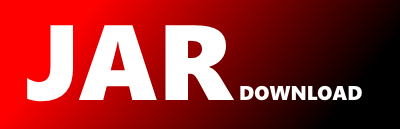
org.protelis.test.observer.SimpleExceptionObserver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protelis-test Show documentation
Show all versions of protelis-test Show documentation
Essential libraries for testing Protelis programs and simulations
/*
* Copyright (C) 2021, Danilo Pianini and contributors listed in the project's build.gradle.kts or pom.xml file.
*
* This file is part of Protelis, and is distributed under the terms of the GNU General Public License,
* with a linking exception, as described in the file LICENSE.txt in this project's top directory.
*/
package org.protelis.test.observer;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.Optional;
/**
* Simple exception observer.
*/
public final class SimpleExceptionObserver implements ExceptionObserver {
private final List exceptions = new LinkedList<>();
@Override
public Exception exceptionThrown(final Exception ex) {
exceptions.add(ex);
return ex;
}
@Override
public List getExceptionList() {
return Collections.unmodifiableList(exceptions);
}
@Override
public Optional getLastException() {
try {
return Optional.of(((LinkedList) exceptions).getLast());
} catch (NoSuchElementException e) {
return Optional.empty();
}
}
@Override
public Optional getFirstException() {
try {
return Optional.of(((LinkedList) exceptions).getFirst());
} catch (NoSuchElementException e) {
return Optional.empty();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy