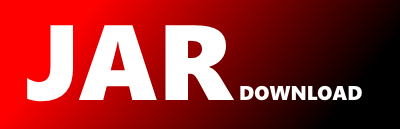
org.plutext.jaxb.svg11.ObjectFactory Maven / Gradle / Ivy
Show all versions of jaxb-svg11 Show documentation
/*
* Copyright 2010, Plutext Pty Ltd.
*
* This file is part of docx4j.
docx4j is licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package org.plutext.jaxb.svg11;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.plutext.jaxb.svg11 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _SVGMarkerClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Marker.class");
private final static QName _SVGMaskClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Mask.class");
private final static QName _Pattern_QNAME = new QName("http://www.w3.org/2000/svg", "pattern");
private final static QName _FeGaussianBlur_QNAME = new QName("http://www.w3.org/2000/svg", "feGaussianBlur");
private final static QName _Tspan_QNAME = new QName("http://www.w3.org/2000/svg", "tspan");
private final static QName _SVGFontClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Font.class");
private final static QName _SVGConditionalClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Conditional.class");
private final static QName _FeConvolveMatrix_QNAME = new QName("http://www.w3.org/2000/svg", "feConvolveMatrix");
private final static QName _TextPath_QNAME = new QName("http://www.w3.org/2000/svg", "textPath");
private final static QName _Svg_QNAME = new QName("http://www.w3.org/2000/svg", "svg");
private final static QName _FeImage_QNAME = new QName("http://www.w3.org/2000/svg", "feImage");
private final static QName _A_QNAME = new QName("http://www.w3.org/2000/svg", "a");
private final static QName _SVGUseClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Use.class");
private final static QName _G_QNAME = new QName("http://www.w3.org/2000/svg", "g");
private final static QName _SVGGradientClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Gradient.class");
private final static QName _SVGAnimationClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Animation.class");
private final static QName _Defs_QNAME = new QName("http://www.w3.org/2000/svg", "defs");
private final static QName _FeDiffuseLighting_QNAME = new QName("http://www.w3.org/2000/svg", "feDiffuseLighting");
private final static QName _SVGColorProfileClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.ColorProfile.class");
private final static QName _AltGlyph_QNAME = new QName("http://www.w3.org/2000/svg", "altGlyph");
private final static QName _Path_QNAME = new QName("http://www.w3.org/2000/svg", "path");
private final static QName _Style_QNAME = new QName("http://www.w3.org/2000/svg", "style");
private final static QName _Polyline_QNAME = new QName("http://www.w3.org/2000/svg", "polyline");
private final static QName _SVGStructureClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Structure.class");
private final static QName _FeSpecularLighting_QNAME = new QName("http://www.w3.org/2000/svg", "feSpecularLighting");
private final static QName _Title_QNAME = new QName("http://www.w3.org/2000/svg", "title");
private final static QName _SVGStyleClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Style.class");
private final static QName _SVGHyperlinkClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Hyperlink.class");
private final static QName _SVGPatternClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Pattern.class");
private final static QName _ColorProfile_QNAME = new QName("http://www.w3.org/2000/svg", "color-profile");
private final static QName _Line_QNAME = new QName("http://www.w3.org/2000/svg", "line");
private final static QName _Use_QNAME = new QName("http://www.w3.org/2000/svg", "use");
private final static QName _Circle_QNAME = new QName("http://www.w3.org/2000/svg", "circle");
private final static QName _Switch_QNAME = new QName("http://www.w3.org/2000/svg", "switch");
private final static QName _FeOffset_QNAME = new QName("http://www.w3.org/2000/svg", "feOffset");
private final static QName _FeMerge_QNAME = new QName("http://www.w3.org/2000/svg", "feMerge");
private final static QName _Set_QNAME = new QName("http://www.w3.org/2000/svg", "set");
private final static QName _SVGShapeClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Shape.class");
private final static QName _SVGTextClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Text.class");
private final static QName _Desc_QNAME = new QName("http://www.w3.org/2000/svg", "desc");
private final static QName _Filter_QNAME = new QName("http://www.w3.org/2000/svg", "filter");
private final static QName _FontFace_QNAME = new QName("http://www.w3.org/2000/svg", "font-face");
private final static QName _View_QNAME = new QName("http://www.w3.org/2000/svg", "view");
private final static QName _Marker_QNAME = new QName("http://www.w3.org/2000/svg", "marker");
private final static QName _AnimateColor_QNAME = new QName("http://www.w3.org/2000/svg", "animateColor");
private final static QName _SVGDescriptionClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Description.class");
private final static QName _SVGFilterClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Filter.class");
private final static QName _FeComponentTransfer_QNAME = new QName("http://www.w3.org/2000/svg", "feComponentTransfer");
private final static QName _AnimateMotion_QNAME = new QName("http://www.w3.org/2000/svg", "animateMotion");
private final static QName _AltGlyphDef_QNAME = new QName("http://www.w3.org/2000/svg", "altGlyphDef");
private final static QName _Script_QNAME = new QName("http://www.w3.org/2000/svg", "script");
private final static QName _Rect_QNAME = new QName("http://www.w3.org/2000/svg", "rect");
private final static QName _Image_QNAME = new QName("http://www.w3.org/2000/svg", "image");
private final static QName _SVGImageClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Image.class");
private final static QName _Symbol_QNAME = new QName("http://www.w3.org/2000/svg", "symbol");
private final static QName _LinearGradient_QNAME = new QName("http://www.w3.org/2000/svg", "linearGradient");
private final static QName _FeDisplacementMap_QNAME = new QName("http://www.w3.org/2000/svg", "feDisplacementMap");
private final static QName _Font_QNAME = new QName("http://www.w3.org/2000/svg", "font");
private final static QName _RadialGradient_QNAME = new QName("http://www.w3.org/2000/svg", "radialGradient");
private final static QName _FeColorMatrix_QNAME = new QName("http://www.w3.org/2000/svg", "feColorMatrix");
private final static QName _Ellipse_QNAME = new QName("http://www.w3.org/2000/svg", "ellipse");
private final static QName _Text_QNAME = new QName("http://www.w3.org/2000/svg", "text");
private final static QName _SVGFilterPrimitiveClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.FilterPrimitive.class");
private final static QName _SVGTextContentClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.TextContent.class");
private final static QName _Metadata_QNAME = new QName("http://www.w3.org/2000/svg", "metadata");
private final static QName _Tref_QNAME = new QName("http://www.w3.org/2000/svg", "tref");
private final static QName _FeMorphology_QNAME = new QName("http://www.w3.org/2000/svg", "feMorphology");
private final static QName _FeBlend_QNAME = new QName("http://www.w3.org/2000/svg", "feBlend");
private final static QName _Polygon_QNAME = new QName("http://www.w3.org/2000/svg", "polygon");
private final static QName _AnimateTransform_QNAME = new QName("http://www.w3.org/2000/svg", "animateTransform");
private final static QName _Mask_QNAME = new QName("http://www.w3.org/2000/svg", "mask");
private final static QName _FeFlood_QNAME = new QName("http://www.w3.org/2000/svg", "feFlood");
private final static QName _FeTurbulence_QNAME = new QName("http://www.w3.org/2000/svg", "feTurbulence");
private final static QName _FeTile_QNAME = new QName("http://www.w3.org/2000/svg", "feTile");
private final static QName _SVGViewClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.View.class");
private final static QName _SVGScriptClass_QNAME = new QName("http://www.w3.org/2000/svg", "SVG.Script.class");
private final static QName _Animate_QNAME = new QName("http://www.w3.org/2000/svg", "animate");
private final static QName _FeComposite_QNAME = new QName("http://www.w3.org/2000/svg", "feComposite");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.plutext.jaxb.svg11
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link TextPath }
*
*/
public TextPath createTextPath() {
return new TextPath();
}
/**
* Create an instance of {@link Title }
*
*/
public Title createTitle() {
return new Title();
}
/**
* Create an instance of {@link SVGAnimateMotionContent }
*
*/
public SVGAnimateMotionContent createSVGAnimateMotionContent() {
return new SVGAnimateMotionContent();
}
/**
* Create an instance of {@link SVGMaskContent }
*
*/
public SVGMaskContent createSVGMaskContent() {
return new SVGMaskContent();
}
/**
* Create an instance of {@link SVGMarkerContent }
*
*/
public SVGMarkerContent createSVGMarkerContent() {
return new SVGMarkerContent();
}
/**
* Create an instance of {@link SVGFeOffsetContent }
*
*/
public SVGFeOffsetContent createSVGFeOffsetContent() {
return new SVGFeOffsetContent();
}
/**
* Create an instance of {@link SVGPatternClass }
*
*/
public SVGPatternClass createSVGPatternClass() {
return new SVGPatternClass();
}
/**
* Create an instance of {@link SVGClipPathContent }
*
*/
public SVGClipPathContent createSVGClipPathContent() {
return new SVGClipPathContent();
}
/**
* Create an instance of {@link FeFuncA }
*
*/
public FeFuncA createFeFuncA() {
return new FeFuncA();
}
/**
* Create an instance of {@link SVGDefsContent }
*
*/
public SVGDefsContent createSVGDefsContent() {
return new SVGDefsContent();
}
/**
* Create an instance of {@link FeOffset }
*
*/
public FeOffset createFeOffset() {
return new FeOffset();
}
/**
* Create an instance of {@link AltGlyphDef }
*
*/
public AltGlyphDef createAltGlyphDef() {
return new AltGlyphDef();
}
/**
* Create an instance of {@link FeDiffuseLighting }
*
*/
public FeDiffuseLighting createFeDiffuseLighting() {
return new FeDiffuseLighting();
}
/**
* Create an instance of {@link ClipPath }
*
*/
public ClipPath createClipPath() {
return new ClipPath();
}
/**
* Create an instance of {@link Polygon }
*
*/
public Polygon createPolygon() {
return new Polygon();
}
/**
* Create an instance of {@link FeConvolveMatrix }
*
*/
public FeConvolveMatrix createFeConvolveMatrix() {
return new FeConvolveMatrix();
}
/**
* Create an instance of {@link SVGAnimateContent }
*
*/
public SVGAnimateContent createSVGAnimateContent() {
return new SVGAnimateContent();
}
/**
* Create an instance of {@link FeDisplacementMap }
*
*/
public FeDisplacementMap createFeDisplacementMap() {
return new FeDisplacementMap();
}
/**
* Create an instance of {@link Rect }
*
*/
public Rect createRect() {
return new Rect();
}
/**
* Create an instance of {@link SVGFeFuncGContent }
*
*/
public SVGFeFuncGContent createSVGFeFuncGContent() {
return new SVGFeFuncGContent();
}
/**
* Create an instance of {@link SVGViewContent }
*
*/
public SVGViewContent createSVGViewContent() {
return new SVGViewContent();
}
/**
* Create an instance of {@link SVGFeFuncRContent }
*
*/
public SVGFeFuncRContent createSVGFeFuncRContent() {
return new SVGFeFuncRContent();
}
/**
* Create an instance of {@link FeComposite }
*
*/
public FeComposite createFeComposite() {
return new FeComposite();
}
/**
* Create an instance of {@link SVGFilterContent }
*
*/
public SVGFilterContent createSVGFilterContent() {
return new SVGFilterContent();
}
/**
* Create an instance of {@link SVGLineContent }
*
*/
public SVGLineContent createSVGLineContent() {
return new SVGLineContent();
}
/**
* Create an instance of {@link SVGAnimateTransformContent }
*
*/
public SVGAnimateTransformContent createSVGAnimateTransformContent() {
return new SVGAnimateTransformContent();
}
/**
* Create an instance of {@link SVGFeGaussianBlurContent }
*
*/
public SVGFeGaussianBlurContent createSVGFeGaussianBlurContent() {
return new SVGFeGaussianBlurContent();
}
/**
* Create an instance of {@link AltGlyph }
*
*/
public AltGlyph createAltGlyph() {
return new AltGlyph();
}
/**
* Create an instance of {@link FontFaceUri }
*
*/
public FontFaceUri createFontFaceUri() {
return new FontFaceUri();
}
/**
* Create an instance of {@link FeFuncR }
*
*/
public FeFuncR createFeFuncR() {
return new FeFuncR();
}
/**
* Create an instance of {@link Line }
*
*/
public Line createLine() {
return new Line();
}
/**
* Create an instance of {@link Set }
*
*/
public Set createSet() {
return new Set();
}
/**
* Create an instance of {@link SVGRectContent }
*
*/
public SVGRectContent createSVGRectContent() {
return new SVGRectContent();
}
/**
* Create an instance of {@link SVGSvgContent }
*
*/
public SVGSvgContent createSVGSvgContent() {
return new SVGSvgContent();
}
/**
* Create an instance of {@link AnimateMotion }
*
*/
public AnimateMotion createAnimateMotion() {
return new AnimateMotion();
}
/**
* Create an instance of {@link AnimateTransform }
*
*/
public AnimateTransform createAnimateTransform() {
return new AnimateTransform();
}
/**
* Create an instance of {@link SVGFilterClass }
*
*/
public SVGFilterClass createSVGFilterClass() {
return new SVGFilterClass();
}
/**
* Create an instance of {@link FeTurbulence }
*
*/
public FeTurbulence createFeTurbulence() {
return new FeTurbulence();
}
/**
* Create an instance of {@link SVGFeSpotLightContent }
*
*/
public SVGFeSpotLightContent createSVGFeSpotLightContent() {
return new SVGFeSpotLightContent();
}
/**
* Create an instance of {@link FeTile }
*
*/
public FeTile createFeTile() {
return new FeTile();
}
/**
* Create an instance of {@link Metadata }
*
*/
public Metadata createMetadata() {
return new Metadata();
}
/**
* Create an instance of {@link FeFuncB }
*
*/
public FeFuncB createFeFuncB() {
return new FeFuncB();
}
/**
* Create an instance of {@link FePointLight }
*
*/
public FePointLight createFePointLight() {
return new FePointLight();
}
/**
* Create an instance of {@link SVGMaskClass }
*
*/
public SVGMaskClass createSVGMaskClass() {
return new SVGMaskClass();
}
/**
* Create an instance of {@link SVGFeDisplacementMapContent }
*
*/
public SVGFeDisplacementMapContent createSVGFeDisplacementMapContent() {
return new SVGFeDisplacementMapContent();
}
/**
* Create an instance of {@link FeGaussianBlur }
*
*/
public FeGaussianBlur createFeGaussianBlur() {
return new FeGaussianBlur();
}
/**
* Create an instance of {@link SVGTextPathContent }
*
*/
public SVGTextPathContent createSVGTextPathContent() {
return new SVGTextPathContent();
}
/**
* Create an instance of {@link SVGUseClass }
*
*/
public SVGUseClass createSVGUseClass() {
return new SVGUseClass();
}
/**
* Create an instance of {@link FontFaceName }
*
*/
public FontFaceName createFontFaceName() {
return new FontFaceName();
}
/**
* Create an instance of {@link SVGPatternContent }
*
*/
public SVGPatternContent createSVGPatternContent() {
return new SVGPatternContent();
}
/**
* Create an instance of {@link SVGFePointLightContent }
*
*/
public SVGFePointLightContent createSVGFePointLightContent() {
return new SVGFePointLightContent();
}
/**
* Create an instance of {@link Stop }
*
*/
public Stop createStop() {
return new Stop();
}
/**
* Create an instance of {@link SVGFeSpecularLightingContent }
*
*/
public SVGFeSpecularLightingContent createSVGFeSpecularLightingContent() {
return new SVGFeSpecularLightingContent();
}
/**
* Create an instance of {@link FeSpotLight }
*
*/
public FeSpotLight createFeSpotLight() {
return new FeSpotLight();
}
/**
* Create an instance of {@link Glyph }
*
*/
public Glyph createGlyph() {
return new Glyph();
}
/**
* Create an instance of {@link Path }
*
*/
public Path createPath() {
return new Path();
}
/**
* Create an instance of {@link FeMorphology }
*
*/
public FeMorphology createFeMorphology() {
return new FeMorphology();
}
/**
* Create an instance of {@link ForeignObject }
*
*/
public ForeignObject createForeignObject() {
return new ForeignObject();
}
/**
* Create an instance of {@link FontFaceSrc }
*
*/
public FontFaceSrc createFontFaceSrc() {
return new FontFaceSrc();
}
/**
* Create an instance of {@link FeImage }
*
*/
public FeImage createFeImage() {
return new FeImage();
}
/**
* Create an instance of {@link FeDistantLight }
*
*/
public FeDistantLight createFeDistantLight() {
return new FeDistantLight();
}
/**
* Create an instance of {@link SVGViewClass }
*
*/
public SVGViewClass createSVGViewClass() {
return new SVGViewClass();
}
/**
* Create an instance of {@link AltGlyphItem }
*
*/
public AltGlyphItem createAltGlyphItem() {
return new AltGlyphItem();
}
/**
* Create an instance of {@link SVGSymbolContent }
*
*/
public SVGSymbolContent createSVGSymbolContent() {
return new SVGSymbolContent();
}
/**
* Create an instance of {@link SVGImageContent }
*
*/
public SVGImageContent createSVGImageContent() {
return new SVGImageContent();
}
/**
* Create an instance of {@link SVGMissingGlyphContent }
*
*/
public SVGMissingGlyphContent createSVGMissingGlyphContent() {
return new SVGMissingGlyphContent();
}
/**
* Create an instance of {@link Cursor }
*
*/
public Cursor createCursor() {
return new Cursor();
}
/**
* Create an instance of {@link Tref }
*
*/
public Tref createTref() {
return new Tref();
}
/**
* Create an instance of {@link SVGSetContent }
*
*/
public SVGSetContent createSVGSetContent() {
return new SVGSetContent();
}
/**
* Create an instance of {@link SVGGContent }
*
*/
public SVGGContent createSVGGContent() {
return new SVGGContent();
}
/**
* Create an instance of {@link SVGAnimateColorContent }
*
*/
public SVGAnimateColorContent createSVGAnimateColorContent() {
return new SVGAnimateColorContent();
}
/**
* Create an instance of {@link SVGFeFloodContent }
*
*/
public SVGFeFloodContent createSVGFeFloodContent() {
return new SVGFeFloodContent();
}
/**
* Create an instance of {@link MissingGlyph }
*
*/
public MissingGlyph createMissingGlyph() {
return new MissingGlyph();
}
/**
* Create an instance of {@link G }
*
*/
public G createG() {
return new G();
}
/**
* Create an instance of {@link SVGLinearGradientContent }
*
*/
public SVGLinearGradientContent createSVGLinearGradientContent() {
return new SVGLinearGradientContent();
}
/**
* Create an instance of {@link SVGPolylineContent }
*
*/
public SVGPolylineContent createSVGPolylineContent() {
return new SVGPolylineContent();
}
/**
* Create an instance of {@link SVGAltGlyphItemContent }
*
*/
public SVGAltGlyphItemContent createSVGAltGlyphItemContent() {
return new SVGAltGlyphItemContent();
}
/**
* Create an instance of {@link Hkern }
*
*/
public Hkern createHkern() {
return new Hkern();
}
/**
* Create an instance of {@link Symbol }
*
*/
public Symbol createSymbol() {
return new Symbol();
}
/**
* Create an instance of {@link FeSpecularLighting }
*
*/
public FeSpecularLighting createFeSpecularLighting() {
return new FeSpecularLighting();
}
/**
* Create an instance of {@link SVGTextContent }
*
*/
public SVGTextContent createSVGTextContent() {
return new SVGTextContent();
}
/**
* Create an instance of {@link SVGGlyphContent }
*
*/
public SVGGlyphContent createSVGGlyphContent() {
return new SVGGlyphContent();
}
/**
* Create an instance of {@link FeMergeNode }
*
*/
public FeMergeNode createFeMergeNode() {
return new FeMergeNode();
}
/**
* Create an instance of {@link SVGFeFuncAContent }
*
*/
public SVGFeFuncAContent createSVGFeFuncAContent() {
return new SVGFeFuncAContent();
}
/**
* Create an instance of {@link SVGFeCompositeContent }
*
*/
public SVGFeCompositeContent createSVGFeCompositeContent() {
return new SVGFeCompositeContent();
}
/**
* Create an instance of {@link SVGFontFaceUriContent }
*
*/
public SVGFontFaceUriContent createSVGFontFaceUriContent() {
return new SVGFontFaceUriContent();
}
/**
* Create an instance of {@link SVGTspanContent }
*
*/
public SVGTspanContent createSVGTspanContent() {
return new SVGTspanContent();
}
/**
* Create an instance of {@link Circle }
*
*/
public Circle createCircle() {
return new Circle();
}
/**
* Create an instance of {@link SVGFontContent }
*
*/
public SVGFontContent createSVGFontContent() {
return new SVGFontContent();
}
/**
* Create an instance of {@link SVGRadialGradientContent }
*
*/
public SVGRadialGradientContent createSVGRadialGradientContent() {
return new SVGRadialGradientContent();
}
/**
* Create an instance of {@link SVGColorProfileContent }
*
*/
public SVGColorProfileContent createSVGColorProfileContent() {
return new SVGColorProfileContent();
}
/**
* Create an instance of {@link Mpath }
*
*/
public Mpath createMpath() {
return new Mpath();
}
/**
* Create an instance of {@link AnimateColor }
*
*/
public AnimateColor createAnimateColor() {
return new AnimateColor();
}
/**
* Create an instance of {@link FontFace }
*
*/
public FontFace createFontFace() {
return new FontFace();
}
/**
* Create an instance of {@link SVGFeMorphologyContent }
*
*/
public SVGFeMorphologyContent createSVGFeMorphologyContent() {
return new SVGFeMorphologyContent();
}
/**
* Create an instance of {@link Defs }
*
*/
public Defs createDefs() {
return new Defs();
}
/**
* Create an instance of {@link Svg }
*
*/
public Svg createSvg() {
return new Svg();
}
/**
* Create an instance of {@link SVGFeMergeContent }
*
*/
public SVGFeMergeContent createSVGFeMergeContent() {
return new SVGFeMergeContent();
}
/**
* Create an instance of {@link SVGFeDiffuseLightingContent }
*
*/
public SVGFeDiffuseLightingContent createSVGFeDiffuseLightingContent() {
return new SVGFeDiffuseLightingContent();
}
/**
* Create an instance of {@link SVGSwitchContent }
*
*/
public SVGSwitchContent createSVGSwitchContent() {
return new SVGSwitchContent();
}
/**
* Create an instance of {@link SVGConditionalClass }
*
*/
public SVGConditionalClass createSVGConditionalClass() {
return new SVGConditionalClass();
}
/**
* Create an instance of {@link FeMerge }
*
*/
public FeMerge createFeMerge() {
return new FeMerge();
}
/**
* Create an instance of {@link FeFuncG }
*
*/
public FeFuncG createFeFuncG() {
return new FeFuncG();
}
/**
* Create an instance of {@link SVGStyleClass }
*
*/
public SVGStyleClass createSVGStyleClass() {
return new SVGStyleClass();
}
/**
* Create an instance of {@link Font }
*
*/
public Font createFont() {
return new Font();
}
/**
* Create an instance of {@link SVGAltGlyphDefContent }
*
*/
public SVGAltGlyphDefContent createSVGAltGlyphDefContent() {
return new SVGAltGlyphDefContent();
}
/**
* Create an instance of {@link SVGFeFuncBContent }
*
*/
public SVGFeFuncBContent createSVGFeFuncBContent() {
return new SVGFeFuncBContent();
}
/**
* Create an instance of {@link Text }
*
*/
public Text createText() {
return new Text();
}
/**
* Create an instance of {@link SVGImageClass }
*
*/
public SVGImageClass createSVGImageClass() {
return new SVGImageClass();
}
/**
* Create an instance of {@link SVGUseContent }
*
*/
public SVGUseContent createSVGUseContent() {
return new SVGUseContent();
}
/**
* Create an instance of {@link SVGHyperlinkClass }
*
*/
public SVGHyperlinkClass createSVGHyperlinkClass() {
return new SVGHyperlinkClass();
}
/**
* Create an instance of {@link SVGPathContent }
*
*/
public SVGPathContent createSVGPathContent() {
return new SVGPathContent();
}
/**
* Create an instance of {@link SVGFeConvolveMatrixContent }
*
*/
public SVGFeConvolveMatrixContent createSVGFeConvolveMatrixContent() {
return new SVGFeConvolveMatrixContent();
}
/**
* Create an instance of {@link SVGFontFaceSrcContent }
*
*/
public SVGFontFaceSrcContent createSVGFontFaceSrcContent() {
return new SVGFontFaceSrcContent();
}
/**
* Create an instance of {@link RadialGradient }
*
*/
public RadialGradient createRadialGradient() {
return new RadialGradient();
}
/**
* Create an instance of {@link SVGPolygonContent }
*
*/
public SVGPolygonContent createSVGPolygonContent() {
return new SVGPolygonContent();
}
/**
* Create an instance of {@link DefinitionSrc }
*
*/
public DefinitionSrc createDefinitionSrc() {
return new DefinitionSrc();
}
/**
* Create an instance of {@link SVGStopContent }
*
*/
public SVGStopContent createSVGStopContent() {
return new SVGStopContent();
}
/**
* Create an instance of {@link SVGFeColorMatrixContent }
*
*/
public SVGFeColorMatrixContent createSVGFeColorMatrixContent() {
return new SVGFeColorMatrixContent();
}
/**
* Create an instance of {@link SVGFontFaceContent }
*
*/
public SVGFontFaceContent createSVGFontFaceContent() {
return new SVGFontFaceContent();
}
/**
* Create an instance of {@link SVGAContent }
*
*/
public SVGAContent createSVGAContent() {
return new SVGAContent();
}
/**
* Create an instance of {@link SVGFeDistantLightContent }
*
*/
public SVGFeDistantLightContent createSVGFeDistantLightContent() {
return new SVGFeDistantLightContent();
}
/**
* Create an instance of {@link FeColorMatrix }
*
*/
public FeColorMatrix createFeColorMatrix() {
return new FeColorMatrix();
}
/**
* Create an instance of {@link Tspan }
*
*/
public Tspan createTspan() {
return new Tspan();
}
/**
* Create an instance of {@link SVGFeTurbulenceContent }
*
*/
public SVGFeTurbulenceContent createSVGFeTurbulenceContent() {
return new SVGFeTurbulenceContent();
}
/**
* Create an instance of {@link Animate }
*
*/
public Animate createAnimate() {
return new Animate();
}
/**
* Create an instance of {@link FeBlend }
*
*/
public FeBlend createFeBlend() {
return new FeBlend();
}
/**
* Create an instance of {@link SVGFeMergeNodeContent }
*
*/
public SVGFeMergeNodeContent createSVGFeMergeNodeContent() {
return new SVGFeMergeNodeContent();
}
/**
* Create an instance of {@link FeComponentTransfer }
*
*/
public FeComponentTransfer createFeComponentTransfer() {
return new FeComponentTransfer();
}
/**
* Create an instance of {@link SVGTrefContent }
*
*/
public SVGTrefContent createSVGTrefContent() {
return new SVGTrefContent();
}
/**
* Create an instance of {@link SVGFeComponentTransferContent }
*
*/
public SVGFeComponentTransferContent createSVGFeComponentTransferContent() {
return new SVGFeComponentTransferContent();
}
/**
* Create an instance of {@link SVGScriptClass }
*
*/
public SVGScriptClass createSVGScriptClass() {
return new SVGScriptClass();
}
/**
* Create an instance of {@link FeFlood }
*
*/
public FeFlood createFeFlood() {
return new FeFlood();
}
/**
* Create an instance of {@link SVGCursorContent }
*
*/
public SVGCursorContent createSVGCursorContent() {
return new SVGCursorContent();
}
/**
* Create an instance of {@link Polyline }
*
*/
public Polyline createPolyline() {
return new Polyline();
}
/**
* Create an instance of {@link FontFaceFormat }
*
*/
public FontFaceFormat createFontFaceFormat() {
return new FontFaceFormat();
}
/**
* Create an instance of {@link SVGMpathContent }
*
*/
public SVGMpathContent createSVGMpathContent() {
return new SVGMpathContent();
}
/**
* Create an instance of {@link SVGColorProfileClass }
*
*/
public SVGColorProfileClass createSVGColorProfileClass() {
return new SVGColorProfileClass();
}
/**
* Create an instance of {@link SVGFeImageContent }
*
*/
public SVGFeImageContent createSVGFeImageContent() {
return new SVGFeImageContent();
}
/**
* Create an instance of {@link Ellipse }
*
*/
public Ellipse createEllipse() {
return new Ellipse();
}
/**
* Create an instance of {@link SVGCircleContent }
*
*/
public SVGCircleContent createSVGCircleContent() {
return new SVGCircleContent();
}
/**
* Create an instance of {@link Desc }
*
*/
public Desc createDesc() {
return new Desc();
}
/**
* Create an instance of {@link GlyphRef }
*
*/
public GlyphRef createGlyphRef() {
return new GlyphRef();
}
/**
* Create an instance of {@link SVGFeBlendContent }
*
*/
public SVGFeBlendContent createSVGFeBlendContent() {
return new SVGFeBlendContent();
}
/**
* Create an instance of {@link SVGFeTileContent }
*
*/
public SVGFeTileContent createSVGFeTileContent() {
return new SVGFeTileContent();
}
/**
* Create an instance of {@link Vkern }
*
*/
public Vkern createVkern() {
return new Vkern();
}
/**
* Create an instance of {@link SVGEllipseContent }
*
*/
public SVGEllipseContent createSVGEllipseContent() {
return new SVGEllipseContent();
}
/**
* Create an instance of {@link LinearGradient }
*
*/
public LinearGradient createLinearGradient() {
return new LinearGradient();
}
/**
* Create an instance of {@link SVGMarkerClass }
*
*/
public SVGMarkerClass createSVGMarkerClass() {
return new SVGMarkerClass();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SVGMarkerClass }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "SVG.Marker.class")
public JAXBElement createSVGMarkerClass(SVGMarkerClass value) {
return new JAXBElement(_SVGMarkerClass_QNAME, SVGMarkerClass.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SVGMaskClass }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "SVG.Mask.class")
public JAXBElement createSVGMaskClass(SVGMaskClass value) {
return new JAXBElement(_SVGMaskClass_QNAME, SVGMaskClass.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SVGPatternClass }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "pattern", substitutionHeadNamespace = "http://www.w3.org/2000/svg", substitutionHeadName = "SVG.Pattern.class")
public JAXBElement createPattern(SVGPatternClass value) {
return new JAXBElement(_Pattern_QNAME, SVGPatternClass.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FeGaussianBlur }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "feGaussianBlur", substitutionHeadNamespace = "http://www.w3.org/2000/svg", substitutionHeadName = "SVG.FilterPrimitive.class")
public JAXBElement createFeGaussianBlur(FeGaussianBlur value) {
return new JAXBElement(_FeGaussianBlur_QNAME, FeGaussianBlur.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Tspan }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "tspan", substitutionHeadNamespace = "http://www.w3.org/2000/svg", substitutionHeadName = "SVG.TextContent.class")
public JAXBElement createTspan(Tspan value) {
return new JAXBElement(_Tspan_QNAME, Tspan.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2000/svg", name = "SVG.Font.class")
public JAXBElement