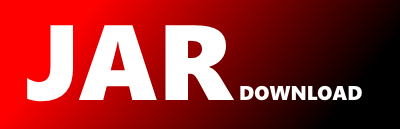
prompto.reader.XMLReader Maven / Gradle / Ivy
package prompto.reader;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.IntStream;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import prompto.intrinsic.PromptoDocument;
public class XMLReader {
public static PromptoDocument read(String xml, boolean keepNamespaces, boolean keepAttributes) throws IOException {
return new XMLReader(keepNamespaces, keepAttributes).read(xml);
}
boolean keepNamespaces;
boolean keepAttributes;
public XMLReader(boolean keepNamespaces, boolean keepAttributes) {
this.keepNamespaces = keepNamespaces;
this.keepAttributes = keepAttributes;
}
private PromptoDocument read(String xml) throws IOException {
try (InputStream input = new ByteArrayInputStream(xml.getBytes())){
DocumentBuilderFactory factory = DocumentBuilderFactory.newDefaultInstance();
factory.setNamespaceAware(keepAttributes);
factory.setIgnoringComments(true);
factory.setIgnoringElementContentWhitespace(true);
Document doc = factory.newDocumentBuilder().parse(input);
return (PromptoDocument)convertDocument(doc);
} catch(Exception e) {
throw new IOException(e.getMessage());
}
}
private PromptoDocument convertDocument(Document doc) {
PromptoDocument result = new PromptoDocument<>();
convertElement(result, doc.getDocumentElement());
return result;
}
private void convertElement(PromptoDocument parent, Element element) {
var tagName = element.getTagName();
// cater for repeated elements
if(parent.containsKey(tagName))
convertListElement(parent, tagName, element);
else
parent.put(tagName, convertElementValue(element));
}
@SuppressWarnings("unchecked")
private void convertListElement(PromptoDocument parent, String tagName, Element element) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy