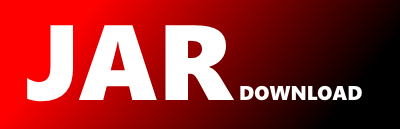
prompto.intrinsic.IterableWithCounts Maven / Gradle / Ivy
The newest version!
package prompto.intrinsic;
import java.util.Iterator;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.StreamSupport;
public interface IterableWithCounts extends Iterable {
Long getCount();
default long getNativeCount() {
return getCount();
}
Long getTotalCount();
default Iterable filter(Predicate predicate) {
Iterator items = this.iterator();
return new Iterable() {
@Override
public Iterator iterator() {
return new Iterator() {
T current;
@Override
public boolean hasNext() {
current = null;
while(items.hasNext()) {
current = items.next();
if(predicate.test(current))
return true;
}
current = null;
return false;
}
@Override
public T next() {
return current;
}
};
}
@Override
public String toString() {
return StreamSupport.stream(this.spliterator(), false)
.map(Object::toString)
.collect(Collectors.toList())
.toString();
}
};
}
default PromptoList toList() {
PromptoList result = new PromptoList(false);
for(T item : this)
result.add(item);
return result;
}
default PromptoSet toSet() {
PromptoSet result = new PromptoSet();
for(T item : this)
result.add(item);
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy