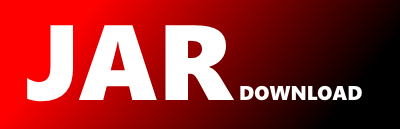
org.projectnessie.versioned.persist.adapter.ImmutableCommitLogEntry Maven / Gradle / Ivy
package org.projectnessie.versioned.persist.adapter;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.primitives.Longs;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import com.google.protobuf.ByteString;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.projectnessie.versioned.Hash;
import org.projectnessie.versioned.Key;
/**
* Immutable implementation of {@link CommitLogEntry}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCommitLogEntry.builder()}.
*/
@Generated(from = "CommitLogEntry", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableCommitLogEntry
implements CommitLogEntry {
private final long createdTime;
private final Hash hash;
private final long commitSeq;
private final ImmutableList parents;
private final ByteString metadata;
private final ImmutableList puts;
private final ImmutableList deletes;
private final @Nullable KeyList keyList;
private final ImmutableList keyListsIds;
private final int keyListDistance;
private ImmutableCommitLogEntry(
long createdTime,
Hash hash,
long commitSeq,
ImmutableList parents,
ByteString metadata,
ImmutableList puts,
ImmutableList deletes,
@Nullable KeyList keyList,
ImmutableList keyListsIds,
int keyListDistance) {
this.createdTime = createdTime;
this.hash = hash;
this.commitSeq = commitSeq;
this.parents = parents;
this.metadata = metadata;
this.puts = puts;
this.deletes = deletes;
this.keyList = keyList;
this.keyListsIds = keyListsIds;
this.keyListDistance = keyListDistance;
}
/**
*Creation timestamp in microseconds since epoch.
*/
@Override
public long getCreatedTime() {
return createdTime;
}
/**
* @return The value of the {@code hash} attribute
*/
@Override
public Hash getHash() {
return hash;
}
/**
* Monotonically increasing counter representing the number of commits since the "beginning of
* time.
*/
@Override
public long getCommitSeq() {
return commitSeq;
}
/**
*Zero, one or more parent-entry hashes of this commit, nearest parent first.
*/
@Override
public ImmutableList getParents() {
return parents;
}
/**
*Serialized commit-metadata.
*/
@Override
public ByteString getMetadata() {
return metadata;
}
/**
* List of all {@code Put} operations, with their keys, content-types and serialized {@code
* Content}.
*/
@Override
public ImmutableList getPuts() {
return puts;
}
/**
*List of "unchanged" keys, from {@code Delete} commit operations.
*/
@Override
public ImmutableList getDeletes() {
return deletes;
}
/**
* The list of all "reachable" or "known" {@link org.projectnessie.versioned.Key}s up to this
* commit-log-entry's parent commit consists of all entries in this {@link
* org.projectnessie.versioned.persist.adapter.KeyList} plus the {@link
* org.projectnessie.versioned.persist.adapter.KeyListEntity}s via {@link #getKeyListsIds()}.
* This key-list checkpoint, if present, does not contain the key-changes in this
* entry in {@link #getPuts()} and {@link #getDeletes()}.
*/
@Override
public @Nullable KeyList getKeyList() {
return keyList;
}
/**
* IDs of for the linked {@link org.projectnessie.versioned.persist.adapter.KeyListEntity} that,
* together with {@link #getKeyList()} make the complete {@link org.projectnessie.versioned.Key}
* for this commit.
*/
@Override
public ImmutableList getKeyListsIds() {
return keyListsIds;
}
/**
*Number of commits since the last complete key-list.
*/
@Override
public int getKeyListDistance() {
return keyListDistance;
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getCreatedTime() createdTime} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for createdTime
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withCreatedTime(long value) {
if (this.createdTime == value) return this;
return new ImmutableCommitLogEntry(
value,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getHash() hash} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hash
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withHash(Hash value) {
if (this.hash == value) return this;
Hash newValue = Objects.requireNonNull(value, "hash");
return new ImmutableCommitLogEntry(
this.createdTime,
newValue,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getCommitSeq() commitSeq} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for commitSeq
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withCommitSeq(long value) {
if (this.commitSeq == value) return this;
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
value,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getParents() parents}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withParents(Hash... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
newValue,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getParents() parents}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of parents elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withParents(Iterable extends Hash> elements) {
if (this.parents == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
newValue,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getMetadata() metadata} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for metadata
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withMetadata(ByteString value) {
if (this.metadata == value) return this;
ByteString newValue = Objects.requireNonNull(value, "metadata");
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
newValue,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getPuts() puts}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withPuts(KeyWithBytes... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
newValue,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getPuts() puts}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of puts elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withPuts(Iterable extends KeyWithBytes> elements) {
if (this.puts == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
newValue,
this.deletes,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getDeletes() deletes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withDeletes(Key... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
newValue,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getDeletes() deletes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of deletes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withDeletes(Iterable extends Key> elements) {
if (this.deletes == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
newValue,
this.keyList,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getKeyList() keyList} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for keyList (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withKeyList(@Nullable KeyList value) {
if (this.keyList == value) return this;
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
value,
this.keyListsIds,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getKeyListsIds() keyListsIds}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withKeyListsIds(Hash... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
newValue,
this.keyListDistance);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CommitLogEntry#getKeyListsIds() keyListsIds}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of keyListsIds elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCommitLogEntry withKeyListsIds(Iterable extends Hash> elements) {
if (this.keyListsIds == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
newValue,
this.keyListDistance);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitLogEntry#getKeyListDistance() keyListDistance} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for keyListDistance
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitLogEntry withKeyListDistance(int value) {
if (this.keyListDistance == value) return this;
return new ImmutableCommitLogEntry(
this.createdTime,
this.hash,
this.commitSeq,
this.parents,
this.metadata,
this.puts,
this.deletes,
this.keyList,
this.keyListsIds,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableCommitLogEntry} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableCommitLogEntry
&& equalTo(0, (ImmutableCommitLogEntry) another);
}
private boolean equalTo(int synthetic, ImmutableCommitLogEntry another) {
return createdTime == another.createdTime
&& hash.equals(another.hash)
&& commitSeq == another.commitSeq
&& parents.equals(another.parents)
&& metadata.equals(another.metadata)
&& puts.equals(another.puts)
&& deletes.equals(another.deletes)
&& Objects.equals(keyList, another.keyList)
&& keyListsIds.equals(another.keyListsIds)
&& keyListDistance == another.keyListDistance;
}
/**
* Computes a hash code from attributes: {@code createdTime}, {@code hash}, {@code commitSeq}, {@code parents}, {@code metadata}, {@code puts}, {@code deletes}, {@code keyList}, {@code keyListsIds}, {@code keyListDistance}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Longs.hashCode(createdTime);
h += (h << 5) + hash.hashCode();
h += (h << 5) + Longs.hashCode(commitSeq);
h += (h << 5) + parents.hashCode();
h += (h << 5) + metadata.hashCode();
h += (h << 5) + puts.hashCode();
h += (h << 5) + deletes.hashCode();
h += (h << 5) + Objects.hashCode(keyList);
h += (h << 5) + keyListsIds.hashCode();
h += (h << 5) + keyListDistance;
return h;
}
/**
* Prints the immutable value {@code CommitLogEntry} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("CommitLogEntry")
.omitNullValues()
.add("createdTime", createdTime)
.add("hash", hash)
.add("commitSeq", commitSeq)
.add("parents", parents)
.add("metadata", metadata)
.add("puts", puts)
.add("deletes", deletes)
.add("keyList", keyList)
.add("keyListsIds", keyListsIds)
.add("keyListDistance", keyListDistance)
.toString();
}
/**
* Creates an immutable copy of a {@link CommitLogEntry} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CommitLogEntry instance
*/
public static ImmutableCommitLogEntry copyOf(CommitLogEntry instance) {
if (instance instanceof ImmutableCommitLogEntry) {
return (ImmutableCommitLogEntry) instance;
}
return ImmutableCommitLogEntry.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCommitLogEntry ImmutableCommitLogEntry}.
*
* ImmutableCommitLogEntry.builder()
* .createdTime(long) // required {@link CommitLogEntry#getCreatedTime() createdTime}
* .hash(org.projectnessie.versioned.Hash) // required {@link CommitLogEntry#getHash() hash}
* .commitSeq(long) // required {@link CommitLogEntry#getCommitSeq() commitSeq}
* .addParents|addAllParents(org.projectnessie.versioned.Hash) // {@link CommitLogEntry#getParents() parents} elements
* .metadata(com.google.protobuf.ByteString) // required {@link CommitLogEntry#getMetadata() metadata}
* .addPuts|addAllPuts(org.projectnessie.versioned.persist.adapter.KeyWithBytes) // {@link CommitLogEntry#getPuts() puts} elements
* .addDeletes|addAllDeletes(org.projectnessie.versioned.Key) // {@link CommitLogEntry#getDeletes() deletes} elements
* .keyList(org.projectnessie.versioned.persist.adapter.KeyList | null) // nullable {@link CommitLogEntry#getKeyList() keyList}
* .addKeyListsIds|addAllKeyListsIds(org.projectnessie.versioned.Hash) // {@link CommitLogEntry#getKeyListsIds() keyListsIds} elements
* .keyListDistance(int) // required {@link CommitLogEntry#getKeyListDistance() keyListDistance}
* .build();
*
* @return A new ImmutableCommitLogEntry builder
*/
public static ImmutableCommitLogEntry.Builder builder() {
return new ImmutableCommitLogEntry.Builder();
}
/**
* Builds instances of type {@link ImmutableCommitLogEntry ImmutableCommitLogEntry}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CommitLogEntry", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_CREATED_TIME = 0x1L;
private static final long INIT_BIT_HASH = 0x2L;
private static final long INIT_BIT_COMMIT_SEQ = 0x4L;
private static final long INIT_BIT_METADATA = 0x8L;
private static final long INIT_BIT_KEY_LIST_DISTANCE = 0x10L;
private long initBits = 0x1fL;
private long createdTime;
private @Nullable Hash hash;
private long commitSeq;
private ImmutableList.Builder parents = ImmutableList.builder();
private @Nullable ByteString metadata;
private ImmutableList.Builder puts = ImmutableList.builder();
private ImmutableList.Builder deletes = ImmutableList.builder();
private @Nullable KeyList keyList;
private ImmutableList.Builder keyListsIds = ImmutableList.builder();
private int keyListDistance;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CommitLogEntry} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(CommitLogEntry instance) {
Objects.requireNonNull(instance, "instance");
createdTime(instance.getCreatedTime());
hash(instance.getHash());
commitSeq(instance.getCommitSeq());
addAllParents(instance.getParents());
metadata(instance.getMetadata());
addAllPuts(instance.getPuts());
addAllDeletes(instance.getDeletes());
@Nullable KeyList keyListValue = instance.getKeyList();
if (keyListValue != null) {
keyList(keyListValue);
}
addAllKeyListsIds(instance.getKeyListsIds());
keyListDistance(instance.getKeyListDistance());
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getCreatedTime() createdTime} attribute.
* @param createdTime The value for createdTime
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder createdTime(long createdTime) {
this.createdTime = createdTime;
initBits &= ~INIT_BIT_CREATED_TIME;
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getHash() hash} attribute.
* @param hash The value for hash
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder hash(Hash hash) {
this.hash = Objects.requireNonNull(hash, "hash");
initBits &= ~INIT_BIT_HASH;
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getCommitSeq() commitSeq} attribute.
* @param commitSeq The value for commitSeq
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder commitSeq(long commitSeq) {
this.commitSeq = commitSeq;
initBits &= ~INIT_BIT_COMMIT_SEQ;
return this;
}
/**
* Adds one element to {@link CommitLogEntry#getParents() parents} list.
* @param element A parents element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParents(Hash element) {
this.parents.add(element);
return this;
}
/**
* Adds elements to {@link CommitLogEntry#getParents() parents} list.
* @param elements An array of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParents(Hash... elements) {
this.parents.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link CommitLogEntry#getParents() parents} list.
* @param elements An iterable of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder parents(Iterable extends Hash> elements) {
this.parents = ImmutableList.builder();
return addAllParents(elements);
}
/**
* Adds elements to {@link CommitLogEntry#getParents() parents} list.
* @param elements An iterable of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllParents(Iterable extends Hash> elements) {
this.parents.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getMetadata() metadata} attribute.
* @param metadata The value for metadata
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder metadata(ByteString metadata) {
this.metadata = Objects.requireNonNull(metadata, "metadata");
initBits &= ~INIT_BIT_METADATA;
return this;
}
/**
* Adds one element to {@link CommitLogEntry#getPuts() puts} list.
* @param element A puts element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addPuts(KeyWithBytes element) {
this.puts.add(element);
return this;
}
/**
* Adds elements to {@link CommitLogEntry#getPuts() puts} list.
* @param elements An array of puts elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addPuts(KeyWithBytes... elements) {
this.puts.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link CommitLogEntry#getPuts() puts} list.
* @param elements An iterable of puts elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder puts(Iterable extends KeyWithBytes> elements) {
this.puts = ImmutableList.builder();
return addAllPuts(elements);
}
/**
* Adds elements to {@link CommitLogEntry#getPuts() puts} list.
* @param elements An iterable of puts elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllPuts(Iterable extends KeyWithBytes> elements) {
this.puts.addAll(elements);
return this;
}
/**
* Adds one element to {@link CommitLogEntry#getDeletes() deletes} list.
* @param element A deletes element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addDeletes(Key element) {
this.deletes.add(element);
return this;
}
/**
* Adds elements to {@link CommitLogEntry#getDeletes() deletes} list.
* @param elements An array of deletes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addDeletes(Key... elements) {
this.deletes.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link CommitLogEntry#getDeletes() deletes} list.
* @param elements An iterable of deletes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder deletes(Iterable extends Key> elements) {
this.deletes = ImmutableList.builder();
return addAllDeletes(elements);
}
/**
* Adds elements to {@link CommitLogEntry#getDeletes() deletes} list.
* @param elements An iterable of deletes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllDeletes(Iterable extends Key> elements) {
this.deletes.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getKeyList() keyList} attribute.
* @param keyList The value for keyList (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder keyList(@Nullable KeyList keyList) {
this.keyList = keyList;
return this;
}
/**
* Adds one element to {@link CommitLogEntry#getKeyListsIds() keyListsIds} list.
* @param element A keyListsIds element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addKeyListsIds(Hash element) {
this.keyListsIds.add(element);
return this;
}
/**
* Adds elements to {@link CommitLogEntry#getKeyListsIds() keyListsIds} list.
* @param elements An array of keyListsIds elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addKeyListsIds(Hash... elements) {
this.keyListsIds.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link CommitLogEntry#getKeyListsIds() keyListsIds} list.
* @param elements An iterable of keyListsIds elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder keyListsIds(Iterable extends Hash> elements) {
this.keyListsIds = ImmutableList.builder();
return addAllKeyListsIds(elements);
}
/**
* Adds elements to {@link CommitLogEntry#getKeyListsIds() keyListsIds} list.
* @param elements An iterable of keyListsIds elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllKeyListsIds(Iterable extends Hash> elements) {
this.keyListsIds.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link CommitLogEntry#getKeyListDistance() keyListDistance} attribute.
* @param keyListDistance The value for keyListDistance
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder keyListDistance(int keyListDistance) {
this.keyListDistance = keyListDistance;
initBits &= ~INIT_BIT_KEY_LIST_DISTANCE;
return this;
}
/**
* Builds a new {@link ImmutableCommitLogEntry ImmutableCommitLogEntry}.
* @return An immutable instance of CommitLogEntry
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCommitLogEntry build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableCommitLogEntry(
createdTime,
hash,
commitSeq,
parents.build(),
metadata,
puts.build(),
deletes.build(),
keyList,
keyListsIds.build(),
keyListDistance);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_CREATED_TIME) != 0) attributes.add("createdTime");
if ((initBits & INIT_BIT_HASH) != 0) attributes.add("hash");
if ((initBits & INIT_BIT_COMMIT_SEQ) != 0) attributes.add("commitSeq");
if ((initBits & INIT_BIT_METADATA) != 0) attributes.add("metadata");
if ((initBits & INIT_BIT_KEY_LIST_DISTANCE) != 0) attributes.add("keyListDistance");
return "Cannot build CommitLogEntry, some of required attributes are not set " + attributes;
}
}
}