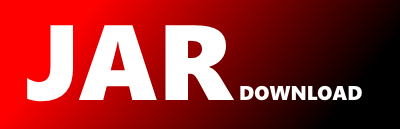
org.projectnessie.versioned.persist.adapter.ImmutableGlobalLogCompactionParams Maven / Gradle / Ivy
package org.projectnessie.versioned.persist.adapter;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.Booleans;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GlobalLogCompactionParams}.
*
* Use the builder to create immutable instances:
* {@code ImmutableGlobalLogCompactionParams.builder()}.
*/
@Generated(from = "GlobalLogCompactionParams", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableGlobalLogCompactionParams
implements GlobalLogCompactionParams {
private final boolean isEnabled;
private final int noCompactionWhenCompactedWithin;
private final int noCompactionUpToLength;
private ImmutableGlobalLogCompactionParams(ImmutableGlobalLogCompactionParams.Builder builder) {
if (builder.isEnabledIsSet()) {
initShim.isEnabled(builder.isEnabled);
}
if (builder.noCompactionWhenCompactedWithinIsSet()) {
initShim.noCompactionWhenCompactedWithin(builder.noCompactionWhenCompactedWithin);
}
if (builder.noCompactionUpToLengthIsSet()) {
initShim.noCompactionUpToLength(builder.noCompactionUpToLength);
}
this.isEnabled = initShim.isEnabled();
this.noCompactionWhenCompactedWithin = initShim.getNoCompactionWhenCompactedWithin();
this.noCompactionUpToLength = initShim.getNoCompactionUpToLength();
this.initShim = null;
}
private ImmutableGlobalLogCompactionParams(boolean isEnabled, int noCompactionWhenCompactedWithin, int noCompactionUpToLength) {
this.isEnabled = isEnabled;
this.noCompactionWhenCompactedWithin = noCompactionWhenCompactedWithin;
this.noCompactionUpToLength = noCompactionUpToLength;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "GlobalLogCompactionParams", generator = "Immutables")
private final class InitShim {
private byte isEnabledBuildStage = STAGE_UNINITIALIZED;
private boolean isEnabled;
boolean isEnabled() {
if (isEnabledBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isEnabledBuildStage == STAGE_UNINITIALIZED) {
isEnabledBuildStage = STAGE_INITIALIZING;
this.isEnabled = isEnabledInitialize();
isEnabledBuildStage = STAGE_INITIALIZED;
}
return this.isEnabled;
}
void isEnabled(boolean isEnabled) {
this.isEnabled = isEnabled;
isEnabledBuildStage = STAGE_INITIALIZED;
}
private byte noCompactionWhenCompactedWithinBuildStage = STAGE_UNINITIALIZED;
private int noCompactionWhenCompactedWithin;
int getNoCompactionWhenCompactedWithin() {
if (noCompactionWhenCompactedWithinBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (noCompactionWhenCompactedWithinBuildStage == STAGE_UNINITIALIZED) {
noCompactionWhenCompactedWithinBuildStage = STAGE_INITIALIZING;
this.noCompactionWhenCompactedWithin = getNoCompactionWhenCompactedWithinInitialize();
noCompactionWhenCompactedWithinBuildStage = STAGE_INITIALIZED;
}
return this.noCompactionWhenCompactedWithin;
}
void noCompactionWhenCompactedWithin(int noCompactionWhenCompactedWithin) {
this.noCompactionWhenCompactedWithin = noCompactionWhenCompactedWithin;
noCompactionWhenCompactedWithinBuildStage = STAGE_INITIALIZED;
}
private byte noCompactionUpToLengthBuildStage = STAGE_UNINITIALIZED;
private int noCompactionUpToLength;
int getNoCompactionUpToLength() {
if (noCompactionUpToLengthBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (noCompactionUpToLengthBuildStage == STAGE_UNINITIALIZED) {
noCompactionUpToLengthBuildStage = STAGE_INITIALIZING;
this.noCompactionUpToLength = getNoCompactionUpToLengthInitialize();
noCompactionUpToLengthBuildStage = STAGE_INITIALIZED;
}
return this.noCompactionUpToLength;
}
void noCompactionUpToLength(int noCompactionUpToLength) {
this.noCompactionUpToLength = noCompactionUpToLength;
noCompactionUpToLengthBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (isEnabledBuildStage == STAGE_INITIALIZING) attributes.add("isEnabled");
if (noCompactionWhenCompactedWithinBuildStage == STAGE_INITIALIZING) attributes.add("noCompactionWhenCompactedWithin");
if (noCompactionUpToLengthBuildStage == STAGE_INITIALIZING) attributes.add("noCompactionUpToLength");
return "Cannot build GlobalLogCompactionParams, attribute initializers form cycle " + attributes;
}
}
private boolean isEnabledInitialize() {
return GlobalLogCompactionParams.super.isEnabled();
}
private int getNoCompactionWhenCompactedWithinInitialize() {
return GlobalLogCompactionParams.super.getNoCompactionWhenCompactedWithin();
}
private int getNoCompactionUpToLengthInitialize() {
return GlobalLogCompactionParams.super.getNoCompactionUpToLength();
}
/**
* @return The value of the {@code isEnabled} attribute
*/
@Override
public boolean isEnabled() {
InitShim shim = this.initShim;
return shim != null
? shim.isEnabled()
: this.isEnabled;
}
/**
* When the global-log contains a compacted entry within this number of entries, global-log
* compaction will not happen, defaults to {@value #DEFAULT_NO_COMPACTION_WHEN_COMPACTED_WITHIN}.
*/
@Override
public int getNoCompactionWhenCompactedWithin() {
InitShim shim = this.initShim;
return shim != null
? shim.getNoCompactionWhenCompactedWithin()
: this.noCompactionWhenCompactedWithin;
}
/**
* When the global-log contains only up to this number of entries, global-log compaction will not
* happen, defaults to {@value #DEFAULT_NO_COMPACTION_UP_TO_LENGTH}.
*/
@Override
public int getNoCompactionUpToLength() {
InitShim shim = this.initShim;
return shim != null
? shim.getNoCompactionUpToLength()
: this.noCompactionUpToLength;
}
/**
* Copy the current immutable object by setting a value for the {@link GlobalLogCompactionParams#isEnabled() isEnabled} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isEnabled
* @return A modified copy of the {@code this} object
*/
public final ImmutableGlobalLogCompactionParams withIsEnabled(boolean value) {
if (this.isEnabled == value) return this;
return new ImmutableGlobalLogCompactionParams(value, this.noCompactionWhenCompactedWithin, this.noCompactionUpToLength);
}
/**
* Copy the current immutable object by setting a value for the {@link GlobalLogCompactionParams#getNoCompactionWhenCompactedWithin() noCompactionWhenCompactedWithin} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for noCompactionWhenCompactedWithin
* @return A modified copy of the {@code this} object
*/
public final ImmutableGlobalLogCompactionParams withNoCompactionWhenCompactedWithin(int value) {
if (this.noCompactionWhenCompactedWithin == value) return this;
return new ImmutableGlobalLogCompactionParams(this.isEnabled, value, this.noCompactionUpToLength);
}
/**
* Copy the current immutable object by setting a value for the {@link GlobalLogCompactionParams#getNoCompactionUpToLength() noCompactionUpToLength} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for noCompactionUpToLength
* @return A modified copy of the {@code this} object
*/
public final ImmutableGlobalLogCompactionParams withNoCompactionUpToLength(int value) {
if (this.noCompactionUpToLength == value) return this;
return new ImmutableGlobalLogCompactionParams(this.isEnabled, this.noCompactionWhenCompactedWithin, value);
}
/**
* This instance is equal to all instances of {@code ImmutableGlobalLogCompactionParams} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableGlobalLogCompactionParams
&& equalTo(0, (ImmutableGlobalLogCompactionParams) another);
}
private boolean equalTo(int synthetic, ImmutableGlobalLogCompactionParams another) {
return isEnabled == another.isEnabled
&& noCompactionWhenCompactedWithin == another.noCompactionWhenCompactedWithin
&& noCompactionUpToLength == another.noCompactionUpToLength;
}
/**
* Computes a hash code from attributes: {@code isEnabled}, {@code noCompactionWhenCompactedWithin}, {@code noCompactionUpToLength}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Booleans.hashCode(isEnabled);
h += (h << 5) + noCompactionWhenCompactedWithin;
h += (h << 5) + noCompactionUpToLength;
return h;
}
/**
* Prints the immutable value {@code GlobalLogCompactionParams} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("GlobalLogCompactionParams")
.omitNullValues()
.add("isEnabled", isEnabled)
.add("noCompactionWhenCompactedWithin", noCompactionWhenCompactedWithin)
.add("noCompactionUpToLength", noCompactionUpToLength)
.toString();
}
/**
* Creates an immutable copy of a {@link GlobalLogCompactionParams} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GlobalLogCompactionParams instance
*/
public static ImmutableGlobalLogCompactionParams copyOf(GlobalLogCompactionParams instance) {
if (instance instanceof ImmutableGlobalLogCompactionParams) {
return (ImmutableGlobalLogCompactionParams) instance;
}
return ImmutableGlobalLogCompactionParams.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableGlobalLogCompactionParams ImmutableGlobalLogCompactionParams}.
*
* ImmutableGlobalLogCompactionParams.builder()
* .isEnabled(boolean) // optional {@link GlobalLogCompactionParams#isEnabled() isEnabled}
* .noCompactionWhenCompactedWithin(int) // optional {@link GlobalLogCompactionParams#getNoCompactionWhenCompactedWithin() noCompactionWhenCompactedWithin}
* .noCompactionUpToLength(int) // optional {@link GlobalLogCompactionParams#getNoCompactionUpToLength() noCompactionUpToLength}
* .build();
*
* @return A new ImmutableGlobalLogCompactionParams builder
*/
public static ImmutableGlobalLogCompactionParams.Builder builder() {
return new ImmutableGlobalLogCompactionParams.Builder();
}
/**
* Builds instances of type {@link ImmutableGlobalLogCompactionParams ImmutableGlobalLogCompactionParams}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GlobalLogCompactionParams", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long OPT_BIT_IS_ENABLED = 0x1L;
private static final long OPT_BIT_NO_COMPACTION_WHEN_COMPACTED_WITHIN = 0x2L;
private static final long OPT_BIT_NO_COMPACTION_UP_TO_LENGTH = 0x4L;
private long optBits;
private boolean isEnabled;
private int noCompactionWhenCompactedWithin;
private int noCompactionUpToLength;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code GlobalLogCompactionParams} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(GlobalLogCompactionParams instance) {
Objects.requireNonNull(instance, "instance");
isEnabled(instance.isEnabled());
noCompactionWhenCompactedWithin(instance.getNoCompactionWhenCompactedWithin());
noCompactionUpToLength(instance.getNoCompactionUpToLength());
return this;
}
/**
* Initializes the value for the {@link GlobalLogCompactionParams#isEnabled() isEnabled} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GlobalLogCompactionParams#isEnabled() isEnabled}.
* @param isEnabled The value for isEnabled
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder isEnabled(boolean isEnabled) {
this.isEnabled = isEnabled;
optBits |= OPT_BIT_IS_ENABLED;
return this;
}
/**
* Initializes the value for the {@link GlobalLogCompactionParams#getNoCompactionWhenCompactedWithin() noCompactionWhenCompactedWithin} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GlobalLogCompactionParams#getNoCompactionWhenCompactedWithin() noCompactionWhenCompactedWithin}.
* @param noCompactionWhenCompactedWithin The value for noCompactionWhenCompactedWithin
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder noCompactionWhenCompactedWithin(int noCompactionWhenCompactedWithin) {
this.noCompactionWhenCompactedWithin = noCompactionWhenCompactedWithin;
optBits |= OPT_BIT_NO_COMPACTION_WHEN_COMPACTED_WITHIN;
return this;
}
/**
* Initializes the value for the {@link GlobalLogCompactionParams#getNoCompactionUpToLength() noCompactionUpToLength} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GlobalLogCompactionParams#getNoCompactionUpToLength() noCompactionUpToLength}.
* @param noCompactionUpToLength The value for noCompactionUpToLength
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder noCompactionUpToLength(int noCompactionUpToLength) {
this.noCompactionUpToLength = noCompactionUpToLength;
optBits |= OPT_BIT_NO_COMPACTION_UP_TO_LENGTH;
return this;
}
/**
* Builds a new {@link ImmutableGlobalLogCompactionParams ImmutableGlobalLogCompactionParams}.
* @return An immutable instance of GlobalLogCompactionParams
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableGlobalLogCompactionParams build() {
return new ImmutableGlobalLogCompactionParams(this);
}
private boolean isEnabledIsSet() {
return (optBits & OPT_BIT_IS_ENABLED) != 0;
}
private boolean noCompactionWhenCompactedWithinIsSet() {
return (optBits & OPT_BIT_NO_COMPACTION_WHEN_COMPACTED_WITHIN) != 0;
}
private boolean noCompactionUpToLengthIsSet() {
return (optBits & OPT_BIT_NO_COMPACTION_UP_TO_LENGTH) != 0;
}
}
}