0Value).getPropertyArray(arg0Value);
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
@DenyReplace
private static final class SetDynamicTypeNode_SetDynamicTypeData extends Node {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link DynamicObjectLibraryImpl#setDynamicType}
* Parameter: {@link SetDynamicTypeNode} setCache
* Inline method: {@link SetDynamicTypeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int setDynamicType_state_0_;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#setDynamicType}
* Parameter: {@link SetDynamicTypeNode} setCache
* Inline method: {@link SetDynamicTypeNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object setDynamicTypeNode__setDynamicType_setCache__field1_;
SetDynamicTypeNode_SetDynamicTypeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
@DenyReplace
private static final class SetShapeFlagsNode_SetShapeFlagsData extends Node {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link DynamicObjectLibraryImpl#setShapeFlags}
* Parameter: {@link SetFlagsNode} setCache
* Inline method: {@link SetFlagsNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int setShapeFlags_state_0_;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#setShapeFlags}
* Parameter: {@link SetFlagsNode} setCache
* Inline method: {@link SetFlagsNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object setShapeFlagsNode__setShapeFlags_setCache__field1_;
SetShapeFlagsNode_SetShapeFlagsData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
@DenyReplace
private static final class MarkSharedNode_MarkSharedData extends Node {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link DynamicObjectLibraryImpl#markShared}
* Parameter: {@link MakeSharedNode} setCache
* Inline method: {@link MakeSharedNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int markShared_state_0_;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#markShared}
* Parameter: {@link MakeSharedNode} setCache
* Inline method: {@link MakeSharedNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object markSharedNode__markShared_setCache__field1_;
MarkSharedNode_MarkSharedData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
@DenyReplace
private static final class ResetShapeNode_ResetShapeData extends Node {
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link DynamicObjectLibraryImpl#resetShape}
* Parameter: {@link ResetShapeNode} setCache
* Inline method: {@link ResetShapeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int resetShape_state_0_;
/**
* Source Info:
* Specialization: {@link DynamicObjectLibraryImpl#resetShape}
* Parameter: {@link ResetShapeNode} setCache
* Inline method: {@link ResetShapeNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object resetShapeNode__resetShape_setCache__field1_;
ResetShapeNode_ResetShapeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
@GeneratedBy(DynamicObjectLibraryImpl.class)
@DenyReplace
private static final class Uncached extends DynamicObjectLibrary {
private final Class extends DynamicObject> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((DynamicObject) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return CompilerDirectives.isExact(receiver, this.receiverClass_) && accepts_(receiver);
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public Shape getShape(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getShape(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public Object getOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getOrDefault(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public int getIntOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getIntOrDefault(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public double getDoubleOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getDoubleOrDefault(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public long getLongOrDefault(DynamicObject arg0Value, Object arg1Value, Object arg2Value) throws UnexpectedResultException {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getLongOrDefault(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean containsKey(DynamicObject arg0Value, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.containsKey(arg0Value, arg1Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public void put(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.put(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void putInt(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.putInt(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void putLong(DynamicObject arg0Value, Object arg1Value, long arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.putLong(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void putDouble(DynamicObject arg0Value, Object arg1Value, double arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.putDouble(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean putIfPresent(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.putIfPresent(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public void putWithFlags(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.putWithFlags(arg0Value, arg1Value, arg2Value, arg3Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void putConstant(DynamicObject arg0Value, Object arg1Value, Object arg2Value, int arg3Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.putConstant(arg0Value, arg1Value, arg2Value, arg3Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public Property getProperty(DynamicObject arg0Value, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getProperty(arg0Value, arg1Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean setPropertyFlags(DynamicObject arg0Value, Object arg1Value, int arg2Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.setPropertyFlags(arg0Value, arg1Value, arg2Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean removeKey(DynamicObject arg0Value, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.removeKey(arg0Value, arg1Value, (arg0Value.getShape()), (KeyCacheNode.getUncached()));
}
@TruffleBoundary
@Override
public Object getDynamicType(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getDynamicType(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public boolean setDynamicType(DynamicObject arg0Value, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.setDynamicType(arg0Value, arg1Value, (this), (arg0Value.getShape()), (SetDynamicTypeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public int getShapeFlags(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getShapeFlags(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public boolean setShapeFlags(DynamicObject arg0Value, int arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.setShapeFlags(arg0Value, arg1Value, (this), (arg0Value.getShape()), (SetFlagsNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public boolean isShared(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.isShared(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public void markShared(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
DynamicObjectLibraryImpl.markShared(arg0Value, (this), (arg0Value.getShape()), (MakeSharedNodeGen.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean updateShape(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.updateShape(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public boolean resetShape(DynamicObject arg0Value, Shape arg1Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.resetShape(arg0Value, arg1Value, (this), (arg0Value.getShape()), (ResetShapeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public Object[] getKeyArray(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getKeyArray(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
@Override
public Property[] getPropertyArray(DynamicObject arg0Value) {
// declared: true
assert this.accepts(arg0Value) : "Invalid library usage. Library does not accept given receiver.";
return DynamicObjectLibraryImpl.getPropertyArray(arg0Value, (arg0Value.getShape()));
}
@TruffleBoundary
private static boolean accepts_(Object arg0Value_) {
DynamicObject arg0Value = ((DynamicObject) arg0Value_);
return DynamicObjectLibraryImpl.accepts(arg0Value, (arg0Value.getShape()));
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy