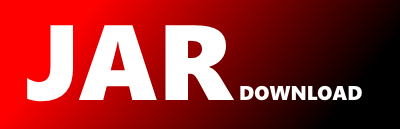
org.psjava.algo.graph.bfs.BFS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of psjava Show documentation
Show all versions of psjava Show documentation
Problem Solving Library for Java
The newest version!
package org.psjava.algo.graph.bfs;
import org.psjava.ds.graph.Graph;
import org.psjava.ds.graph.DirectedEdge;
import org.psjava.ds.queue.Queue;
import org.psjava.ds.set.MutableSet;
import org.psjava.goods.GoodMutableSetFactory;
import org.psjava.goods.GoodQueueFactory;
public class BFS {
private static class QueueItem {
int depth;
V v;
QueueItem(int depth, V v) {
this.depth = depth;
this.v = v;
}
}
public static > void traverse(Graph adj, Iterable startVertices, BFSVisitor visitor) {
MutableSet discovered = GoodMutableSetFactory.getInstance().create();
SimpleStopper stopper = new SimpleStopper();
Queue> queue = GoodQueueFactory.getInstance().create();
for (V v : startVertices) {
queue.enque(new QueueItem(0, v));
discovered.add(v);
visitor.onDiscover(v, 0, stopper);
if (stopper.isStopped())
break;
}
while (!queue.isEmpty() && !stopper.isStopped()) {
QueueItem cur = queue.deque();
for (E edge : adj.getEdges(cur.v)) {
V nextv = edge.to();
if (!discovered.contains(nextv)) {
discovered.add(nextv);
visitor.onWalk(edge);
visitor.onDiscover(nextv, cur.depth + 1, stopper);
if (stopper.isStopped())
break;
queue.enque(new QueueItem(cur.depth + 1, nextv));
}
}
}
}
private BFS() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy