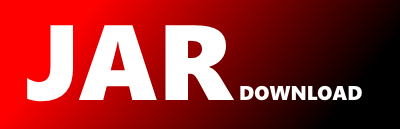
org.projectnessie.versioned.persist.adapter.ImmutableDifference Maven / Gradle / Ivy
package org.projectnessie.versioned.persist.adapter;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import com.google.protobuf.ByteString;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.projectnessie.versioned.Key;
/**
* Immutable implementation of {@link Difference}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDifference.builder()}.
*/
@Generated(from = "Difference", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableDifference implements Difference {
private final Key key;
private final @Nullable ByteString global;
private final @Nullable ByteString fromValue;
private final @Nullable ByteString toValue;
private ImmutableDifference(
Key key,
@Nullable ByteString global,
@Nullable ByteString fromValue,
@Nullable ByteString toValue) {
this.key = key;
this.global = global;
this.fromValue = fromValue;
this.toValue = toValue;
}
/**
* @return The value of the {@code key} attribute
*/
@Override
public Key getKey() {
return key;
}
/**
* @return The value of the {@code global} attribute
*/
@Override
public Optional getGlobal() {
return Optional.ofNullable(global);
}
/**
* @return The value of the {@code fromValue} attribute
*/
@Override
public Optional getFromValue() {
return Optional.ofNullable(fromValue);
}
/**
* @return The value of the {@code toValue} attribute
*/
@Override
public Optional getToValue() {
return Optional.ofNullable(toValue);
}
/**
* Copy the current immutable object by setting a value for the {@link Difference#getKey() key} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for key
* @return A modified copy of the {@code this} object
*/
public final ImmutableDifference withKey(Key value) {
if (this.key == value) return this;
Key newValue = Objects.requireNonNull(value, "key");
return new ImmutableDifference(newValue, this.global, this.fromValue, this.toValue);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Difference#getGlobal() global} attribute.
* @param value The value for global
* @return A modified copy of {@code this} object
*/
public final ImmutableDifference withGlobal(ByteString value) {
@Nullable ByteString newValue = Objects.requireNonNull(value, "global");
if (this.global == newValue) return this;
return new ImmutableDifference(this.key, newValue, this.fromValue, this.toValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Difference#getGlobal() global} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for global
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableDifference withGlobal(Optional extends ByteString> optional) {
@Nullable ByteString value = optional.orElse(null);
if (this.global == value) return this;
return new ImmutableDifference(this.key, value, this.fromValue, this.toValue);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Difference#getFromValue() fromValue} attribute.
* @param value The value for fromValue
* @return A modified copy of {@code this} object
*/
public final ImmutableDifference withFromValue(ByteString value) {
@Nullable ByteString newValue = Objects.requireNonNull(value, "fromValue");
if (this.fromValue == newValue) return this;
return new ImmutableDifference(this.key, this.global, newValue, this.toValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Difference#getFromValue() fromValue} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for fromValue
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableDifference withFromValue(Optional extends ByteString> optional) {
@Nullable ByteString value = optional.orElse(null);
if (this.fromValue == value) return this;
return new ImmutableDifference(this.key, this.global, value, this.toValue);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Difference#getToValue() toValue} attribute.
* @param value The value for toValue
* @return A modified copy of {@code this} object
*/
public final ImmutableDifference withToValue(ByteString value) {
@Nullable ByteString newValue = Objects.requireNonNull(value, "toValue");
if (this.toValue == newValue) return this;
return new ImmutableDifference(this.key, this.global, this.fromValue, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Difference#getToValue() toValue} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for toValue
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableDifference withToValue(Optional extends ByteString> optional) {
@Nullable ByteString value = optional.orElse(null);
if (this.toValue == value) return this;
return new ImmutableDifference(this.key, this.global, this.fromValue, value);
}
/**
* This instance is equal to all instances of {@code ImmutableDifference} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDifference
&& equalTo((ImmutableDifference) another);
}
private boolean equalTo(ImmutableDifference another) {
return key.equals(another.key)
&& Objects.equals(global, another.global)
&& Objects.equals(fromValue, another.fromValue)
&& Objects.equals(toValue, another.toValue);
}
/**
* Computes a hash code from attributes: {@code key}, {@code global}, {@code fromValue}, {@code toValue}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + key.hashCode();
h += (h << 5) + Objects.hashCode(global);
h += (h << 5) + Objects.hashCode(fromValue);
h += (h << 5) + Objects.hashCode(toValue);
return h;
}
/**
* Prints the immutable value {@code Difference} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Difference")
.omitNullValues()
.add("key", key)
.add("global", global)
.add("fromValue", fromValue)
.add("toValue", toValue)
.toString();
}
/**
* Creates an immutable copy of a {@link Difference} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Difference instance
*/
public static ImmutableDifference copyOf(Difference instance) {
if (instance instanceof ImmutableDifference) {
return (ImmutableDifference) instance;
}
return ImmutableDifference.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDifference ImmutableDifference}.
*
* ImmutableDifference.builder()
* .key(org.projectnessie.versioned.Key) // required {@link Difference#getKey() key}
* .global(com.google.protobuf.ByteString) // optional {@link Difference#getGlobal() global}
* .fromValue(com.google.protobuf.ByteString) // optional {@link Difference#getFromValue() fromValue}
* .toValue(com.google.protobuf.ByteString) // optional {@link Difference#getToValue() toValue}
* .build();
*
* @return A new ImmutableDifference builder
*/
public static ImmutableDifference.Builder builder() {
return new ImmutableDifference.Builder();
}
/**
* Builds instances of type {@link ImmutableDifference ImmutableDifference}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Difference", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_KEY = 0x1L;
private long initBits = 0x1L;
private @Nullable Key key;
private @Nullable ByteString global;
private @Nullable ByteString fromValue;
private @Nullable ByteString toValue;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Difference} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Difference instance) {
Objects.requireNonNull(instance, "instance");
key(instance.getKey());
Optional globalOptional = instance.getGlobal();
if (globalOptional.isPresent()) {
global(globalOptional);
}
Optional fromValueOptional = instance.getFromValue();
if (fromValueOptional.isPresent()) {
fromValue(fromValueOptional);
}
Optional toValueOptional = instance.getToValue();
if (toValueOptional.isPresent()) {
toValue(toValueOptional);
}
return this;
}
/**
* Initializes the value for the {@link Difference#getKey() key} attribute.
* @param key The value for key
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder key(Key key) {
this.key = Objects.requireNonNull(key, "key");
initBits &= ~INIT_BIT_KEY;
return this;
}
/**
* Initializes the optional value {@link Difference#getGlobal() global} to global.
* @param global The value for global
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder global(ByteString global) {
this.global = Objects.requireNonNull(global, "global");
return this;
}
/**
* Initializes the optional value {@link Difference#getGlobal() global} to global.
* @param global The value for global
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder global(Optional extends ByteString> global) {
this.global = global.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Difference#getFromValue() fromValue} to fromValue.
* @param fromValue The value for fromValue
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder fromValue(ByteString fromValue) {
this.fromValue = Objects.requireNonNull(fromValue, "fromValue");
return this;
}
/**
* Initializes the optional value {@link Difference#getFromValue() fromValue} to fromValue.
* @param fromValue The value for fromValue
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder fromValue(Optional extends ByteString> fromValue) {
this.fromValue = fromValue.orElse(null);
return this;
}
/**
* Initializes the optional value {@link Difference#getToValue() toValue} to toValue.
* @param toValue The value for toValue
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder toValue(ByteString toValue) {
this.toValue = Objects.requireNonNull(toValue, "toValue");
return this;
}
/**
* Initializes the optional value {@link Difference#getToValue() toValue} to toValue.
* @param toValue The value for toValue
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toValue(Optional extends ByteString> toValue) {
this.toValue = toValue.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableDifference ImmutableDifference}.
* @return An immutable instance of Difference
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDifference build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDifference(key, global, fromValue, toValue);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_KEY) != 0) attributes.add("key");
return "Cannot build Difference, some of required attributes are not set " + attributes;
}
}
}