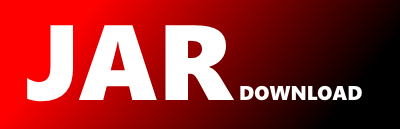
org.projectnessie.versioned.persist.adapter.CommitLogEntry Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Dremio
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.projectnessie.versioned.persist.adapter;
import com.google.protobuf.ByteString;
import java.util.List;
import javax.annotation.Nullable;
import org.immutables.value.Value;
import org.projectnessie.versioned.Hash;
import org.projectnessie.versioned.Key;
/** Represents a commit-log-entry stored in the database. */
@Value.Immutable
public interface CommitLogEntry {
/** Creation timestamp in microseconds since epoch. */
long getCreatedTime();
Hash getHash();
/**
* Monotonically increasing counter representing the number of commits since the "beginning of
* time.
*/
long getCommitSeq();
/** Zero, one or more parent-entry hashes of this commit, nearest parent first. */
List getParents();
/** Serialized commit-metadata. */
ByteString getMetadata();
/**
* List of all {@code Put} operations, with their keys, content-types and serialized {@code
* Content}.
*/
List getPuts();
/** List of "unchanged" keys, from {@code Delete} commit operations. */
List getDeletes();
/**
* The list of all "reachable" or "known" {@link org.projectnessie.versioned.Key}s up to this
* commit-log-entry's parent commit consists of all entries in this {@link
* org.projectnessie.versioned.persist.adapter.KeyList} plus the {@link
* org.projectnessie.versioned.persist.adapter.KeyListEntity}s via {@link #getKeyListsIds()}.
*
* This key-list checkpoint, if present, does not contain the key-changes in this
* entry in {@link #getPuts()} and {@link #getDeletes()}.
*/
@Nullable
KeyList getKeyList();
/**
* IDs of for the linked {@link org.projectnessie.versioned.persist.adapter.KeyListEntity} that,
* together with {@link #getKeyList()} make the complete {@link org.projectnessie.versioned.Key}
* for this commit.
*/
List getKeyListsIds();
/** The indices of the first occupied bucket in each non-embedded segment of the key list. */
@Nullable
List getKeyListEntityOffsets();
/**
* Load factor for key lists hashed using open-addressing.
*
* This is a user-configured value, typically on {@code (0, 1)}. In practice, key lists may be
* hashed at a lower effective load factor than configured here (e.g. for alignment), but
* generally not a higher one.
*/
@Nullable
Float getKeyListLoadFactor();
/**
* The cumulative total of buckets across all segments.
*
*
This is generally much greater than the segment count. Addressable buckets have indices on
* the interval {@code [0, this value)}.
*/
@Nullable
Integer getKeyListBucketCount();
/** Number of commits since the last complete key-list. */
int getKeyListDistance();
List getAdditionalParents();
@Value.Default
default KeyListVariant getKeyListVariant() {
return KeyListVariant.EMBEDDED_AND_EXTERNAL_MRU;
}
default boolean hasKeySummary() {
return getKeyListVariant() != KeyListVariant.EMBEDDED_AND_EXTERNAL_MRU || getKeyList() != null;
}
static CommitLogEntry of(
long createdTime,
Hash hash,
long commitSeq,
Iterable parents,
ByteString metadata,
Iterable puts,
Iterable deletes,
int keyListDistance,
KeyList keyList,
Iterable keyListIds,
Iterable keyListEntityOffsets,
Iterable additionalParents) {
ImmutableCommitLogEntry.Builder c =
ImmutableCommitLogEntry.builder()
.createdTime(createdTime)
.hash(hash)
.commitSeq(commitSeq)
.parents(parents)
.metadata(metadata)
.puts(puts)
.deletes(deletes)
.keyListDistance(keyListDistance)
.keyList(keyList)
.addAllKeyListsIds(keyListIds)
.addAllAdditionalParents(additionalParents);
if (keyListEntityOffsets != null) {
c.addAllKeyListEntityOffsets(keyListEntityOffsets);
}
return c.build();
}
enum KeyListVariant {
/**
* The variant in which Nessie versions 0.10.0..0.30.0 write {@link CommitLogEntry#getKeyList()
* embedded key-list} and {@link KeyListEntity key-list entities}.
*
* Most recently updated {@link KeyListEntry}s appear first.
*
*
The {@link CommitLogEntry#getKeyList() embedded key-list} is filled first up to {@link
* DatabaseAdapterConfig#getMaxKeyListSize()}, more {@link KeyListEntry}s are written to
* "external"{@link KeyListEntity key-list entities} with their IDs in {@link
* CommitLogEntry#getKeyListsIds()}.
*/
EMBEDDED_AND_EXTERNAL_MRU,
/**
* The variant in which Nessie versions since 0.31.0 maintains {@link
* CommitLogEntry#getKeyList() embedded key-list} and {@link KeyListEntity key-list entities}.
*
*
{@link KeyListEntry}s are maintained as an open-addressing hash map with {@link
* org.projectnessie.versioned.Key} as the map key.
*
*
That open-addressing hash map is split into multiple segments, if necessary.
*
*
The first segment is represented by the {@link CommitLogEntry#getKeyList() embedded
* key-list} with a serialized size goal up to {@link
* DatabaseAdapterConfig#getMaxKeyListSize()}. All following segments have a serialized size up
* to {@link DatabaseAdapterConfig#getMaxKeyListEntitySize()} as the goal.
*
*
Maximum size constraints are fulfilled using a best-effort approach.
*/
OPEN_ADDRESSING
}
}