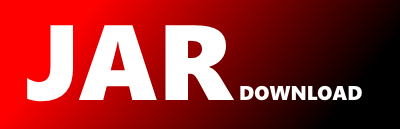
org.projectnessie.versioned.persist.adapter.ImmutableRefLog Maven / Gradle / Ivy
package org.projectnessie.versioned.persist.adapter;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.primitives.Longs;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
import org.projectnessie.versioned.Hash;
/**
* Immutable implementation of {@link RefLog}.
*
* Use the builder to create immutable instances:
* {@code ImmutableRefLog.builder()}.
*/
@Generated(from = "RefLog", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableRefLog implements RefLog {
private final Hash refLogId;
private final String refName;
private final String refType;
private final Hash commitHash;
private final ImmutableList parents;
private final long operationTime;
private final String operation;
private final ImmutableList sourceHashes;
private ImmutableRefLog(
Hash refLogId,
String refName,
String refType,
Hash commitHash,
ImmutableList parents,
long operationTime,
String operation,
ImmutableList sourceHashes) {
this.refLogId = refLogId;
this.refName = refName;
this.refType = refType;
this.commitHash = commitHash;
this.parents = parents;
this.operationTime = operationTime;
this.operation = operation;
this.sourceHashes = sourceHashes;
}
/**
*Reflog id of the current entry.
*/
@Override
public Hash getRefLogId() {
return refLogId;
}
/**
*Reference on which current operation is executed.
*/
@Override
public String getRefName() {
return refName;
}
/**
*Reference type can be 'Branch' or 'Tag'.
*/
@Override
public String getRefType() {
return refType;
}
/**
*Output commit hash of the operation.
*/
@Override
public Hash getCommitHash() {
return commitHash;
}
/**
*Parent reflog id of the current entry.
*/
@Override
public ImmutableList getParents() {
return parents;
}
/**
*Time in microseconds since epoch.
*/
@Override
public long getOperationTime() {
return operationTime;
}
/**
*Operation String mapped to ENUM in {@code RefLogEntry.Operation} of 'persist.proto' file.
*/
@Override
public String getOperation() {
return operation;
}
/**
* Single hash in case of MERGE or ASSIGN. One or more hashes in case of TRANSPLANT. Empty list
* for other operations.
*/
@Override
public ImmutableList getSourceHashes() {
return sourceHashes;
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getRefLogId() refLogId} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for refLogId
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withRefLogId(Hash value) {
if (this.refLogId == value) return this;
Hash newValue = Objects.requireNonNull(value, "refLogId");
return new ImmutableRefLog(
newValue,
this.refName,
this.refType,
this.commitHash,
this.parents,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getRefName() refName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for refName
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withRefName(String value) {
String newValue = Objects.requireNonNull(value, "refName");
if (this.refName.equals(newValue)) return this;
return new ImmutableRefLog(
this.refLogId,
newValue,
this.refType,
this.commitHash,
this.parents,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getRefType() refType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for refType
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withRefType(String value) {
String newValue = Objects.requireNonNull(value, "refType");
if (this.refType.equals(newValue)) return this;
return new ImmutableRefLog(
this.refLogId,
this.refName,
newValue,
this.commitHash,
this.parents,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getCommitHash() commitHash} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for commitHash
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withCommitHash(Hash value) {
if (this.commitHash == value) return this;
Hash newValue = Objects.requireNonNull(value, "commitHash");
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
newValue,
this.parents,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object with elements that replace the content of {@link RefLog#getParents() parents}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRefLog withParents(Hash... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
newValue,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object with elements that replace the content of {@link RefLog#getParents() parents}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of parents elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRefLog withParents(Iterable extends Hash> elements) {
if (this.parents == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
newValue,
this.operationTime,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getOperationTime() operationTime} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for operationTime
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withOperationTime(long value) {
if (this.operationTime == value) return this;
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
this.parents,
value,
this.operation,
this.sourceHashes);
}
/**
* Copy the current immutable object by setting a value for the {@link RefLog#getOperation() operation} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for operation
* @return A modified copy of the {@code this} object
*/
public final ImmutableRefLog withOperation(String value) {
String newValue = Objects.requireNonNull(value, "operation");
if (this.operation.equals(newValue)) return this;
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
this.parents,
this.operationTime,
newValue,
this.sourceHashes);
}
/**
* Copy the current immutable object with elements that replace the content of {@link RefLog#getSourceHashes() sourceHashes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRefLog withSourceHashes(Hash... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
this.parents,
this.operationTime,
this.operation,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link RefLog#getSourceHashes() sourceHashes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of sourceHashes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableRefLog withSourceHashes(Iterable extends Hash> elements) {
if (this.sourceHashes == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableRefLog(
this.refLogId,
this.refName,
this.refType,
this.commitHash,
this.parents,
this.operationTime,
this.operation,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableRefLog} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableRefLog
&& equalTo(0, (ImmutableRefLog) another);
}
private boolean equalTo(int synthetic, ImmutableRefLog another) {
return refLogId.equals(another.refLogId)
&& refName.equals(another.refName)
&& refType.equals(another.refType)
&& commitHash.equals(another.commitHash)
&& parents.equals(another.parents)
&& operationTime == another.operationTime
&& operation.equals(another.operation)
&& sourceHashes.equals(another.sourceHashes);
}
/**
* Computes a hash code from attributes: {@code refLogId}, {@code refName}, {@code refType}, {@code commitHash}, {@code parents}, {@code operationTime}, {@code operation}, {@code sourceHashes}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + refLogId.hashCode();
h += (h << 5) + refName.hashCode();
h += (h << 5) + refType.hashCode();
h += (h << 5) + commitHash.hashCode();
h += (h << 5) + parents.hashCode();
h += (h << 5) + Longs.hashCode(operationTime);
h += (h << 5) + operation.hashCode();
h += (h << 5) + sourceHashes.hashCode();
return h;
}
/**
* Prints the immutable value {@code RefLog} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("RefLog")
.omitNullValues()
.add("refLogId", refLogId)
.add("refName", refName)
.add("refType", refType)
.add("commitHash", commitHash)
.add("parents", parents)
.add("operationTime", operationTime)
.add("operation", operation)
.add("sourceHashes", sourceHashes)
.toString();
}
/**
* Creates an immutable copy of a {@link RefLog} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable RefLog instance
*/
public static ImmutableRefLog copyOf(RefLog instance) {
if (instance instanceof ImmutableRefLog) {
return (ImmutableRefLog) instance;
}
return ImmutableRefLog.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableRefLog ImmutableRefLog}.
*
* ImmutableRefLog.builder()
* .refLogId(org.projectnessie.versioned.Hash) // required {@link RefLog#getRefLogId() refLogId}
* .refName(String) // required {@link RefLog#getRefName() refName}
* .refType(String) // required {@link RefLog#getRefType() refType}
* .commitHash(org.projectnessie.versioned.Hash) // required {@link RefLog#getCommitHash() commitHash}
* .addParents|addAllParents(org.projectnessie.versioned.Hash) // {@link RefLog#getParents() parents} elements
* .operationTime(long) // required {@link RefLog#getOperationTime() operationTime}
* .operation(String) // required {@link RefLog#getOperation() operation}
* .addSourceHashes|addAllSourceHashes(org.projectnessie.versioned.Hash) // {@link RefLog#getSourceHashes() sourceHashes} elements
* .build();
*
* @return A new ImmutableRefLog builder
*/
public static ImmutableRefLog.Builder builder() {
return new ImmutableRefLog.Builder();
}
/**
* Builds instances of type {@link ImmutableRefLog ImmutableRefLog}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "RefLog", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_REF_LOG_ID = 0x1L;
private static final long INIT_BIT_REF_NAME = 0x2L;
private static final long INIT_BIT_REF_TYPE = 0x4L;
private static final long INIT_BIT_COMMIT_HASH = 0x8L;
private static final long INIT_BIT_OPERATION_TIME = 0x10L;
private static final long INIT_BIT_OPERATION = 0x20L;
private long initBits = 0x3fL;
private @Nullable Hash refLogId;
private @Nullable String refName;
private @Nullable String refType;
private @Nullable Hash commitHash;
private ImmutableList.Builder parents = ImmutableList.builder();
private long operationTime;
private @Nullable String operation;
private ImmutableList.Builder sourceHashes = ImmutableList.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code RefLog} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(RefLog instance) {
Objects.requireNonNull(instance, "instance");
refLogId(instance.getRefLogId());
refName(instance.getRefName());
refType(instance.getRefType());
commitHash(instance.getCommitHash());
addAllParents(instance.getParents());
operationTime(instance.getOperationTime());
operation(instance.getOperation());
addAllSourceHashes(instance.getSourceHashes());
return this;
}
/**
* Initializes the value for the {@link RefLog#getRefLogId() refLogId} attribute.
* @param refLogId The value for refLogId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder refLogId(Hash refLogId) {
this.refLogId = Objects.requireNonNull(refLogId, "refLogId");
initBits &= ~INIT_BIT_REF_LOG_ID;
return this;
}
/**
* Initializes the value for the {@link RefLog#getRefName() refName} attribute.
* @param refName The value for refName
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder refName(String refName) {
this.refName = Objects.requireNonNull(refName, "refName");
initBits &= ~INIT_BIT_REF_NAME;
return this;
}
/**
* Initializes the value for the {@link RefLog#getRefType() refType} attribute.
* @param refType The value for refType
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder refType(String refType) {
this.refType = Objects.requireNonNull(refType, "refType");
initBits &= ~INIT_BIT_REF_TYPE;
return this;
}
/**
* Initializes the value for the {@link RefLog#getCommitHash() commitHash} attribute.
* @param commitHash The value for commitHash
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder commitHash(Hash commitHash) {
this.commitHash = Objects.requireNonNull(commitHash, "commitHash");
initBits &= ~INIT_BIT_COMMIT_HASH;
return this;
}
/**
* Adds one element to {@link RefLog#getParents() parents} list.
* @param element A parents element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParents(Hash element) {
this.parents.add(element);
return this;
}
/**
* Adds elements to {@link RefLog#getParents() parents} list.
* @param elements An array of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addParents(Hash... elements) {
this.parents.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link RefLog#getParents() parents} list.
* @param elements An iterable of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder parents(Iterable extends Hash> elements) {
this.parents = ImmutableList.builder();
return addAllParents(elements);
}
/**
* Adds elements to {@link RefLog#getParents() parents} list.
* @param elements An iterable of parents elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllParents(Iterable extends Hash> elements) {
this.parents.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link RefLog#getOperationTime() operationTime} attribute.
* @param operationTime The value for operationTime
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder operationTime(long operationTime) {
this.operationTime = operationTime;
initBits &= ~INIT_BIT_OPERATION_TIME;
return this;
}
/**
* Initializes the value for the {@link RefLog#getOperation() operation} attribute.
* @param operation The value for operation
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder operation(String operation) {
this.operation = Objects.requireNonNull(operation, "operation");
initBits &= ~INIT_BIT_OPERATION;
return this;
}
/**
* Adds one element to {@link RefLog#getSourceHashes() sourceHashes} list.
* @param element A sourceHashes element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSourceHashes(Hash element) {
this.sourceHashes.add(element);
return this;
}
/**
* Adds elements to {@link RefLog#getSourceHashes() sourceHashes} list.
* @param elements An array of sourceHashes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSourceHashes(Hash... elements) {
this.sourceHashes.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link RefLog#getSourceHashes() sourceHashes} list.
* @param elements An iterable of sourceHashes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder sourceHashes(Iterable extends Hash> elements) {
this.sourceHashes = ImmutableList.builder();
return addAllSourceHashes(elements);
}
/**
* Adds elements to {@link RefLog#getSourceHashes() sourceHashes} list.
* @param elements An iterable of sourceHashes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllSourceHashes(Iterable extends Hash> elements) {
this.sourceHashes.addAll(elements);
return this;
}
/**
* Builds a new {@link ImmutableRefLog ImmutableRefLog}.
* @return An immutable instance of RefLog
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableRefLog build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableRefLog(
refLogId,
refName,
refType,
commitHash,
parents.build(),
operationTime,
operation,
sourceHashes.build());
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_REF_LOG_ID) != 0) attributes.add("refLogId");
if ((initBits & INIT_BIT_REF_NAME) != 0) attributes.add("refName");
if ((initBits & INIT_BIT_REF_TYPE) != 0) attributes.add("refType");
if ((initBits & INIT_BIT_COMMIT_HASH) != 0) attributes.add("commitHash");
if ((initBits & INIT_BIT_OPERATION_TIME) != 0) attributes.add("operationTime");
if ((initBits & INIT_BIT_OPERATION) != 0) attributes.add("operation");
return "Cannot build RefLog, some of required attributes are not set " + attributes;
}
}
}