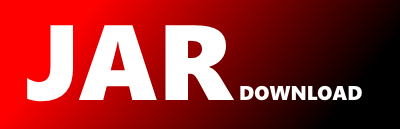
org.psjava.ds.tree.binary.BinaryTreeNodeUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of psjava Show documentation
Show all versions of psjava Show documentation
Problem Solving Library for Java
package org.psjava.ds.tree.binary;
import org.psjava.util.AssertStatus;
public class BinaryTreeNodeUtil {
public static boolean isLeftChild(BinaryTreeNodeWithParent node) {
return node.getParent().hasLeft() && node.getParent().getLeft() == node;
}
public static BinaryTreeNodeWithParent getAnyChild(BinaryTreeNodeWithParent node) {
if (node.hasLeft())
return node.getLeft();
else
return node.getRight();
}
public static void replaceNode(BinaryTreeNodeWithParent originalNode, BinaryTreeNodeWithParent newNode) {
BinaryTreeNodeWithParent parent = originalNode.getParent();
boolean left = isLeftChild(originalNode);
disconnectFromParent(originalNode);
if (left)
connectAsLeftChild(newNode, parent);
else
connectAsRightChild(newNode, parent);
}
public static void connectAsLeftChild(BinaryTreeNodeWithParent node, BinaryTreeNodeWithParent parent) {
AssertStatus.assertTrue(!parent.hasLeft());
AssertStatus.assertTrue(!node.hasParent());
parent.putLeft(node);
node.putParent(parent);
}
public static void connectAsRightChild(BinaryTreeNodeWithParent node, BinaryTreeNodeWithParent parent) {
AssertStatus.assertTrue(!parent.hasRight());
AssertStatus.assertTrue(!node.hasParent());
parent.putRight(node);
node.putParent(parent);
}
public static void disconnectFromParent(BinaryTreeNodeWithParent node) {
BinaryTreeNodeWithParent parent = node.getParent();
if (isLeftChild(node))
parent.removeLeft();
else
parent.removeRight();
node.removeParent();
}
private BinaryTreeNodeUtil() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy