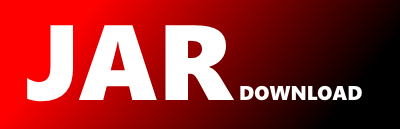
org.psjava.ds.graph.MutableBipartiteGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of psjava Show documentation
Show all versions of psjava Show documentation
Problem Solving Library for Java
package org.psjava.ds.graph;
import org.psjava.ds.Collection;
import org.psjava.ds.array.DynamicArray;
import org.psjava.ds.set.MutableSet;
import org.psjava.goods.GoodMutableSetFactory;
import org.psjava.util.AssertStatus;
import org.psjava.util.IterableToString;
public class MutableBipartiteGraph implements BipartiteGraph {
public static MutableBipartiteGraph create() {
return new MutableBipartiteGraph();
}
private MutableSet left = GoodMutableSetFactory.getInstance().create();
private MutableSet right = GoodMutableSetFactory.getInstance().create();
private DynamicArray> edges = DynamicArray.create();
public void insertLeftVertex(V v) {
AssertStatus.assertTrue(!right.contains(v));
left.insert(v);
}
public void insertRightVertex(V v) {
AssertStatus.assertTrue(!left.contains(v));
right.insert(v);
}
public void addEdge(final V leftv, final V rightv) {
assertVertexExist(leftv, left);
assertVertexExist(rightv, right);
edges.addToLast(SimpleBipartiteGraphEdge.create(leftv, rightv));
}
private void assertVertexExist(V v, MutableSet set) {
AssertStatus.assertTrue(set.contains(v), "vertex is not in graph");
}
@Override
public Collection getLeftVertices() {
return left;
}
@Override
public Collection getRightVertices() {
return right;
}
@Override
public Iterable> getEdges() {
return edges;
}
@Override
public String toString() {
return this.getClass().getSimpleName() + ":" + IterableToString.toString(getEdges());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy