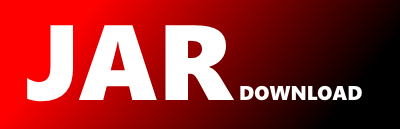
org.projectnessie.services.rest.ContentsResource Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Dremio
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.projectnessie.services.rest;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.enterprise.context.RequestScoped;
import javax.inject.Inject;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.SecurityContext;
import org.projectnessie.api.ContentsApi;
import org.projectnessie.error.NessieNotFoundException;
import org.projectnessie.model.CommitMeta;
import org.projectnessie.model.Contents;
import org.projectnessie.model.ContentsKey;
import org.projectnessie.model.ImmutableMultiGetContentsResponse;
import org.projectnessie.model.MultiGetContentsRequest;
import org.projectnessie.model.MultiGetContentsResponse;
import org.projectnessie.model.MultiGetContentsResponse.ContentsWithKey;
import org.projectnessie.services.config.ServerConfig;
import org.projectnessie.versioned.Hash;
import org.projectnessie.versioned.Key;
import org.projectnessie.versioned.ReferenceNotFoundException;
import org.projectnessie.versioned.VersionStore;
/** REST endpoint for contents. */
@RequestScoped
public class ContentsResource extends BaseResource implements ContentsApi {
@Context SecurityContext securityContext;
@Inject
public ContentsResource(
ServerConfig config,
MultiTenant multiTenant,
VersionStore store) {
super(config, multiTenant, store);
}
@Override
protected SecurityContext getSecurityContext() {
return securityContext;
}
@Override
public Contents getContents(ContentsKey key, String namedRef, String hashOnRef)
throws NessieNotFoundException {
Hash ref = namedRefWithHashOrThrow(namedRef, hashOnRef).getHash();
try {
Contents obj = getStore().getValue(ref, toKey(key));
if (obj != null) {
return obj;
}
throw new NessieNotFoundException("Requested contents do not exist for specified reference.");
} catch (ReferenceNotFoundException e) {
throw new NessieNotFoundException(
String.format("Provided reference [%s] does not exist.", namedRef), e);
}
}
@Override
public MultiGetContentsResponse getMultipleContents(
String namedRef, String hashOnRef, MultiGetContentsRequest request)
throws NessieNotFoundException {
try {
Hash ref = namedRefWithHashOrThrow(namedRef, hashOnRef).getHash();
List externalKeys = request.getRequestedKeys();
List internalKeys =
externalKeys.stream().map(ContentsResource::toKey).collect(Collectors.toList());
List> values = getStore().getValues(ref, internalKeys);
List output = new ArrayList<>();
for (int i = 0; i < externalKeys.size(); i++) {
final int pos = i;
values.get(i).ifPresent(v -> output.add(ContentsWithKey.of(externalKeys.get(pos), v)));
}
return ImmutableMultiGetContentsResponse.builder().contents(output).build();
} catch (ReferenceNotFoundException ex) {
throw new NessieNotFoundException("Unable to find the requested ref.", ex);
}
}
static Key toKey(ContentsKey key) {
return Key.of(key.getElements().toArray(new String[key.getElements().size()]));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy