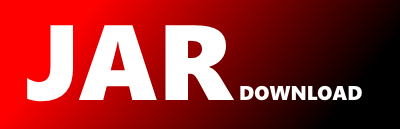
org.projecthusky.xua.pki.PkiManager Maven / Gradle / Ivy
/*
* This code is made available under the terms of the Eclipse Public License v1.0
* in the github project https://github.com/project-husky/husky there you also
* find a list of the contributors and the license information.
*
* This project has been developed further and modified by the joined working group Husky
* on the basis of the eHealth Connector opensource project from June 28, 2021,
* whereas medshare GmbH is the initial and main contributor/author of the eHealth Connector.
*
*/
package org.projecthusky.xua.pki;
import java.io.File;
import java.io.InputStream;
import java.io.OutputStream;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.cert.Certificate;
import java.util.List;
/**
*
*
* Interface describing the methods of the PkiManager.
* Interface welches die Methoden des PkiManagers beschreibt.
*
*
*
*
*/
public interface PkiManager {
/**
* The proprietary keystore implementation provided by the SunJCE provider.
*/
public static final String TYPE_JCEKS = "jceks";
/** The proprietary keystore implementation provided by the SUN provider. */
public static final String TYPE_JKS = "jks";
/**
* The transfer syntax for personal identity information as defined in PKCS
* #12.
*/
public static final String TYPE_PKCS12 = "pkcs12";
/**
*
* Method to registers a Client Key/Certificate in the {@link java.security.KeyStore}.
* Methode um ein ClientKey/Zertifikat im {@link java.security.KeyStore} zu registrieren.
*
*
* @param privateKeyPemPath
* the path of the file with the private key
* der Pfad des Ffiles mit dem privaten Schlüssel
*
*
* @param clientCertPemPath
* the path of the pem file of the certificate
* der Pfad des pem Files des Zertifikates
*
*
* @param alias
* the alias the key and cert should be referenced with
* der Alias unter dem der Schlüssel und das Zertifikat referenziert werden soll
*
*
* @param keyStore
* the keystore the client key/certificate should be addded to
* Der keystore in dem Schlüssel/Zertifikat hinzugefügt werden soll
*
*
* @param aKeyPassword
* the password of the key
* das Passwort des Keys
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
void addClientKeyAndCert(File privateKeyPemPath, File clientCertPemPath, String alias,
KeyStore keyStore, String aKeyPassword) throws KeyStoreException;
/**
*
*
* Method to add a certificate to a keystore.
* Methode um ein öffentliches Zertifikat im Key Store zu registrieren.
*
*
*
* @param publicCertPemPath
* the path of the file the certificate is stored in
* der Pfad des Files des Zertifikates
*
*
* @param alias
* the alias the cert should be referenced with
* der Alias unter dem das Zertifikat referenziert werden soll
*
*
* @param keyStore
* the keystore the certificate should be addded to
* Der Keystore dem das Zertifikat hinzugefügt werden soll
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
void addPublicCert(File publicCertPemPath, String alias, KeyStore keyStore)
throws KeyStoreException;
/**
*
* Method to create a new {@link java.security.KeyStore}. The Keystore is not saved.
* Methode um einen neuen {@link java.security.KeyStore} zu erstellen. Der Keystore wird nicht gespeichert.
*
*
* @param storeType
* the type of the key store (see {@link #TYPE_JKS}, @link #TYPE_JCEKS}, @link #TYPE_PKCS12}).
* der Typ des Keystore (siehe {@link #TYPE_JKS}, {@link #TYPE_JCEKS}, {@link #TYPE_PKCS12}).
*
*
* @return
* the {@link java.security.KeyStore} loaded from file.
* der {@link java.security.KeyStore} geladen vom File.
*
*
* @throws KeyStoreException
* will be thrown when an error occures loading the KeyStore from filesystem.
* wird geworfen wenn ein Fehler beim Laden des KeyStores aus dem Filesystem auftritt
*
*
*
*/
KeyStore createNewStore(String storeType) throws KeyStoreException;
/**
*
*
* Method to list all registered certificates.
* Methode zum Auflisten der registrierten Zertifikate.
*
*
*
* @param keyStore
* the keystore
* Der Keystore
*
*
* @return
* a {@link java.util.List} of all certificate aliases
* Eine {@link java.util.List} von allen Zertifikat Aliases
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
List listCertificateAliases(KeyStore keyStore) throws KeyStoreException;
/**
*
*
* Method to list all registered certificates.
* Methode zum Auflisten der registrierten Zerifikate.
*
*
*
* @param keyStore
* the keystore
* Der Keystore
*
*
* @return
* a {@link java.util.List} of all certificates
* Eine {@link java.util.List} von allen Zertifikaten
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
List listCertificates(KeyStore keyStore) throws KeyStoreException;
/**
*
* Method to load the {@link java.security.KeyStore} from filesystem.
* Methode welches den {@link java.security.KeyStore} vom Filesystem lädt.
*
*
* @param storeInputStream
* the InputStream of the {@link java.security.KeyStore} file.
* der InputStream des {@link java.security.KeyStore} Files.
*
*
* @param storePassword
* the password of the {@link java.security.KeyStore}
* das Passwort des {@link java.security.KeyStore}
*
*
* @param storeType
* the type of the key store (see {@link #TYPE_JKS}, @link #TYPE_JCEKS}, @link #TYPE_PKCS12}).
* der Typ des Keystore (siehe {@link #TYPE_JKS}, {@link #TYPE_JCEKS}, {@link #TYPE_PKCS12}).
*
*
* @return
* the {@link java.security.KeyStore} loaded from file.
* der {@link java.security.KeyStore} geladen vom File.
*
*
* @throws KeyStoreException
* will be thrown when an error occures loading the KeyStore
* wird geworfen wenn ein Fehler beim Laden des KeyStores auftritt
*
*
*
*/
KeyStore loadStore(InputStream storeInputStream, String storePassword, String storeType)
throws KeyStoreException;
/**
*
*
* Removes an Entry from the {@link java.security.KeyStore} referenced by an alias.
* Entfernt ein Eintrag referenziert durch den Alias aus dem {@link java.security.KeyStore}.
*
*
*
* @param alias
* the alias the key and cert should be referenced with
* der Alias unter dem der Schlüssel und das Zertifikat referenziert werden soll
*
*
* @param keyStore
* the keystore the certificate should be removed from
* Der Keystore dem das Zertifikat entfernt werden soll
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
void removeCert(String alias, KeyStore keyStore) throws KeyStoreException;
/**
*
*
* Method to store a {@link java.security.KeyStore} into a file.
* Methode zum Speichern eines {@link java.security.KeyStore} in ein File.
*
*
*
* @param keyStore
* the keystore the certificate should be addded to
* Der Keystore dem das Zertifikat hinzugefügt werden soll
*
*
* @param storeOutputStream
* the OutputStream of the file the keystore to be stored in
* der OutputStream des Files in dem der Keystore gespeichert wird
*
*
* @param storePassword
* the password of the {@link java.security.KeyStore}
* das Passwort des {@link java.security.KeyStore}
*
*
* @throws KeyStoreException
* will be thrown when an error occures storing the KeyStore to filesystem
* wird geworfen wenn ein Fehler beim Speichern des KeyStores ins Filesystem auftritt
*
*
*
*/
void storeStore(KeyStore keyStore, OutputStream storeOutputStream, String storePassword)
throws KeyStoreException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy