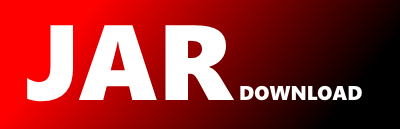
prompto.intrinsic.PromptoList Maven / Gradle / Ivy
The newest version!
package prompto.intrinsic;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.EnumSet;
import java.util.List;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import prompto.value.IMultiplyable;
@SuppressWarnings("serial")
public class PromptoList extends ArrayList implements Filterable, V>, IMultiplyable, IDocumentValueProducer, IJsonNodeProducer {
public static Collector, PromptoList> collector() {
return new Collector, PromptoList>() {
@Override
public Supplier> supplier() {
return () -> new PromptoList(false);
}
@Override
public BiConsumer, T> accumulator() {
return PromptoList::add;
}
@Override
public BinaryOperator> combiner() {
return (l1, l2) -> { l1.addAll(l2); return l1; };
}
@Override
public Function, PromptoList> finisher() {
return Function.identity();
}
@Override
public Set characteristics() {
return Collections.unmodifiableSet(EnumSet.of(Collector.Characteristics.IDENTITY_FINISH));
}
};
}
boolean mutable;
public PromptoList(boolean mutable) {
this.mutable = mutable;
}
public PromptoList(V[] items) {
super(Arrays.asList(items));
this.mutable = false;
}
public PromptoList(Collection extends V> items, boolean mutable) {
super(items);
this.mutable = mutable;
}
public boolean isMutable() {
return mutable;
}
public Long getCount() {
return (long)size();
}
public long getNativeCount() {
return size();
}
public V getLast() {
return get(this.size()-1);
}
public PromptoList multiply(int count) {
PromptoList result = new PromptoList<>(false);
while(count-->0)
result.addAll(this);
return result;
}
public PromptoList slice(long first) {
return slice(first, this.size());
}
public PromptoList slice(long first, long last) {
if (first < 0)
first = this.size() + 1 + first;
if (last < 0)
last = this.size() + 1 + last;
return new PromptoList<>(this.subList((int)(first-1), (int)last), false);
}
public void removeItem(Long item) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
this.remove(item.intValue() - 1);
}
public void removeValue(V value) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
this.remove(value);
}
public void setValue(long index, V value) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
this.set((int)index, value);
}
public void addValue(V value) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
this.add(value);
}
public void insertValue(V value, long atIndex) {
if(!mutable)
PromptoException.throwEnumeratedException("NOT_MUTABLE");
this.add((int)atIndex - 1, value);
}
public Long indexOfValue(V value) {
int idx = this.indexOf(value);
if(idx < 0)
return null;
else
return Long.valueOf(idx + 1);
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public PromptoList sort(boolean descending) {
PromptoList sorted = new PromptoList<>(this, false);
Comparator cmp = descending ?
(o1, o2) -> ((Comparable)o2).compareTo(o1) :
(o1, o2) -> ((Comparable)o1).compareTo(o2);
sorted.sort(cmp);
return sorted;
}
@SuppressWarnings("unchecked")
public PromptoList extends V> sortUsing(Comparator extends V> cmp) {
PromptoList extends V> sorted = new PromptoList<>(this, false);
sorted.sort((Comparator)cmp);
return sorted;
}
public boolean containsAny(Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy