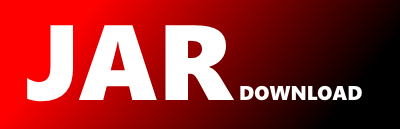
org.postgresql.adba.operations.PgParameterizedRowOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pgadba Show documentation
Show all versions of pgadba Show documentation
ADBA implementation for PostgreSQL
The newest version!
package org.postgresql.adba.operations;
import jdk.incubator.sql2.ParameterizedRowOperation;
import jdk.incubator.sql2.Result;
import jdk.incubator.sql2.SqlType;
import jdk.incubator.sql2.Submission;
import org.postgresql.adba.PgSession;
import org.postgresql.adba.operations.helpers.FutureQueryParameter;
import org.postgresql.adba.operations.helpers.ParameterHolder;
import org.postgresql.adba.operations.helpers.ValueQueryParameter;
import org.postgresql.adba.submissions.GroupSubmission;
import org.postgresql.adba.submissions.RowSubmission;
import java.time.Duration;
import java.util.concurrent.CompletionStage;
import java.util.function.Consumer;
import java.util.stream.Collector;
public class PgParameterizedRowOperation implements ParameterizedRowOperation {
private PgSession connection;
private String sql;
private ParameterHolder holder;
private Collector collector = Collector.of(() -> null, (a, v) -> {
}, (a, b) -> null, a -> null);
private Consumer errorHandler;
private GroupSubmission groupSubmission;
/**
* Creates a ParameterizedRowOperation, an operation that accepts parameters and processes a sequence of rows.
* @param connection connection that the query should be part of
* @param sql the query
* @param groupSubmission the group that this execution should be part of
*/
public PgParameterizedRowOperation(PgSession connection, String sql, GroupSubmission groupSubmission) {
this.connection = connection;
this.sql = sql;
this.holder = new ParameterHolder();
this.groupSubmission = groupSubmission;
}
@Override
public ParameterizedRowOperation onError(Consumer errorHandler) {
if (this.errorHandler != null) {
throw new IllegalStateException("you are not allowed to call onError multiple times");
}
this.errorHandler = errorHandler;
return this;
}
@Override
public ParameterizedRowOperation fetchSize(long rows) throws IllegalArgumentException {
return this;
}
@Override
public ParameterizedRowOperation collect(Collector super Result.RowColumn, A, S> c) {
collector = c;
return this;
}
@Override
public ParameterizedRowOperation set(String id, Object value) {
holder.add(id, new ValueQueryParameter(value));
return this;
}
@Override
public ParameterizedRowOperation set(String id, Object value, SqlType type) {
holder.add(id, new ValueQueryParameter(value, type));
return this;
}
@Override
public ParameterizedRowOperation set(String id, CompletionStage> source) {
holder.add(id, new FutureQueryParameter(source));
return this;
}
@Override
public ParameterizedRowOperation set(String id, CompletionStage> source, SqlType type) {
holder.add(id, new FutureQueryParameter(source, type));
return this;
}
@Override
public ParameterizedRowOperation timeout(Duration minTime) {
return this;
}
@Override
public Submission submit() {
RowSubmission submission = new RowSubmission<>(this::cancel, errorHandler, holder, groupSubmission, sql);
submission.setCollector(collector);
connection.submit(submission);
return submission;
}
private boolean cancel() {
// todo set life cycle to canceled
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy