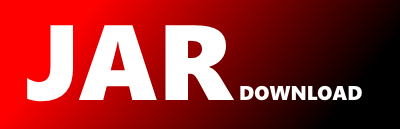
org.primefaces.model.charts.pie.PieChartDataSet Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright (c) 2009-2023 PrimeTek Informatics
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.primefaces.model.charts.pie;
import java.io.IOException;
import java.util.List;
import org.primefaces.model.charts.ChartDataSet;
import org.primefaces.util.ChartUtils;
import org.primefaces.util.FastStringWriter;
/**
* Used to provide DataSet objects to Pie chart component.
* @see https://www.chartjs.org/docs/latest/configuration/elements.html#arc-configuration
*/
public class PieChartDataSet extends ChartDataSet {
private static final long serialVersionUID = 1L;
private List data;
private List backgroundColor;
private List borderColor;
private List borderWidth;
private List hoverBackgroundColor;
private List hoverBorderColor;
private List hoverBorderWidth;
/**
* Gets the list of data in this dataSet
*
* @return List<Number> list of data
*/
public List getData() {
return data;
}
/**
* Sets the list of data in this dataSet
*
* @param data List<Number> list of data
*/
public void setData(List data) {
this.data = data;
}
/**
* Gets the backgroundColor
*
* @return backgroundColor
*/
public List getBackgroundColor() {
return backgroundColor;
}
/**
* Sets the backgroundColor
*
* @param backgroundColor The fill color of the arcs in the dataset.
*/
public void setBackgroundColor(List backgroundColor) {
this.backgroundColor = backgroundColor;
}
/**
* Gets the borderColor
*
* @return borderColor
*/
public List getBorderColor() {
return borderColor;
}
/**
* Sets the borderColor
*
* @param borderColor The border color of the arcs in the dataset.
*/
public void setBorderColor(List borderColor) {
this.borderColor = borderColor;
}
/**
* Gets the borderWidth
*
* @return borderWidth
*/
public List getBorderWidth() {
return borderWidth;
}
/**
* Sets the borderWidth
*
* @param borderWidth The border width of the arcs in the dataset.
*/
public void setBorderWidth(List borderWidth) {
this.borderWidth = borderWidth;
}
/**
* Gets the hoverBackgroundColor
*
* @return hoverBackgroundColor
*/
public List getHoverBackgroundColor() {
return hoverBackgroundColor;
}
/**
* Sets the hoverBackgroundColor
*
* @param hoverBackgroundColor The fill colour of the arcs when hovered.
*/
public void setHoverBackgroundColor(List hoverBackgroundColor) {
this.hoverBackgroundColor = hoverBackgroundColor;
}
/**
* Gets the hoverBorderColor
*
* @return hoverBorderColor
*/
public List getHoverBorderColor() {
return hoverBorderColor;
}
/**
* Sets the hoverBorderColor
*
* @param hoverBorderColor The stroke colour of the arcs when hovered.
*/
public void setHoverBorderColor(List hoverBorderColor) {
this.hoverBorderColor = hoverBorderColor;
}
/**
* Gets the hoverBorderWidth
*
* @return hoverBorderWidth
*/
public List getHoverBorderWidth() {
return hoverBorderWidth;
}
/**
* Sets the hoverBorderWidth
*
* @param hoverBorderWidth The stroke width of the arcs when hovered.
*/
public void setHoverBorderWidth(List hoverBorderWidth) {
this.hoverBorderWidth = hoverBorderWidth;
}
/**
* Gets the type
*
* @return type of current chart
*/
public String getType() {
return "pie";
}
/**
* Write the options of this dataSet
*
* @return options as JSON object
* @throws java.io.IOException If an I/O error occurs
*/
@Override
public String encode() throws IOException {
try (FastStringWriter fsw = new FastStringWriter()) {
fsw.write("{");
ChartUtils.writeDataValue(fsw, "type", this.getType(), false);
ChartUtils.writeDataValue(fsw, "data", this.data, true);
ChartUtils.writeDataValue(fsw, "hidden", this.isHidden(), true);
ChartUtils.writeDataValue(fsw, "backgroundColor", this.backgroundColor, true);
ChartUtils.writeDataValue(fsw, "borderColor", this.borderColor, true);
ChartUtils.writeDataValue(fsw, "borderWidth", this.borderWidth, true);
ChartUtils.writeDataValue(fsw, "hoverBackgroundColor", this.hoverBackgroundColor, true);
ChartUtils.writeDataValue(fsw, "hoverBorderColor", this.hoverBorderColor, true);
ChartUtils.writeDataValue(fsw, "hoverBorderWidth", this.hoverBorderWidth, true);
fsw.write("}");
return fsw.toString();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy