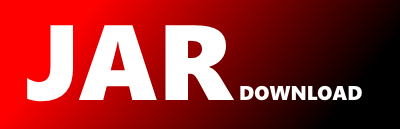
prompto.intrinsic.PromptoDict Maven / Gradle / Ivy
The newest version!
package prompto.intrinsic;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Set;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.JsonNode;
@SuppressWarnings("serial")
public class PromptoDict extends HashMap implements Iterable>, IJsonNodeProducer {
boolean mutable;
public PromptoDict(boolean mutable) {
this.mutable = mutable;
}
public boolean isMutable() {
return mutable;
}
public void setMutable(boolean mutable) {
this.mutable = mutable;
}
public PromptoDict swap() {
PromptoDict swapped = new PromptoDict<>(true);
for (HashMap.Entry kvp : entrySet()) {
String key = String.valueOf(kvp.getValue());
String value = String.valueOf(kvp.getKey());
swapped.put(key, value);
}
swapped.setMutable(false);
return swapped;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("<");
for (HashMap.Entry kvp : entrySet()) {
String key = kvp.getKey().toString();
if(!key.startsWith("\""))
sb.append('"');
sb.append(key);
if(!key.endsWith("\""))
sb.append('"');
sb.append(":");
sb.append(kvp.getValue().toString());
sb.append(", ");
}
if (sb.length() > 2)
sb.setLength(sb.length() - 2);
else
sb.append(":");
sb.append(">");
return sb.toString();
}
@Override
public V get(Object key) {
if(key==null)
throw new NullPointerException();
return super.get(key);
}
@Override
public V put(K key, V value) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
if(key==null)
throw new NullPointerException();
return super.put(key, value);
}
public Long getCount() {
return (long)size();
}
public long getNativeCount() {
return size();
}
public boolean contains(Object item) {
return containsKey(item);
}
public boolean containsAll(Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy