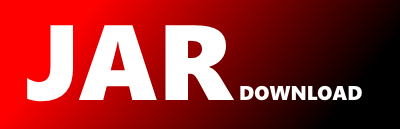
org.projectnessie.versioned.persist.adapter.ImmutableContentAndState Maven / Gradle / Ivy
package org.projectnessie.versioned.persist.adapter;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ContentAndState}.
*
* Use the builder to create immutable instances:
* {@code ImmutableContentAndState.builder()}.
*/
@Generated(from = "ContentAndState", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableContentAndState
implements ContentAndState {
private final CONTENT refState;
private final @Nullable CONTENT globalState;
private ImmutableContentAndState(CONTENT refState, @Nullable CONTENT globalState) {
this.refState = refState;
this.globalState = globalState;
}
/**
* Per-named-reference state for a content key. For example, Iceberg's snapshot-ID, schema-ID,
* partition-spec-ID, default-sort-order-ID.
*/
@Override
public CONTENT getRefState() {
return refState;
}
/**
*Global state for a content key. For example, the pointer to Iceberg's table-metadata.
*/
@Override
public @Nullable CONTENT getGlobalState() {
return globalState;
}
/**
* Copy the current immutable object by setting a value for the {@link ContentAndState#getRefState() refState} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for refState
* @return A modified copy of the {@code this} object
*/
public final ImmutableContentAndState withRefState(CONTENT value) {
if (this.refState == value) return this;
CONTENT newValue = Objects.requireNonNull(value, "refState");
return new ImmutableContentAndState<>(newValue, this.globalState);
}
/**
* Copy the current immutable object by setting a value for the {@link ContentAndState#getGlobalState() globalState} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for globalState (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContentAndState withGlobalState(@Nullable CONTENT value) {
if (this.globalState == value) return this;
return new ImmutableContentAndState<>(this.refState, value);
}
/**
* This instance is equal to all instances of {@code ImmutableContentAndState} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableContentAndState>
&& equalTo(0, (ImmutableContentAndState>) another);
}
private boolean equalTo(int synthetic, ImmutableContentAndState> another) {
return refState.equals(another.refState)
&& Objects.equals(globalState, another.globalState);
}
/**
* Computes a hash code from attributes: {@code refState}, {@code globalState}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + refState.hashCode();
h += (h << 5) + Objects.hashCode(globalState);
return h;
}
/**
* Prints the immutable value {@code ContentAndState} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ContentAndState")
.omitNullValues()
.add("refState", refState)
.add("globalState", globalState)
.toString();
}
/**
* Creates an immutable copy of a {@link ContentAndState} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter CONTENT
* @param instance The instance to copy
* @return A copied immutable ContentAndState instance
*/
public static ImmutableContentAndState copyOf(ContentAndState instance) {
if (instance instanceof ImmutableContentAndState>) {
return (ImmutableContentAndState) instance;
}
return ImmutableContentAndState.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableContentAndState ImmutableContentAndState}.
*
* ImmutableContentAndState.<CONTENT>builder()
* .refState(CONTENT) // required {@link ContentAndState#getRefState() refState}
* .globalState(CONTENT | null) // nullable {@link ContentAndState#getGlobalState() globalState}
* .build();
*
* @param generic parameter CONTENT
* @return A new ImmutableContentAndState builder
*/
public static ImmutableContentAndState.Builder builder() {
return new ImmutableContentAndState.Builder<>();
}
/**
* Builds instances of type {@link ImmutableContentAndState ImmutableContentAndState}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ContentAndState", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_REF_STATE = 0x1L;
private long initBits = 0x1L;
private @Nullable CONTENT refState;
private @Nullable CONTENT globalState;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ContentAndState} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ContentAndState instance) {
Objects.requireNonNull(instance, "instance");
refState(instance.getRefState());
@Nullable CONTENT globalStateValue = instance.getGlobalState();
if (globalStateValue != null) {
globalState(globalStateValue);
}
return this;
}
/**
* Initializes the value for the {@link ContentAndState#getRefState() refState} attribute.
* @param refState The value for refState
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder refState(CONTENT refState) {
this.refState = Objects.requireNonNull(refState, "refState");
initBits &= ~INIT_BIT_REF_STATE;
return this;
}
/**
* Initializes the value for the {@link ContentAndState#getGlobalState() globalState} attribute.
* @param globalState The value for globalState (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder globalState(@Nullable CONTENT globalState) {
this.globalState = globalState;
return this;
}
/**
* Builds a new {@link ImmutableContentAndState ImmutableContentAndState}.
* @return An immutable instance of ContentAndState
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableContentAndState build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableContentAndState<>(refState, globalState);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_REF_STATE) != 0) attributes.add("refState");
return "Cannot build ContentAndState, some of required attributes are not set " + attributes;
}
}
}