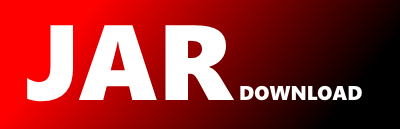
anorm.FunctionAdapter.scala Maven / Gradle / Ivy
The newest version!
package anorm
/** Function adapters to work with extract data. */
private[anorm] trait FunctionAdapter {
/**
* Returns function application with a single column.
* @tparam T1 the type of column
* @tparam R the type of result from `f` applied with the column
* @param f the function applied with column
*/
def to[T1, R](f: Function1[T1, R]): T1 => R = f
/**
* Returns function applicable with a 2-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, R](f: Function2[T1, T2, R]): T1 ~ T2 => R = { case c1 ~ c2 => f(c1, c2) }
/**
* Returns function applicable with a 3-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, R](f: Function3[T1, T2, T3, R]): T1 ~ T2 ~ T3 => R = { case c1 ~ c2 ~ c3 => f(c1, c2, c3) }
/**
* Returns function applicable with a 4-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, R](f: Function4[T1, T2, T3, T4, R]): T1 ~ T2 ~ T3 ~ T4 => R = { case c1 ~ c2 ~ c3 ~ c4 => f(c1, c2, c3, c4) }
/**
* Returns function applicable with a 5-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, R](f: Function5[T1, T2, T3, T4, T5, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 => f(c1, c2, c3, c4, c5) }
/**
* Returns function applicable with a 6-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, R](f: Function6[T1, T2, T3, T4, T5, T6, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 => f(c1, c2, c3, c4, c5, c6) }
/**
* Returns function applicable with a 7-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, R](f: Function7[T1, T2, T3, T4, T5, T6, T7, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 => f(c1, c2, c3, c4, c5, c6, c7) }
/**
* Returns function applicable with a 8-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, R](f: Function8[T1, T2, T3, T4, T5, T6, T7, T8, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 => f(c1, c2, c3, c4, c5, c6, c7, c8) }
/**
* Returns function applicable with a 9-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, R](f: Function9[T1, T2, T3, T4, T5, T6, T7, T8, T9, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9) }
/**
* Returns function applicable with a 10-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, R](f: Function10[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10) }
/**
* Returns function applicable with a 11-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, R](f: Function11[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11) }
/**
* Returns function applicable with a 12-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, R](f: Function12[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12) }
/**
* Returns function applicable with a 13-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, R](f: Function13[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13) }
/**
* Returns function applicable with a 14-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, R](f: Function14[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14) }
/**
* Returns function applicable with a 15-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, R](f: Function15[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15) }
/**
* Returns function applicable with a 16-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, R](f: Function16[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16) }
/**
* Returns function applicable with a 17-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, R](f: Function17[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17) }
/**
* Returns function applicable with a 18-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam T18 the type of column #18
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, R](f: Function18[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 ~ T18 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 ~ c18 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17, c18) }
/**
* Returns function applicable with a 19-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam T18 the type of column #18
* @tparam T19 the type of column #19
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, R](f: Function19[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 ~ T18 ~ T19 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 ~ c18 ~ c19 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17, c18, c19) }
/**
* Returns function applicable with a 20-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam T18 the type of column #18
* @tparam T19 the type of column #19
* @tparam T20 the type of column #20
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, R](f: Function20[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 ~ T18 ~ T19 ~ T20 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 ~ c18 ~ c19 ~ c20 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17, c18, c19, c20) }
/**
* Returns function applicable with a 21-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam T18 the type of column #18
* @tparam T19 the type of column #19
* @tparam T20 the type of column #20
* @tparam T21 the type of column #21
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, R](f: Function21[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 ~ T18 ~ T19 ~ T20 ~ T21 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 ~ c18 ~ c19 ~ c20 ~ c21 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17, c18, c19, c20, c21) }
/**
* Returns function applicable with a 22-column tuple-like.
* @tparam T1 the type of column #1
* @tparam T2 the type of column #2
* @tparam T3 the type of column #3
* @tparam T4 the type of column #4
* @tparam T5 the type of column #5
* @tparam T6 the type of column #6
* @tparam T7 the type of column #7
* @tparam T8 the type of column #8
* @tparam T9 the type of column #9
* @tparam T10 the type of column #10
* @tparam T11 the type of column #11
* @tparam T12 the type of column #12
* @tparam T13 the type of column #13
* @tparam T14 the type of column #14
* @tparam T15 the type of column #15
* @tparam T16 the type of column #16
* @tparam T17 the type of column #17
* @tparam T18 the type of column #18
* @tparam T19 the type of column #19
* @tparam T20 the type of column #20
* @tparam T21 the type of column #21
* @tparam T22 the type of column #22
* @tparam R the type of result from `f` applied with the columns
* @param f the function applied with columns
*/
def to[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22, R](f: Function22[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22, R]): T1 ~ T2 ~ T3 ~ T4 ~ T5 ~ T6 ~ T7 ~ T8 ~ T9 ~ T10 ~ T11 ~ T12 ~ T13 ~ T14 ~ T15 ~ T16 ~ T17 ~ T18 ~ T19 ~ T20 ~ T21 ~ T22 => R = { case c1 ~ c2 ~ c3 ~ c4 ~ c5 ~ c6 ~ c7 ~ c8 ~ c9 ~ c10 ~ c11 ~ c12 ~ c13 ~ c14 ~ c15 ~ c16 ~ c17 ~ c18 ~ c19 ~ c20 ~ c21 ~ c22 => f(c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14, c15, c16, c17, c18, c19, c20, c21, c22) }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy