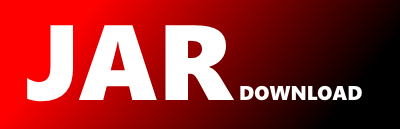
org.powertac.distributionutility.ChargeInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of distribution-utility Show documentation
Show all versions of distribution-utility Show documentation
Balances supply and demand in the current timeslot
/*
* Copyright (c) 2012 by the original author
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.powertac.distributionutility;
import java.util.ArrayList;
import java.util.List;
import org.powertac.common.Broker;
import org.powertac.common.msg.BalancingOrder;
/**
* Per-broker data holder for DU settlement processors
* @author John Collins
*/
class ChargeInfo
{
private Broker broker = null;
private double netLoadKWh = 0.0;
private double balanceChargeP1 = 0.0;
private double balanceChargeP2 = 0.0;
private List balancingOrders = null;
ChargeInfo (Broker broker, double netLoad)
{
this.broker = broker;
this.netLoadKWh = netLoad;
}
// -- getters & setters
Broker getBroker ()
{
return broker;
}
String getBrokerName ()
{
return broker.getUsername();
}
double getNetLoadKWh ()
{
return netLoadKWh;
}
double getBalanceCharge ()
{
return balanceChargeP1 + balanceChargeP2;
}
double getBalanceChargeP1()
{
return balanceChargeP1;
}
void setBalanceChargeP1 (double charge)
{
balanceChargeP1 = charge;
}
double getBalanceChargeP2()
{
return balanceChargeP2;
}
void setBalanceChargeP2 (double charge)
{
balanceChargeP2 = charge;
}
List getBalancingOrders ()
{
return balancingOrders;
}
void addBalancingOrder (BalancingOrder order)
{
if (null == balancingOrders)
balancingOrders = new ArrayList();
balancingOrders.add(order);
}
public String toString ()
{
return ("CI(" + broker.getUsername() + "): p1=" + balanceChargeP1
+ ", p2=" + balanceChargeP2);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy