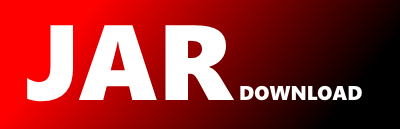
prompto.grammar.ArgumentList Maven / Gradle / Ivy
The newest version!
package prompto.grammar;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import prompto.declaration.IMethodDeclaration;
import prompto.error.SyntaxError;
import prompto.expression.AndExpression;
import prompto.expression.IExpression;
import prompto.expression.UnresolvedIdentifier;
import prompto.param.AttributeParameter;
import prompto.param.IParameter;
import prompto.runtime.Context;
import prompto.transpiler.ITranspilable;
import prompto.transpiler.Transpiler;
import prompto.utils.CodeWriter;
import prompto.value.ContextualExpression;
public class ArgumentList extends LinkedList {
private static final long serialVersionUID = 1L;
public ArgumentList() {
}
public ArgumentList(Collection arguments) {
super(arguments);
}
/* post-fix expression priority for final argument in E dialect */
/* 'xyz with a and b as c' should read 'xyz with a, b as c' NOT 'xyz with (a and b) as c' */
public void checkLastAnd() {
Argument argument = this.getLast();
if(argument!=null && argument.getParameter()!=null && argument.getExpression() instanceof AndExpression) {
AndExpression and = (AndExpression)argument.getExpression();
if(and.getLeft() instanceof UnresolvedIdentifier) {
Identifier id = ((UnresolvedIdentifier)and.getLeft()).getId();
if(Character.isLowerCase(id.toString().charAt(0))) {
this.removeLast();
// add AttributeArgument
AttributeParameter parameter = new AttributeParameter(id);
Argument attribute = new Argument(parameter, null);
this.add(attribute);
// fix last argument
argument.setExpression(and.getRight());
this.add(argument);
}
}
}
}
public int findIndex(Identifier name) {
for(int i=0;i0 && this.get(0).getParameter()==null) {
writer.append(' ');
this.get(idx++).toDialect(writer);
}
if(idx0)
writer.trimLast(2);
writer.append(")");
}
private void toMDialect(CodeWriter writer) {
toODialect(writer);
}
public void declare(Transpiler transpiler, IMethodDeclaration declaration) {
this.forEach(arg -> arg.declare(transpiler, declaration));
}
public void ensureDeclarationOrder(Context context, List list, Set set) {
this.forEach(arg -> arg.ensureDeclarationOrder(context, list, set));
}
public ArgumentList makeArguments(Context context, IMethodDeclaration declaration) {
ArgumentList original = new ArgumentList(this);
ArgumentList resolved = new ArgumentList();
for(int i=0;i0 && this.get(0).getParameter()==null)
index = 0;
if(index>=0) {
argument = original.get(index);
original.remove(index);
}
if(argument==null) {
if (parameter.getDefaultExpression() != null)
resolved.add(new Argument(parameter, parameter.getDefaultExpression()));
else
throw new SyntaxError("Missing argument:" + parameter.getName());
} else {
IExpression expression = new ContextualExpression(context, argument.getExpression());
Argument copy = new Argument(parameter, expression);
copy.setSectionFrom(argument);
resolved.add(copy);
}
}
if(original.size() > 0) {
Argument argument = original.get(0);
IParameter parameter = argument.getParameter();
IExpression expression = argument.getExpression();
String name = parameter==null ? (expression==null ? "" : expression.toString()) : parameter.getName();
throw new SyntaxError("Method has no argument:" + name);
}
return resolved;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy