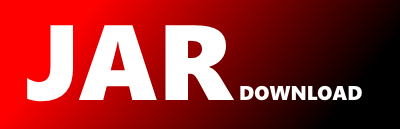
prompto.utils.IValueIterable Maven / Gradle / Ivy
The newest version!
package prompto.utils;
import java.util.Collection;
import java.util.Iterator;
import prompto.expression.IExpression;
import prompto.intrinsic.IterableWithCounts;
import prompto.runtime.Context;
import prompto.value.IValue;
public class IValueIterable implements IterableWithCounts {
Context context;
Collection iterable;
public IValueIterable(Context context, Collection iterable) {
this.context = context;
this.iterable = iterable;
}
@Override
public Long getCount() {
return (long)iterable.size();
}
@Override
public Long getTotalCount() {
return (long)iterable.size();
}
@Override
public Iterator iterator() {
return new Iterator() {
Iterator iterator = iterable.iterator();
@Override
public boolean hasNext() {
return iterator.hasNext();
}
@Override
public IValue next() {
try {
Object value = iterator.next();
if (value instanceof IExpression)
value = ((IExpression) value).interpret(context);
if (value instanceof IValue)
return (IValue) value;
else
throw new InternalError("Item not a value!");
} catch (Throwable t) {
throw new InternalError(t.getMessage());
}
}
@Override
public void remove() {
throw new InternalError("Should never get there");
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy