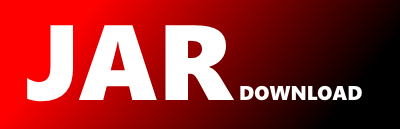
org.pkl.thirdparty.truffle.polyglot.PolyglotIterableFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pkl-tools Show documentation
Show all versions of pkl-tools Show documentation
Fat Jar containing pkl-cli, pkl-codegen-java, pkl-codegen-kotlin, pkl-config-java, pkl-core, pkl-doc, and their shaded third-party dependencies.
// CheckStyle: start generated
package org.pkl.thirdparty.truffle.polyglot;
import org.pkl.thirdparty.truffle.api.CompilerDirectives;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.CompilationFinal;
import org.pkl.thirdparty.truffle.api.CompilerDirectives.TruffleBoundary;
import org.pkl.thirdparty.truffle.api.dsl.GeneratedBy;
import org.pkl.thirdparty.truffle.api.dsl.NeverDefault;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.InlineTarget;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.ReferenceField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.StateField;
import org.pkl.thirdparty.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import org.pkl.thirdparty.truffle.api.interop.InteropLibrary;
import org.pkl.thirdparty.truffle.api.library.LibraryFactory;
import org.pkl.thirdparty.truffle.api.nodes.DenyReplace;
import org.pkl.thirdparty.truffle.api.nodes.EncapsulatingNodeReference;
import org.pkl.thirdparty.truffle.api.nodes.ExplodeLoop;
import org.pkl.thirdparty.truffle.api.nodes.Node;
import org.pkl.thirdparty.truffle.api.nodes.NodeCost;
import org.pkl.thirdparty.truffle.api.profiles.InlinedBranchProfile;
import org.pkl.thirdparty.truffle.polyglot.PolyglotIterable.Cache;
import org.pkl.thirdparty.truffle.polyglot.PolyglotIterable.Cache.GetIteratorNode;
import org.pkl.thirdparty.truffle.polyglot.PolyglotIterable.Cache.PolyglotIterableNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
@GeneratedBy(PolyglotIterable.class)
@SuppressWarnings({"javadoc", "unused"})
final class PolyglotIterableFactory {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@GeneratedBy(Cache.class)
static final class CacheFactory {
/**
* Debug Info:
* Specialization {@link GetIteratorNode#doCached}
* Activation probability: 0.65000
* With/without class size: 27/13 bytes
* Specialization {@link GetIteratorNode#doCached}
* Activation probability: 0.35000
* With/without class size: 13/9 bytes
*
*/
@GeneratedBy(GetIteratorNode.class)
@SuppressWarnings("javadoc")
static final class GetIteratorNodeGen extends GetIteratorNode {
private static final StateField CACHED0_CACHED0_STATE_0_UPDATER = StateField.create(Cached0Data.lookup_(), "cached0_state_0_");
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField CACHED0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cached0_cache", Cached0Data.class);
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
*/
private static final PolyglotToHostNode INLINED_CACHED0_TO_HOST_ = PolyglotToHostNodeGen.inline(InlineTarget.create(PolyglotToHostNode.class, CACHED0_CACHED0_STATE_0_UPDATER.subUpdater(0, 4), ReferenceField.create(Cached0Data.lookup_(), "cached0_toHost__field1_", Object.class), ReferenceField.create(Cached0Data.lookup_(), "cached0_toHost__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED0_ERROR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED0_CACHED0_STATE_0_UPDATER.subUpdater(4, 1)));
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
*/
private static final PolyglotToHostNode INLINED_CACHED1_TO_HOST_ = PolyglotToHostNodeGen.inline(InlineTarget.create(PolyglotToHostNode.class, STATE_0_UPDATER.subUpdater(2, 4), ReferenceField.create(MethodHandles.lookup(), "cached1_toHost__field1_", Object.class), ReferenceField.create(MethodHandles.lookup(), "cached1_toHost__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED1_ERROR_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(6, 1)));
/**
* State Info:
* 0: SpecializationActive {@link GetIteratorNode#doCached}
* 1: SpecializationActive {@link GetIteratorNode#doCached}
* 2-5: InlinedCache
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* 6: InlinedCache
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
@UnsafeAccessedField @Child private Cached0Data cached0_cache;
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object cached1_toHost__field1_;
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node cached1_toHost__field2_;
private GetIteratorNodeGen(Cache cache) {
super(cache);
}
@ExplodeLoop
@Override
protected Object executeImpl(PolyglotLanguageContext arg0Value, Object arg1Value, Object[] arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] || SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */) {
Cached0Data s0_ = this.cached0_cache;
while (s0_ != null) {
if ((s0_.iterables_.accepts(arg1Value))) {
Node node__ = (s0_);
return doCached(arg0Value, arg1Value, arg2Value, node__, s0_.iterables_, INLINED_CACHED0_TO_HOST_, INLINED_CACHED0_ERROR_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */) {
return this.cached1Boundary(state_0, arg0Value, arg1Value, arg2Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object cached1Boundary(int state_0, PolyglotLanguageContext arg0Value, Object arg1Value, Object[] arg2Value) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
Node node__ = (this);
InteropLibrary iterables__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
return doCached(arg0Value, arg1Value, arg2Value, node__, iterables__, INLINED_CACHED1_TO_HOST_, INLINED_CACHED1_ERROR_);
}
} finally {
encapsulating_.set(prev_);
}
}
private Object executeAndSpecialize(PolyglotLanguageContext arg0Value, Object arg1Value, Object[] arg2Value) {
int state_0 = this.state_0_;
{
Node node__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */) {
while (true) {
int count0_ = 0;
Cached0Data s0_ = CACHED0_CACHE_UPDATER.getVolatile(this);
Cached0Data s0_original = s0_;
while (s0_ != null) {
if ((s0_.iterables_.accepts(arg1Value))) {
node__ = (s0_);
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert (s0_.iterables_.accepts(arg1Value));
if (count0_ < (PolyglotIterableNode.LIMIT)) {
s0_ = this.insert(new Cached0Data(s0_original));
node__ = (s0_);
InteropLibrary iterables__ = s0_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(iterables__, "Specialization 'doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)' cache 'iterables' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.iterables_ = iterables__;
if (!CACHED0_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */;
this.state_0_ = state_0;
}
}
if (s0_ != null) {
return doCached(arg0Value, arg1Value, arg2Value, node__, s0_.iterables_, INLINED_CACHED0_TO_HOST_, INLINED_CACHED0_ERROR_);
}
break;
}
}
}
{
InteropLibrary iterables__ = null;
Node node__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
node__ = (this);
iterables__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
this.cached0_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[Cache.GetIteratorNode.doCached(PolyglotLanguageContext, Object, Object[], Node, InteropLibrary, PolyglotToHostNode, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return doCached(arg0Value, arg1Value, arg2Value, node__, iterables__, INLINED_CACHED1_TO_HOST_, INLINED_CACHED1_ERROR_);
} finally {
encapsulating_.set(prev_);
}
}
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11) & ((state_0 & 0b11) - 1)) == 0 /* is-single */) {
Cached0Data s0_ = this.cached0_cache;
if ((s0_ == null || s0_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static GetIteratorNode create(Cache cache) {
return new GetIteratorNodeGen(cache);
}
@GeneratedBy(GetIteratorNode.class)
@DenyReplace
private static final class Cached0Data extends Node {
@Child Cached0Data next_;
/**
* State Info:
* 0-3: InlinedCache
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* 4: InlinedCache
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link InlinedBranchProfile} error
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cached0_state_0_;
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link InteropLibrary} iterables
*/
@Child InteropLibrary iterables_;
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object cached0_toHost__field1_;
/**
* Source Info:
* Specialization: {@link GetIteratorNode#doCached}
* Parameter: {@link PolyglotToHostNode} toHost
* Inline method: {@link PolyglotToHostNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node cached0_toHost__field2_;
Cached0Data(Cached0Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy