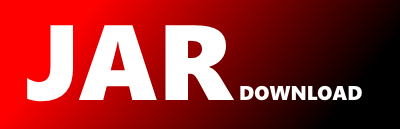
prompto.prompto.pec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of WebSite Show documentation
Show all versions of WebSite Show documentation
Prompto Documentation Web Site POM
define Callback as abstract method
define AnyCallback as abstract method receiving Any value
define BooleanCallback as abstract method receiving Boolean value
define IntegerCallback as abstract method receiving Integer value
define DecimalCallback as abstract method receiving Decimal value
define TextCallback as abstract method receiving Text value
define DateCallback as abstract method receiving Decimal value
define TimeCallback as abstract method receiving Time value
define DateTimeCallback as abstract method receiving DateTime value
define PeriodCallback as abstract method receiving Period value
define UuidCallback as abstract method receiving Uuid value
define DocumentCallback as abstract method receiving Document value
define Any as native category with attributes id and text, and bindings:
define category bindings as:
Java: prompto.intrinsic.PromptoAny
C#: prompto.value.AnyValue
Python2: AnyValue from module: prompto.value.AnyValue
Python3: AnyValue from module: prompto.value.AnyValue
JavaScript: AnyValue from module: prompto/intrinsic/AnyValue.js
and methods:
define id as getter doing:
Java: return System.identityHashCode(this);
C#: return System.Runtime.CompilerServices.RuntimeHelpers.GetHashCode(this);
Python2: return id(self)
Python3: return id(self)
JavaScript: return this.id;
define text as getter doing:
Java: return this.toString();
C#: return this.ToString();
Python2: return str(self)
Python3: return str(self)
JavaScript: return this.toString();
define Attribute as native category with attribute name, and bindings:
define category bindings as:
Java: prompto.declaration.AttributeDeclaration
C#: prompto.declaration.AttributeDeclaration
Python2: AttributeDeclaration from module: prompto.declaration.AttributeDeclaration
Python3: AttributeDeclaration from module: prompto.declaration.AttributeDeclaration
JavaScript: AttributeDeclaration from module: prompto/declaration/AttributeDeclaration.js
define findAttribute as native method receiving name returning Attribute doing:
Java: return $context.findAttribute(name);
C#: return $context.findAttribute(name);
Python2: return $context.findAttribute(name)
Python3: return $context.findAttribute(name)
JavaScript: return $context.findAttribute(name);
define getAllAttributes as native method returning Attribute[] doing:
Java: return $context.getAllAttributes();
C#: return $context.getAllAttributes();
Python2: return $context.getAllAttributes()
Python3: return $context.getAllAttributes()
JavaScript: return $context.getAllAttributes();
define "findAttribute id" as test method doing:
a = findAttribute "id"
and verifying:
a is an Attribute
a.name = "id"
define "findAttribute name" as test method doing:
a = findAttribute "name"
and verifying:
a is an Attribute
a.name = "name"
define "findAttribute text" as test method doing:
a = findAttribute "text"
and verifying:
a is an Attribute
a.name = "text"
define "at least 3 items getAllAttributes" as test method doing:
l = getAllAttributes
and verifying:
l is an Attribute[]
l.count >= 3
// runtime reference of any object
define id as Integer attribute
// database reference of stored object
define dbId as storable DbId attribute
// any object has a text attribute, used for display
define text as storable Text attribute with value and words index
// many objects have a name, it is not unique
define name as storable Text attribute with key and value index
// many objects have a description, it is not unique
define description as storable Text attribute with words index
// many objects have an image, it is not unique
define image as storable Image attribute
// many events have a timeStamp
define timeStamp as storable DateTime attribute
// urls, files and fragments have a path
define path as storable Text attribute
// external text sources have an encoding such as "UTF-8"
define encoding as storable Text attribute
// many systems require a login
define login as storable Text attribute with key and value index
// many systems require a password
define password as Text attribute
// users typically have an email
define email as storable Text attribute with value index
define isAuditEnabled as native method returning Boolean doing:
Java: return $store.isAuditEnabled();
C#: return $store.IsAuditEnabled();
Python2: return $store.isAuditEnabled()
Python3: return $store.isAuditEnabled()
JavaScript: return $store.isAuditEnabled();
define fetchLatestAuditMetadataId as native method receiving DbId dbId returning DbId doing:
Java: return $store.fetchLatestAuditMetadataId(dbId);
C#: return $store.FetchLatestAuditMetadataId(dbId);
Python2: return $store.fetchLatestAuditMetadataId(dbId)
Python3: return $store.fetchLatestAuditMetadataId(dbId)
JavaScript: return $store.fetchLatestAuditMetadataId(dbId);
define fetchAllAuditMetadataIds as native method receiving DbId dbId returning DbId[] doing:
Java: return $store.fetchAllAuditMetadataIds(dbId);
C#: return $store.FetchAllAuditMetadataIds(dbId);
Python2: return $store.fetchAllAuditMetadataIds(dbId)
Python3: return $store.fetchAllAuditMetadataIds(dbId)
JavaScript: return $store.fetchAllAuditMetadataIds(dbId);
define fetchAuditMetadata as native method receiving DbId dbId returning Document doing:
Java: return $store.fetchAuditMetadataAsDocument(dbId);
C#: return $store.FetchAuditMetadataAsDocument(dbId);
Python2: return $store.fetchAuditMetadataAsDocument(dbId)
Python3: return $store.fetchAuditMetadataAsDocument(dbId)
JavaScript: return $store.fetchAuditMetadataAsDocument(dbId);
define fetchDbIdsAffectedByAuditMetadataId as native method receiving DbId dbId returning DbId[] doing:
Java: return $store.fetchDbIdsAffectedByAuditMetadataId(dbId);
C#: return $store.fetchDbIdsAffectedByAuditMetadataId(dbId);
Python2: return $store.fetchDbIdsAffectedByAuditMetadataId(dbId)
Python3: return $store.fetchDbIdsAffectedByAuditMetadataId(dbId)
JavaScript: return $store.fetchDbIdsAffectedByAuditMetadataId(dbId);
define fetchLatestAuditRecord as native method receiving DbId dbId returning Document doing:
Java: return $store.fetchLatestAuditRecordAsDocument(dbId);
C#: return $store.FetchLatestAuditRecordAsDocument(dbId);
Python2: return $store.fetchLatestAuditRecordAsDocument(dbId)
Python3: return $store.fetchLatestAuditRecordAsDocument(dbId)
JavaScript: return $store.fetchLatestAuditRecordAsDocument(dbId);
define fetchAllAuditRecords as native method receiving DbId dbId returning Document[] doing:
Java: return $store.fetchAllAuditRecordsAsDocuments(dbId);
C#: return $store.FetchAllAuditRecordsAsDocuments(dbId);
Python2: return $store.fetchAllAuditRecordsAsDocuments(dbId)
Python3: return $store.fetchAllAuditRecordsAsDocuments(dbId)
JavaScript: return $store.fetchAllAuditRecordsAsDocuments(dbId);
define fetchAuditRecordsMatching as native method receiving Document auditPredicates = nothing and Document instancePredicates = nothing returning Document[] doing:
Java: return $store.fetchAuditRecordsMatchingAsDocuments(auditPredicates, instancePredicates);
C#: return $store.FetchAuditRecordsMatchingAsDocuments(auditPredicates, instancePredicates);
Python2: return $store.fetchAuditRecordsMatchingAsDocuments(auditPredicates, instancePredicates)
Python3: return $store.fetchAuditRecordsMatchingAsDocuments(auditPredicates, instancePredicates)
JavaScript: return $store.fetchAuditRecordsMatchingAsDocuments(auditPredicates, instancePredicates);
define deleteAuditRecord as native method receiving DbId dbId returning Boolean doing:
Java: return $store.deleteAuditRecord(dbId);
C#: return $store.DeleteAuditRecord(dbId);
Python2: return $store.deleteAuditRecord(dbId)
Python3: return $store.deleteAuditRecord(dbId)
JavaScript: return $store.deleteAuditRecord(dbId);
define deleteAuditMetadata as native method receiving DbId dbId returning Boolean doing:
Java: return $store.deleteAuditMetadata(dbId);
C#: return $store.DeleteAuditMetadata(dbId);
Python2: return $store.deleteAuditMetadata(dbId)
Python3: return $store.deleteAuditMetadata(dbId)
JavaScript: return $store.deleteAuditMetadata(dbId);
define Category as native category with attribute name, and bindings:
define category bindings as:
Java: prompto.declaration.CategoryDeclaration
C#: prompto.declaration.CategoryDeclaration
Python2: CategoryDeclaration from module: prompto.declaration.CategoryDeclaration
Python3: CategoryDeclaration from module: prompto.declaration.CategoryDeclaration
JavaScript: CategoryDeclaration from module: prompto/declaration/CategoryDeclaration.js
define getCurrentCloud as native method returning Text doing:
Java: return prompto.cloud.Cloud.current().name();
define getApplicationConfiguration as native method returning Document doing:
Java: return prompto.runtime.Standalone.getApplicationConfiguration();
define Error as enumerated category with attributes name and text, and symbols:
DIVIDE_BY_ZERO with "Divide by zero!" as text
INDEX_OUT_OF_RANGE with "Index out of range!" as text
NULL_REFERENCE with "Null reference!" as text
NOT_MUTABLE with "Not a mutable object!" as text
NOT_STORABLE with "Not a storable object!" as text
AUDIT_DISABLED with "Audit is disabled!" as text
READ_WRITE with "Read/write failed!" as text
define DecimalConstant as enumerated Decimal with symbols:
PI with 3.14159265358979323846 as value
E with 2.7182818284590452354 as value
define parseInteger as native method receiving Text text returning Integer doing:
Java: return Long.parseLong(text);
C#: return System.Int64.Parse(text);
Python2: return int(text)
Python3: return int(text)
JavaScript: return parseInt(text);
define parseDecimal as native method receiving Text text returning Decimal doing:
Java: return Double.parseDouble(text);
C#: return System.Double.Parse(text, System.Globalization.CultureInfo.InvariantCulture);
Python2: return float(text)
Python3: return float(text)
JavaScript: return parseFloat(text);
define parseDate as native method receiving Text text returning Date doing:
Java: return prompto.intrinsic.PromptoDate.parse(text);
C#: return prompto.value.DateValue.Parse(text);
Python2: return DateValue.Parse(text) from module: prompto.value.DateValue
Python3: return DateValue.Parse(text) from module: prompto.value.DateValue
JavaScript: return LocalDate.parse(text); from module: prompto/intrinsic/LocalDate.js
define parseTime as native method receiving Text text returning Time doing:
Java: return prompto.intrinsic.PromptoTime.parse(text);
C#: return prompto.value.TimeValue.Parse(text);
Python2: return TimeValue.Parse(text) from module: prompto.value.TimeValue
Python3: return TimeValue.Parse(text) from module: prompto.value.TimeValue
JavaScript: return LocalTime.parse(text); from module: prompto/intrinsic/LocalTime.js
define parseDateTime as native method receiving Text text returning DateTime doing:
Java: return prompto.intrinsic.PromptoDateTime.parse(text);
C#: return prompto.value.DateTimeValue.Parse(text);
Python2: return DateTimeValue.Parse(text) from module: prompto.value.DateTimeValue
Python3: return DateTimeValue.Parse(text) from module: prompto.value.DateTimeValue
JavaScript: return DateTime.parse(text); from module: prompto/intrinsic/DateTime.js
define parseUuid as native method receiving Text text returning Uuid doing:
Java: return java.util.UUID.fromString(text);
C#: return System.Guid.Parse(text);
Python2: return UUID(text) from module: uuid
Python3: return UUID(text) from module: uuid
JavaScript: return UUID.fromString(text); from module: prompto/intrinsic/UUID.js
define parseVersion as native method receiving Text text returning Version doing:
Java: return prompto.intrinsic.PromptoVersion.parse(text);
C#: return prompto.value.VersionValue.Parse(text);
Python2: return VersionValue.Parse(text) from module: prompto.value.VersionValue
Python3: return VersionValue.Parse(text) from module: prompto.value.VersionValue
JavaScript: return Version.parse(text); from module: prompto/intrinsic/Version.js
define parsePeriod as native method receiving Text text returning Period doing:
Java: return prompto.intrinsic.PromptoPeriod.parse(text);
C#: return prompto.value.PeriodValue.Parse(text);
Python2: return PeriodValue.Parse(text) from module: prompto.value.PeriodValue
Python3: return PeriodValue.Parse(text) from module: prompto.value.PeriodValue
JavaScript: return Period.parse(text); from module: prompto/intrinsic/Period.js
define "parses Integer" as test method doing:
i = parseInteger "123"
and verifying:
i = 123
define "parses Integer with leading 0" as test method doing:
i = parseInteger "0123"
and verifying:
i = 123
define "parses Decimal" as test method doing:
i = parseDecimal "123.45"
and verifying:
i = 123.45
define "parses Date" as test method doing:
d = parseDate "2018-02-17"
and verifying:
d = '2018-02-17'
define "parses Time" as test method doing:
t = parseTime "16:15:18"
and verifying:
t = '16:15:18'
define "parses DateTime" as test method doing:
dt = parseDateTime "2018-02-17T16:15:18"
and verifying:
dt = '2018-02-17T16:15:18'
define "parses Uuid" as test method doing:
u = parseUuid "11223344-aabb-ccdd-eeff-112233445566"
and verifying:
u = '11223344-aabb-ccdd-eeff-112233445566'
define "parses Version" as test method doing:
v = parseVersion "v1.2.3"
and verifying:
v = 'v1.2.3'
define "parses Period" as test method doing:
p = parsePeriod "PT1S"
and verifying:
p = 'PT1S'
define now as native method returning DateTime doing:
Java: return prompto.intrinsic.PromptoDateTime.now();
C#: return System.DateTimeOffset.Now;
Python2: return datetime.utcnow() from module: datetime
Python3: return datetime.utcnow() from module: datetime
JavaScript: return DateTime.now(); from module: prompto/intrinsic/DateTime.js
define "now is a DateTime" as test method doing:
a = now
and verifying:
a is a DateTime
define today as method returning Date doing:
return now.date
define "today is a Date" as test method doing:
a = today
and verifying:
a is a Date
// waits for n milliseconds (consuming all CPU in JavaScript)
define sleep as native method receiving Integer millis doing:
Java: java.lang.Thread.sleep(millis);
C#: prompto.utils.Utils.Sleep(millis);
Python2: sleep(millis) from module: prompto.utils.Utils
Python3: sleep(millis) from module: prompto.utils.Utils
JavaScript: sleep(millis); from module: prompto/utils/Utils.js
define "sleeps for 1 second" as test method doing:
start = now
sleep 1000
end = now
and verifying:
end >= start + 'PT1S'
define scheduleJob as native method receiving Callback callback, DateTime executeAt, Period repeatEvery = Nothing and Text jobName = Nothing returning Integer doing:
Java: return prompto.runtime.Scheduler.schedule(callback, executeAt, repeatEvery, jobName);
C#: return prompto.runtime.Scheduler.schedule(callback, executeAt, repeatEvery, jobName);
Python2: return Scheduler.schedule(callback, executeAt, repeatEvery, jobName) from module: prompto.runtime.Scheduler
Python3: return Scheduler.schedule(callback, executeAt, repeatEvery, jobName) from module: prompto.runtime.Scheduler
JavaScript: return Scheduler.schedule(callback, executeAt, repeatEvery, jobName); from module: prompto/runtime/Scheduler.js
define cancelJob as native method receiving Integer jobId doing:
Java: prompto.runtime.Scheduler.cancel(jobId);
C#: prompto.runtime.Scheduler.cancel(jobId);
Python2: Scheduler.cancel(jobId) from module: prompto.runtime.Scheduler
Python3: Scheduler.cancel(jobId) from module: prompto.runtime.Scheduler
JavaScript: Scheduler.cancel(jobId); from module: prompto/runtime/Scheduler.js
// returns a random UUID as defined by http://www.ietf.org/rfc/rfc4122.txt
define randomUuid as native method returning Uuid doing:
Java: return java.util.UUID.randomUUID();
C#: return System.Guid.NewGuid();
Python2: return uuid4() from module: uuid
Python3: return uuid4() from module: uuid
JavaScript: return UUID.create(); from module: prompto/intrinsic/UUID.js
define "randomUuid is not null" as test method doing:
uuid = randomUuid
and verifying:
uuid is not Nothing
define isServer as native method returning Boolean doing:
Java: return $context.isServer();
C#: return false;
Python2: return False
Python3: return False
JavaScript: return false;
define fetchTextResource as native method receiving path returning Text doing:
Java: return $context.fetchTextResource(path);
C#: return $context.fetchTextResource(path);
Python2: return $context.fetchTextResource(path)
Python3: return $context.fetchTextResource(path)
JavaScript: return $context.fetchTextResource(path);
define fetchBinaryResource as native method receiving path returning Blob doing:
Java: return $context.fetchBinaryResource(path);
C#: return $context.fetchBinaryResource(path);
Python2: return $context.fetchBinaryResource(path)
Python3: return $context.fetchBinaryResource(path)
JavaScript: return $context.fetchBinaryResource(path);
define nextSequenceValue as native method receiving Text name returning Integer doing:
Java: return $store.nextSequenceValue(name);
C#: return $store.NextSequenceValue(name);
Python2: return $store.nextSequenceValue(name)
Python3: return $store.nextSequenceValue(name)
JavaScript: return $store.nextSequenceValue(name);
define "nextSequenceValue increases" as test method doing:
v1 = nextSequenceValue "BIG"
v2 = nextSequenceValue "BIG"
v3 = nextSequenceValue "BIG"
and verifying:
v1 = 1
v2 = 2
v3 = 3
define Buffer as native resource with attribute text, and bindings:
define category bindings as:
Java: prompto.io.Buffer
C#: prompto.io.Buffer
Python2: Buffer from module: prompto.io.Buffer
Python3: Buffer from module: prompto.io.Buffer
JavaScript: Buffer from module: prompto/io/Buffer.js
define "writes to Buffer" as test method doing:
content = ""
with buffer = Buffer, do:
write "some content" to buffer
write "other content" to buffer
content = buffer.text
and verifying:
"some content" in content
"other content" in content
// a wrapper around native console printing
define printNative as native method receiving any value and NativeWriter writer doing:
Java: writer.print(value);
C#: writer.Write(value);
Python2: print(objects=str(value), end="", file=writer)
Python3: print(objects=str(value), end="", file=writer)
JavaScript: writer.write(value.toString());
// a wrapper around heterogeneous native console io
define NativeWriter as native category with bindings:
define category bindings as:
Java: java.io.PrintStream
C#: System.IO.TextWriter
Python2: StringIO from module: StringIO
Python3: StringIO from module: io
JavaScript: writer from module: prompto/io/io.js
// a wrapper around heterogeneous native stdout
define stdout as native method returning NativeWriter doing:
Java: return System.out;
C#: return System.Console.Out;
Python2: return stdout from module: sys
Python3: return stdout from module: sys
JavaScript: return stdout; from module: prompto/io/io.js
// a wrapper around heterogeneous native stdin
define stderr as native method returning NativeWriter doing:
Java: return System.err;
C#: return System.Console.Error;
Python2: return stderr from module: sys
Python3: return stderr from module: sys
JavaScript: return stderr; from module: prompto/io/io.js
// an abstract Writer which can be derived (unlike NativeWriter)
define Writer as category with methods:
define print as abstract method receiving any value
// the native Writer implementation
define nativeWriter as NativeWriter attribute
define ConsoleWriter as Writer with attribute nativeWriter, and methods:
define print as method receiving any value doing:
printNative value with nativeWriter as writer
// the buffered Writer implementation (useful for testing)
define TextWriter as Writer with attribute text, and methods:
define print as method receiving any value doing:
if text is nothing:
text = "" + value
else:
text = text + value
// now the interesting methods
define print as method receiving any value doing:
out = invoke: stdout
printNative value with out as writer
define printLine as method receiving any value doing:
out = invoke: stdout
value = "" + value + "\n"
printNative value with out as writer
define print as method receiving any value and Writer writer doing:
writer.print with value as value
define printLine as method receiving any value and Writer writer doing:
print value with writer as writer
print '\n' with writer as writer
// and the corresponding tests
define "print a text" as test method doing:
w = mutable TextWriter
print "Hello" with w as writer
print " John" with w as writer
and verifying:
w.text = "Hello John"
define "print an integer" as test method doing:
w = mutable TextWriter
print 12345 with w as writer
and verifying:
w.text = "12345"
define "printLine a text" as test method doing:
w = mutable TextWriter
print "Hello" with w as writer
printLine " John" with w as writer
and verifying:
w.text = "Hello John\n"
define "printLine an integer" as test method doing:
w = mutable TextWriter
printLine 12345 with w as writer
and verifying:
w.text = "12345\n"
define Email as native category with bindings:
define category bindings as:
Java: prompto.internet.Email
C#: prompto.internet.Email
Python2: Email from module: prompto.internet.Email
Python3: Email from module: prompto.internet.Email
JavaScript: Email from module: prompto/internet/Email.js
and methods:
define setFrom as method receiving email and name = nothing doing:
Java: this.setFrom(email, name);
C#: this.setFrom(email, name);
Python2: this.setFrom(email, name)
Python3: this.setFrom(email, name);
JavaScript: this.setFrom(email, name);
define addTo as method receiving email and name = nothing doing:
Java: this.addTo(email, name);
C#: this.addTo(email, name);
Python2: this.addTo(email, name)
Python3: this.addTo(email, name);
JavaScript: this.addTo(email, name);
define addCC as method receiving email and name = nothing doing:
Java: this.addCC(email, name);
C#: this.addCC(email, name);
Python2: this.addCC(email, name)
Python3: this.addCC(email, name);
JavaScript: this.addCC(email, name);
define addBCC as method receiving email and name = nothing doing:
Java: this.addBCC(email, name);
C#: this.addBCC(email, name);
Python2: this.addBCC(email, name)
Python3: this.addBCC(email, name);
JavaScript: this.addBCC(email, name);
define setSubject as method receiving Text subject doing:
Java: this.setSubject(subject);
C#: this.setSubject(subject);
Python2: this.setSubject(subject)
Python3: this.setSubject(subject);
JavaScript: this.setSubject(subject);
define addBody as method receiving Text body and Text mimeType doing:
Java: this.addBody(body, mimeType);
C#: this.addBody(body, mimeType);
Python2: this.addBody(body, mimeType)
Python3: this.addBody(body, mimeType);
JavaScript: this.addBody(body, mimeType);
define send as method receiving Text hostName = Nothing, Integer port = -1, Boolean useTLS = false, login = Nothing, password = Nothing and Boolean useSSL = false doing:
Java: this.send(hostName, port, useTLS, login, password, useSSL);
C#: this.send(hostName, port, useTLS, login, password, useSSL);
Python2: this.send(hostName, useTLS, port, login, password, useSSL)
Python3: this.send(hostName, useTLS, port, login, password, useSSL);
JavaScript: this.send(hostName, useTLS, port, login, password, useSSL);
define htmlEncode as native method receiving Text value returning Text doing:
Java: return prompto.internet.Html.encode(value);
C#: return System.Net.WebUtility.HtmlEncode(value);
Python2: return escape(value) from module: cgi
Python3: return escape(value) from module: html
JavaScript: return htmlEncode(value); from module: prompto/internet/Html.js
define htmlDecode as native method receiving Text value returning Text doing:
Java: return prompto.internet.Html.decode(value);
C#: return System.Net.WebUtility.HtmlDecode(value);
Python2: return HTMLParser().unescape(value) from module: HTMLParser
Python3: return unescape(value) from module: html.parser
JavaScript: return htmlDecode(value); from module: prompto/internet/Html.js
define "encode Html entity" as test method doing:
encoded = htmlEncode ""
and verifying:
encoded = "<ab>"
define "decode Html entity" as test method doing:
encoded = htmlDecode "<ab>"
and verifying:
encoded = ""
define "decode Html charcode" as test method doing:
encoded = htmlDecode "a1b"
and verifying:
encoded = "a1b"
define HttpMethod as enumerated Text with symbols:
HTTP_CONNECT with "CONNECT" as value
HTTP_DELETE with "DELETE" as value
HTTP_GET with "GET" as value
HTTP_HEAD with "HEAD" as value
HTTP_OPTIONS with "OPTIONS" as value
HTTP_PATCH with "PATCH" as value
HTTP_POST with "POST" as value
HTTP_PUT with "PUT" as value
HTTP_TRACE with "TRACE" as value
define httpMethod as storable HttpMethod attribute
define HttpHeader as native category with attributes name and text, and bindings:
define category bindings as:
Java: prompto.internet.HttpHeader
C#: prompto.internet.HttpHeader
Python2: HttpHeader from module: prompto.internet.HttpHeader
Python3: HttpHeader from module: prompto.internet.HttpHeader
JavaScript: HttpHeader from module: prompto/internet/HttpHeader.js
define httpHeaders as HttpHeader[] attribute
define HttpContentType as enumerated Text with symbols:
HTTP_URL_ENCODED with "application/x-www-form-urlencoded" as value
HTTP_FORM_DATA with "multipart/form-data" as value
HTTP_TEXT_PLAIN with "text/plain" as value
HTTP_APPLICATION_JSON with "application/json" as value
define Url as native resource with attributes path, encoding, httpMethod and httpHeaders, and bindings:
define category bindings as:
Java: prompto.internet.Url
C#: prompto.internet.Url
Python2: Url from module: prompto.internet.Url
Python3: Url from module: prompto.internet.Url
JavaScript: Url from module: prompto/internet/Url.js
define url as Url attribute
define "reads from www.google.com" as test method doing:
// need a cross-domain enabled page for browser testing
content = read all from Url with "https://www.google.com/" as path
and verifying:
content contains "doctype"
define "reads async from www.google.com" as test method doing:
// need a cross-domain enabled page for browser testing
content = ""
read all from Url with "https://www.google.com/" as path then with result:
content = result
and verifying:
content contains "doctype"
define encodeURI as native method receiving Text uri returning Text doing:
Java: return java.net.URLEncoder.encode(uri, "UTF-8");
C#: return System.Web.HttpUtility.UrlEncode(uri);
Python2: return quote(uri) from module: urllib
Python3: return quote(uri) from module: urllib.parse
JavaScript: return encodeURIComponent(uri);
define decodeURI as native method receiving Text uri returning Text doing:
Java: return java.net.URLDecoder.decode(uri, "UTF-8");
C#: return System.Web.HttpUtility.UrlDecode(uri);
Python2: return unquote(uri) from module: urllib
Python3: return unquote(uri) from module: urllib.parse
JavaScript: return decodeURIComponent(uri);
define "encodes and decodes uri" as test method doing:
uri = "Black Mamba"
encoded = encodeURI uri
decoded = decodeURI encoded
and verifying:
encoded = "Black%20Mamba" or encoded = "Black+Mamba"
uri = decoded
define listRootPaths as native method returning Text[] doing:
Java: return prompto.path.Path.listRoots();
C#: return prompto.path.Path.listRoots();
Python2: return listRoots() from module: prompto.path.Path
Python3: return listRoots() from module: prompto.path.Path
JavaScript: return listRoots(); from module: prompto/path/Path.js
define "listRootPaths returns a Text[]" as test method doing:
roots = listRootPaths
and verifying:
roots is a Text[]
roots.count > 0
define listChildPaths as native method receiving path returning Text[] doing:
Java: return prompto.path.Path.listChildren(path);
C#: return prompto.path.Path.listChildren(path);
Python2: return listChildren(path) from module: prompto.path.Path
Python3: return listChildren(path) from module: prompto.path.Path
JavaScript: return listChildren(path); from module: prompto/path/Path.js
define "listChildPaths returns a Text[]" as test method doing:
children = listChildPaths "/"
and verifying:
children is a Text[]
children.count > 0
define pathExists as native method receiving path returning Boolean doing:
Java: return prompto.path.Path.pathExists(path);
C#: return prompto.path.Path.pathExists(path);
Python2: return pathExists(path) from module: prompto.path.Path
Python3: return pathExists(path) from module: prompto.path.Path
JavaScript: return pathExists(path); from module: prompto/path/Path.js
define "pathExists returns a Boolean" as test method doing:
exists = pathExists "/"
and verifying:
exists is true
define pathIsFile as native method receiving path returning Boolean doing:
Java: return prompto.path.Path.pathIsFile(path);
C#: return prompto.path.Path.pathIsFile(path);
Python2: return pathIsFile(path) from module: prompto.path.Path
Python3: return pathIsFile(path) from module: prompto.path.Path
JavaScript: return pathIsFile(path); from module: prompto/path/Path.js
define "pathIsFile returns a Boolean" as test method doing:
isFile = pathIsFile "/"
and verifying:
isFile is false
define pathIsDirectory as native method receiving path returning Boolean doing:
Java: return prompto.path.Path.pathIsDirectory(path);
C#: return prompto.path.Path.pathIsDirectory(path);
Python2: return pathIsDirectory(path) from module: prompto.path.Path
Python3: return pathIsDirectory(path) from module: prompto.path.Path
JavaScript: return pathIsDirectory(path); from module: prompto/path/Path.js
define "pathIsDirectory returns a Boolean" as test method doing:
isDir = pathIsDirectory "/"
and verifying:
isDir is true
define pathIsLink as native method receiving path returning Boolean doing:
Java: return prompto.path.Path.pathIsLink(path);
C#: return prompto.path.Path.pathIsLink(path);
Python2: return pathIsLink(path) from module: prompto.path.Path
Python3: return pathIsLink(path) from module: prompto.path.Path
JavaScript: return pathIsLink(path); from module: prompto/path/Path.js
define "pathIsLink returns a Boolean" as test method doing:
isLink = pathIsLink "/"
and verifying:
isLink is false
define compressToTempPath as native method receiving path returning Text doing:
Java: return prompto.path.Path.compressToTempPath(path);
C#: return prompto.path.Path.compressToTempPath(path);
Python2: return compressToTempPath(path) from module: prompto.path.Path
Python3: return compressToTempPath(path) from module: prompto.path.Path
JavaScript: return compressToTempPath(path); from module: prompto/path/Path.js
define decompressToTempPath as native method receiving path returning Text doing:
Java: return prompto.path.Path.decompressToTempPath(path);
C#: return prompto.path.Path.decompressToTempPath(path);
Python2: return decompressToTempPath(path) from module: prompto.path.Path
Python3: return decompressToTempPath(path) from module: prompto.path.Path
JavaScript: return decompressToTempPath(path); from module: prompto/path/Path.js
define sysGetEnvironmentVariable as native method receiving Text name returning Text doing:
Java: return java.lang.System.getenv(name);
C#: return System.Environment.GetEnvironmentVariable(name);
Python2: return getenv(name) from module: os
Python3: return getenv(name) from module: os
JavaScript: return process.env[name];
define "sysGetEnvironmentVariable returns a Text" as test method doing:
home = sysGetEnvironmentVariable "HOME"
and verifying:
home is a Text
define iterateCsv as native method receiving text, Text<:> columnNames = nothing, Character separator = ',', Character quote = '"' returning Iterator doing:
Java: return prompto.reader.CSVReader.iterator(text, columnNames, separator, quote);
C#: return prompto.reader.CSVReader.iterator(text, columnNames, separator, quote);
Python2: return csvIterate(text, columnNames, separator, quote) from module: prompto.reader.CSVReader
Python3: return csvIterate(text, columnNames, separator, quote) from module: prompto.reader.CSVReader
JavaScript: return csvIterate(text, columnNames, separator, quote); from module: prompto/reader/CSVReader.js
define readCsv as native method receiving text, Text<:> columnNames = nothing, Character separator = ',', Character quote = '"' returning Document[] doing:
Java: return prompto.reader.CSVReader.read(text, columnNames, separator, quote);
C#: return prompto.reader.CSVReader.read(text, columnNames, separator, quote);
Python2: return csvRead(text, columnNames, separator, quote) from module: prompto.reader.CSVReader
Python3: return csvRead(text, columnNames, separator, quote) from module: prompto.reader.CSVReader
JavaScript: return csvRead(text, columnNames, separator, quote); from module: prompto/reader/CSVReader.js
define readCsvHeaders as native method receiving text, Character separator = ',', Character quote = '"' returning Text[] doing:
Java: return prompto.reader.CSVReader.readHeaders(text, separator, quote);
C#: return prompto.reader.CSVReader.readHeaders(text, separator, quote);
Python2: return csvReadHeaders(text, separator, quote) from module: prompto.reader.CSVReader
Python3: return csvReadHeaders(text, separator, quote) from module: prompto.reader.CSVReader
JavaScript: return csvReadHeaders(text, separator, quote); from module: prompto/reader/CSVReader.js
define "reads from csv text" as test method doing:
docs = readCsv with "id,name\n1,John\n2,Riou\\, Sylvie\n" as text
and verifying:
docs.count = 2
docs[1].id = "1"
docs[1].name = "John"
docs[2].id = "2"
docs[2].name = "Riou, Sylvie"
define "reads headers from csv text" as test method doing:
headers = readCsvHeaders with "id,name\n1,John\n2,Riou\\, Sylvie\n" as text
and verifying:
headers.count = 2
headers[1] = "id"
headers[2] = "name"
define "iterates from csv text" as test method doing:
iterCsv = iterateCsv with "id,name\n1,John\n2,Riou\\, Sylvie\n" as text
docs = [] as Document[]
for each doc in iterCsv:
docs = docs + [doc]
and verifying:
docs.count = 2
docs[1].id = "1"
docs[1].name = "John"
docs[2].id = "2"
docs[2].name = "Riou, Sylvie"
define "maps csv headers" as test method doing:
names = < "Code" : "id", "Name" : "name" >
docs = readCsv with "Code,Name\n1,John\n2,Riou\\, Sylvie\n" as text and names as columnNames
and verifying:
docs.count = 2
docs[1].id = "1"
docs[1].name = "John"
docs[2].id = "2"
docs[2].name = "Riou, Sylvie"
define writeCsv as native method receiving Document[] docs, Text[] columnsList, Text<:> columnNames = nothing, Character separator = ',', Character quote = '"' returning Text doing:
Java: return prompto.writer.CSVWriter.write(docs, columnsList, columnNames, separator, quote);
C#: return prompto.writer.CSVWriter.write(docs, columnsList, columnNames, separator, quote);
Python2: return csvWrite(docs, columnsList, columnNames, separator, quote) from module: prompto.writer.CSVWriter
Python3: return csvWrite(docs, columnsList, columnNames, separator, quote) from module: prompto.writer.CSVWriter
JavaScript: return csvWrite(docs, columnsList, columnNames, separator, quote); from module: prompto/writer/CSVWriter.js
define "writes csv to text" as test method doing:
doc = { firstName: "John", lastName: "Smith", birthDate: '1212-12-12', salary: 22222 }
text = writeCsv with [ doc ] as docs, ["firstName", "lastName", "birthDate", "salary"] as columnsList, < "firstName": "First name", "lastName" : "Last name", "birthDate": "Birth date" > as columnNames
and verifying:
text = "First name,Last name,Birth date,salary\nJohn,Smith,1212-12-12,22222\n"
define readJson as native method receiving text returning Any doing:
Java: return prompto.reader.JSONReader.read(text);
C#: return prompto.reader.JSONReader.read(text);
Python2: return jsonRead(text) from module: prompto.reader.JSONReader
Python3: return jsonRead(text) from module: prompto.reader.JSONReader
JavaScript: return jsonRead(text); from module: prompto/reader/JSONReader.js
define "test that readJson returns a boolean" as test method doing:
o = readJson "true"
and verifying:
o is a Boolean
o is true
define "test that readJson returns an Integer" as test method doing:
o = readJson "237"
and verifying:
o is an Integer
o = 237
define "test that readJson returns a Decimal" as test method doing:
o = readJson "123.02"
and verifying:
o is a Decimal
o = 123.02
define "test that readJson returns a Text" as test method doing:
o = readJson "\"some text\""
and verifying:
o is a Text
o = "some text"
define "test that readJson returns a Document" as test method doing:
o = (readJson "{\"field\":122}") as Document
and verifying:
o is a Document
o.field = 122
define "test that readJson returns a List" as test method doing:
o = (readJson "[{\"field\":122}]") as Document[]
and verifying:
o is a Document[]
o[1].field = 122
define "test that readJson returns a complex Document" as test method doing:
json = "[{\"field\":122, \"list\": [{\"field\":123, \"doc\": {\"name\": \"John\"}}], \"doc\": { \"list\": [ 122, 233, 344] }}]"
o = (readJson json) as Document[]
and verifying:
o is a Document[]
o[1].field = 122
define readYaml as native method receiving text returning Document[] doing:
Java: return prompto.reader.YAMLReader.read(text);
C#: return prompto.reader.YAMLReader.read(text);
Python2: return yamlRead(text) from module: prompto.reader.YAMLReader
Python3: return yamlRead(text) from module: prompto.reader.YAMLReader
JavaScript: return yamlRead(text); from module: prompto/reader/YAMLReader.js
define "test that readYaml returns a complex Document" as test method doing:
yaml = "field: 122\ndata:\n list:\n - field: 123\n - doc:\n name: John\n doc:\n list:\n - 122\n - 233\n - 344"
o = readYaml yaml
and verifying:
o is a Document[]
o[1].field = 122
define writeYaml as native method receiving Document[] docs returning Text doing:
Java: return prompto.writer.YAMLWriter.write(docs);
C#: return prompto.writer.YAMLWriter.write(docs);
Python2: return yamlWrite(docs) from module: prompto.writer.YAMLWriter
Python3: return yamlWrite(docs) from module: prompto.writer.YAMLWriter
JavaScript: return yamlWrite(docs); from module: prompto/writer/YAMLWriter.js
define "test that writeYaml writes a complex Document" as test method doing:
src = [{ field: 122, list: [ { field: 123 }, { doc: { name: "John", doc: { list: [ 122, 233, 344 ] } } } ] }]
yaml = writeYaml src
dst = readYaml yaml
and verifying:
dst is a Document[]
dst = src
define LoginFactory as native category with bindings:
define category bindings as:
Java: prompto.security.auth.source.IAuthenticationSource
and methods:
define createLogin as method receiving login and password doing:
Java: this.createLogin(login, password);
define updateLogin as method receiving login and password doing:
Java: this.updateLogin(login, password);
define checkLogin as method receiving login and password returning Boolean doing:
Java: return this.checkLogin(login, password);
define hasLogin as method receiving login returning Boolean doing:
Java: return this.hasLogin(login);
define close as method doing:
Java: this.close();
define serviceHandler as abstract method receiving Document doc
define installServiceHandler as native method receiving Text path and serviceHandler doing:
Java: $server.installHandler(path, serviceHandler);
define getLoginFactory as native method receiving Text config = nothing returning LoginFactory doing:
Java: $server.getLoginFactory(config);
define getHttpUser as native method returning Text doing:
Java: return $server.getHttpUser();
define getHttpSession as native method returning Document doing:
Java: return $server.getHttpSession();
define httpLogout as native method receiving Text path doing:
Java: $server.httpLogout(path);
define getHttpPort as native method returning Integer doing:
Java: return $server.getHttpPort();
JavaScript: return self.location.port;
define getHttpQueryParameter as native method receiving Text name returning Text doing:
Java: return $server.getHttpQueryParameter(name);
JavaScript: return browserGetQueryParameter(name); from module: prompto/web/Browser.js
define httpRedirect as native method receiving Text path doing:
Java: $server.httpRedirect(path);
JavaScript: browserGoto(location); from module: prompto/web/Browser.js
@Inlined
define ClickEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
@Inlined
define MouseEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
@Inlined
define KeyboardEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
@Inlined
define RadioChangedEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define getSelectedRadio as method returning Text doing:
JavaScript: return this.target.id;
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
@Inlined
define InputChangedEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define getCurrentText as method returning Text doing:
JavaScript: return this.target.defaultValue;
define getProposedText as method returning Text doing:
JavaScript: return this.target.value;
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
@Inlined
define SubmitEvent as native category with bindings:
define category bindings as:
JavaScript: Event
and methods:
define stopPropagation as method doing:
JavaScript: this.stopPropagation();
define preventDefault as method doing:
JavaScript: this.preventDefault();
define ClickEventCallback as abstract method receiving ClickEvent event
define MouseEventCallback as abstract method receiving MouseEvent event
define KeyboardEventCallback as abstract method receiving KeyboardEvent event
// TODO kept for compatibility, but should be removed
define RadioChangedCallback as abstract method receiving RadioChangedEvent event
define RadioChangedEventCallback as abstract method receiving RadioChangedEvent event
// TODO kept for compatibility, but should be removed
define InputChangedCallback as abstract method receiving InputChangedEvent event
define InputChangedEventCallback as abstract method receiving InputChangedEvent event
define SubmitEventCallback as abstract method receiving SubmitEvent event
define DateChangedCallback as abstract method receiving Date value
define ToggleChangedCallback as abstract method receiving Boolean value
define ItemSelectedCallback as abstract method receiving Any value
define PageSelectedCallback as abstract method receiving Integer value
@Inlined
define FileRef as native category with bindings:
define category bindings as:
JavaScript: FileRef from module: prompto/web/FileRef.js
and methods:
define getName as method returning Text doing:
JavaScript: return this.file.name;
define getMimeType as method returning Text doing:
JavaScript: return this.file.type;
define getSize as method returning Integer doing:
JavaScript: return this.file.size;
define readText as method receiving TextCallback callback doing:
JavaScript: this.readText(callback);
define readImage as method returning Image doing:
JavaScript: return this.readImage();
define readBlob as method returning Blob doing:
JavaScript: return this.readBlob();
define FileRefCallback as abstract method receiving FileRef file
define selectFile as native method receiving FileRefCallback callback and Text<> mimeTypes = nothing doing:
JavaScript: selectFileRef(callback, mimeTypes); from module: prompto/web/FileRef.js
define getReactWidgetProps as native method receiving ReactWidget w returning Document doing:
JavaScript: return w.props;
define getReactWidgetState as native method receiving ReactWidget w returning Document doing:
JavaScript: return w.state;
define setReactWidgetState as native method receiving ReactWidget w, Document state and Callback callback = nothing doing:
JavaScript: w.setState(state, callback);
define ReactStateAndPropsUpdater as abstract method receiving Document state and Document props returning Document
define setReactWidgetState as native method receiving ReactWidget w, ReactStateAndPropsUpdater updater and Callback callback = nothing doing:
JavaScript: w.setState(updater, callback);
define forceReactWidgetRender as native method receiving ReactWidget w and Callback callback = nothing doing:
JavaScript: w.forceUpdate(callback);
define showReactModal as native method receiving Html dialog doing:
JavaScript: ReactUtils.showModal(dialog);
define hideReactModal as native method doing:
JavaScript: ReactUtils.hideModal();
define showReactContextMenu as native method receiving ClickEvent event and Html menu doing:
JavaScript: ReactUtils.showContextMenu(event, menu);
define hideReactContextMenu as native method doing:
JavaScript: ReactUtils.hideContextMenu();
@WidgetField(name="state", type=Document)
@WidgetField(name="refs", type=Document)
@WidgetField(name="props", type=Document, isProperties=true)
define ReactWidget as widget with methods:
@Static
@Callback
define getDerivedStateFromProps as method receiving Document props and Document state doing:
return Nothing
@Callback
define componentDidMount as method doing:
// nothing to do
@Callback
define render as abstract method returning Html
@Callback
define componentWillUnmount as method doing:
// nothing to do
@Callback
define shouldComponentUpdate as method receiving Document nextProps and Document nextState returning Boolean doing:
return true
@Callback
define getSnapshotBeforeUpdate as method receiving Document prevProps and Document prevState returning Boolean doing:
return nothing
@Callback
define componentDidUpdate as method receiving Document prevProps, Document prevState and Document snapshot returning Boolean doing:
// nothing to do
define getProperties as method doing:
return getReactWidgetProps self
define getState as method doing:
return getReactWidgetState self
define setState as method receiving Document state and Callback callback = nothing doing:
setReactWidgetState self with state and callback
define setState as method receiving ReactStateAndPropsUpdater updater and Callback callback = nothing doing:
setReactWidgetState self with updater and callback
define forceRender as method receiving Callback callback = nothing doing:
forceReactWidgetRender self with callback
define WidgetCallback as abstract method receiving any ref
define alert as native method receiving Text text doing:
JavaScript: alert(text);
define downloadFile as native method receiving Text url and Text name = Nothing doing:
JavaScript: downloadFile(url, name); from module: prompto/web/Browser.js
define openBrowser as native method receiving Text url, Text name = Nothing and Text methodName = "open" returning Document doing:
JavaScript: return openBrowser(methodName, url, name); from module: prompto/web/Browser.js
define browserHistoryBack as native method doing:
JavaScript: window.history.back();
define browserHistoryForward as native method doing:
JavaScript: window.history.forward();
define browserHistoryGo as native method receiving Integer count doing:
JavaScript: window.history.go(count);
define browserHistoryCount as native method returning Integer doing:
JavaScript: return window.history.length;
define browserGoto as native method receiving Text location doing:
JavaScript: browserGoto(location); from module: prompto/web/Browser.js
define KeyboardShortcutCallback as abstract method returning Boolean
define browserBindKeyboardShortcuts as native method receiving Text[] shortcuts and KeyboardShortcutCallback callback doing:
JavaScript: browserBindKeyboardShortcuts(shortcuts, callback); from module: prompto/web/Browser.js
define browserGetWindow as native method returning Document doing:
JavaScript: return window;
define WebSocketCallback as abstract method receiving WebSocket value
define openWebSocket as native method receiving Text url, AnyCallback onMessage, Callback onClose = nothing and WebSocketCallback callback = nothing doing:
JavaScript: openWebSocket(url, onMessage, onClose, callback); from module: prompto/web/WebSocket.js
@Inlined
define WebSocket as native category with bindings:
define category bindings as:
JavaScript: window.WebSocket
and methods:
define close as method doing:
JavaScript: this.close();
© 2015 - 2024 Weber Informatics LLC | Privacy Policy