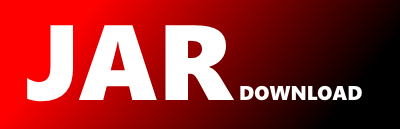
org.primefaces.extensions.component.gchart.model.DefaultGChartModel Maven / Gradle / Ivy
package org.primefaces.extensions.component.gchart.model;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import org.primefaces.extensions.util.json.GsonConverter;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
class DefaultGChartModel implements GChartModel{
private static final long serialVersionUID = -4757917806522708660L;
public DefaultGChartModel(List rows, GChartType gChartType,
Map options, List columns) {
super();
this.rows = rows;
this.gChartType = gChartType;
this.options = options;
this.columns = columns;
}
private List rows;
private GChartType gChartType;
private Map options;
private List columns;
public GChartType getgChartType() {
return gChartType;
}
public Map getOptions() {
return options;
}
public Collection getColumns() {
return columns;
}
public GChartType getChartType() {
return gChartType;
}
public Collection getRows() {
return rows;
}
public String toJson() {
JsonObject root = new JsonObject();
root.addProperty("type",this.getChartType().getChartName());
root.add("options", GsonConverter.getGson().toJsonTree(this.getOptions()));
root.add("data",extractData());
return GsonConverter.getGson().toJson(root);
}
@SuppressWarnings("unchecked")
protected JsonElement extractData() {
Collection> dataTable = new ArrayList>(0);
dataTable.add((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy