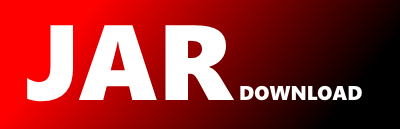
prompto.intrinsic.PromptoAny Maven / Gradle / Ivy
The newest version!
package prompto.intrinsic;
@SuppressWarnings("unchecked")
public class PromptoAny {
public static void setMember(Object instance, Object key, Object value) {
if(instance instanceof PromptoDocument)
((PromptoDocument
© 2015 - 2024 Weber Informatics LLC | Privacy Policy