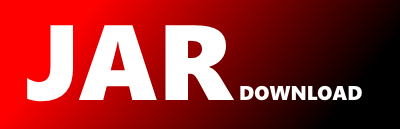
org.ploin.web.flow.Flow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ploinFaces Show documentation
Show all versions of ploinFaces Show documentation
With ploinFaces you can define a flow/conversation for a JSF-App. A flow contains views and attributes. If you leave the flow, the attributes will be removed from the http-session. This is similar to SpringWebFlow, but much easier und designed for JSF!
The newest version!
/**
* Copyright [2008] [PLOIN GmbH -> http://www.ploin.de]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.ploin.web.flow;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
/**
* $LastChangedBy: r.reiz $
* $Revision: 91 $
* $Date: 2010-01-18 22:26:53 +0100 (Mon, 18 Jan 2010) $
*/
public class Flow implements Serializable{
private static final long serialVersionUID = -7628817559417313411L;
private String flowId = "";
private Set views = new HashSet();
private Set attributes = new HashSet();
private Set ignoreViews = new HashSet();
private Set includeAuthoritys = new HashSet();
private Set excludeAuthoritys = new HashSet();
private Set subFlows = new HashSet();
private Boolean disableUrlNavigation = Boolean.FALSE;
private String authoritySource = null;
private String appName = null;
private String accessDeniedPage = null;
private String beforeFlowAction = null;
private String afterFlowAction = null;
private String beforeLifecycleAction = null;
private String afterLifecycleAction = null;
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Flow flow = (Flow) o;
if (flowId != null ? !flowId.equals(flow.flowId) : flow.flowId != null) return false;
return true;
}
public int hashCode() {
return (flowId != null ? flowId.hashCode() : 0);
}
public Set getSubFlows() {
return subFlows;
}
public void setSubFlows(Set subFlows) {
this.subFlows = subFlows;
}
public boolean addSubFlow(String flowId){
return getSubFlows().add(flowId);
}
public String getAfterFlowAction() {
return afterFlowAction;
}
public void setAfterFlowAction(String afterFlowAction) {
this.afterFlowAction = afterFlowAction;
}
public String getAfterLifecycleAction() {
return afterLifecycleAction;
}
public void setAfterLifecycleAction(String afterLifecycleAction) {
this.afterLifecycleAction = afterLifecycleAction;
}
public String getBeforeFlowAction() {
return beforeFlowAction;
}
public void setBeforeFlowAction(String beforeFlowAction) {
this.beforeFlowAction = beforeFlowAction;
}
public String getBeforeLifecycleAction() {
return beforeLifecycleAction;
}
public void setBeforeLifecycleAction(String beforeLifecycleAction) {
this.beforeLifecycleAction = beforeLifecycleAction;
}
public Set getViews() {
return views;
}
public void setViews(Set views) {
this.views = views;
}
public boolean addView(String view){
if (getViews() == null){
setViews(new HashSet());
}
return getViews().add(view);
}
public Set getAttributes() {
return attributes;
}
public void setAttributes(Set attributes) {
this.attributes = attributes;
}
public boolean addAttribute(String attribute){
if (getAttributes() == null){
setAttributes(new HashSet());
}
return getAttributes().add(attribute);
}
public boolean viewContains(String view) {
return views.contains(view);
}
public boolean attributeContains(String attribute) {
return attributes.contains(attribute);
}
public Set getIncludeAuthoritys() {
return includeAuthoritys;
}
public void setIncludeAuthoritys(Set includeAuthoritys) {
this.includeAuthoritys = includeAuthoritys;
}
public boolean addIncludeAuthority(String auth){
if (getIncludeAuthoritys() == null){
setIncludeAuthoritys(new HashSet());
}
return getIncludeAuthoritys().add(auth);
}
public boolean includeContains(String auth){
return includeAuthoritys.contains(auth);
}
public Set getExcludeAuthoritys() {
return excludeAuthoritys;
}
public void setExcludeAuthoritys(Set excludeAuthoritys) {
this.excludeAuthoritys = excludeAuthoritys;
}
public boolean addExcludeAuthority(String auth){
if (getExcludeAuthoritys() == null){
setExcludeAuthoritys(new HashSet());
}
return getExcludeAuthoritys().add(auth);
}
public boolean excludeContains(String auth){
return excludeAuthoritys.contains(auth);
}
public String getAuthoritySource() {
return authoritySource;
}
public void setAuthoritySource(String authoritySource) {
this.authoritySource = authoritySource;
}
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public String getAccessDeniedPage() {
return accessDeniedPage;
}
public void setAccessDeniedPage(String accessDeniedPage) {
this.accessDeniedPage = accessDeniedPage;
}
public String getFlowId() {
return flowId;
}
public void setFlowId(String flowId) {
this.flowId = flowId;
}
public Set getIgnoreViews() {
return ignoreViews;
}
public void setIgnoreViews(Set ignoreViews) {
this.ignoreViews = ignoreViews;
}
public boolean addIgnoreView(String view){
if (getIgnoreViews() == null){
setIgnoreViews(new HashSet());
}
return getIgnoreViews().add(view);
}
public Boolean getDisableUrlNavigation() {
return disableUrlNavigation;
}
public void setDisableUrlNavigation(Boolean disableUrlNavigation) {
this.disableUrlNavigation = disableUrlNavigation;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy