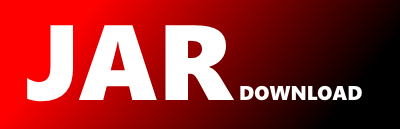
org.psjava.algo.graph.shortestpath.NegativeCycleFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of psjava Show documentation
Show all versions of psjava Show documentation
Problem Solving Library for Java
The newest version!
package org.psjava.algo.graph.shortestpath;
import org.psjava.ds.Collection;
import org.psjava.ds.deque.DoubleLinkedList;
import org.psjava.ds.graph.AllEdgeInGraph;
import org.psjava.ds.graph.DirectedWeightedEdge;
import org.psjava.ds.graph.Graph;
import org.psjava.ds.graph.MutableDirectedGraph;
import org.psjava.ds.numbersystrem.AddableNumberSystem;
import org.psjava.ds.numbersystrem.InfinitableAddableNumberSystem;
import org.psjava.ds.set.MutableSet;
import org.psjava.goods.GoodMutableSetFactory;
import org.psjava.util.AssertStatus;
/** Finds any one of possible negative cycles in a graph. */
public class NegativeCycleFinder {
private static class AugmentedEdge> implements DirectedWeightedEdge
© 2015 - 2025 Weber Informatics LLC | Privacy Policy