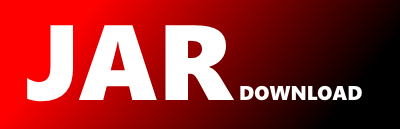
prompto.config.IRuntimeConfiguration Maven / Gradle / Ivy
The newest version!
package prompto.config;
import java.net.URL;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.function.Supplier;
import com.esotericsoftware.yamlbeans.YamlException;
import com.esotericsoftware.yamlbeans.document.YamlMapping;
import com.esotericsoftware.yamlbeans.document.YamlSequence;
import prompto.intrinsic.PromptoVersion;
import prompto.runtime.Mode;
public interface IRuntimeConfiguration {
Supplier> getRuntimeLibs();
IStoreConfiguration getCodeStoreConfiguration();
IStoreConfiguration getDataStoreConfiguration();
IDebugConfiguration getDebugConfiguration();
Mode getRuntimeMode();
Map getArguments();
String getApplicationName();
PromptoVersion getApplicationVersion();
URL[] getAddOnURLs();
URL[] getResourceURLs();
boolean isLoadRuntime();
YamlMapping toYaml() throws YamlException;
T withRuntimeLibs(Supplier> supplier);
T withCodeStoreConfiguration(IStoreConfiguration supplier);
T withDataStoreConfiguration(IStoreConfiguration supplier);
T withAddOnURLs(URL[] addOnURLS);
T withApplicationName(String name);
T withApplicationVersion(PromptoVersion version);
T withResourceURLs(URL[] resourceURLs);
T withRuntimeMode(Mode mode);
T withLoadRuntime(boolean set);
T withDebugConfiguration(IDebugConfiguration config);
@SuppressWarnings("unchecked")
public static class Inline implements IRuntimeConfiguration {
Supplier> runtimeLibs = null; // always passed from code
Supplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy