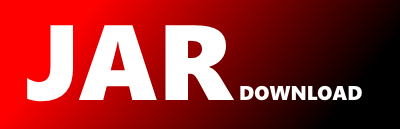
org.puimula.libvoikko.Dictionary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libvoikko Show documentation
Show all versions of libvoikko Show documentation
Java API for libvoikko, library of free natural language processing tools
/* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is Libvoikko: Library of natural language processing tools.
* The Initial Developer of the Original Code is Harri Pitkänen .
* Portions created by the Initial Developer are Copyright (C) 2010
* the Initial Developer. All Rights Reserved.
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*********************************************************************************/
package org.puimula.libvoikko;
/**
* Represents a morphological dictionary.
*/
public class Dictionary implements Comparable {
private final String language;
private final String script;
private final String variant;
private final String description;
public Dictionary(String language, String script, String variant, String description) {
this.language = language;
this.script = script;
this.variant = variant;
this.description = description;
}
/**
* @return the language part of BCP 47 language tag
*/
public String getLanguage() {
return language;
}
/**
* @return the script part of BCP 47 language tag or empty string if not specified
*/
public String getScript() {
return script;
}
/**
* @return the variant (BCP 47 private use tag)
*/
public String getVariant() {
return variant;
}
/**
* @return human readable description of the dictionary
*/
public String getDescription() {
return description;
}
public int compareTo(Dictionary arg0) {
int cmp = language.compareTo(arg0.getLanguage());
if (cmp != 0) {
return cmp;
}
cmp = script.compareTo(arg0.getScript());
if (cmp != 0) {
return cmp;
}
cmp = variant.compareTo(arg0.getVariant());
if (cmp != 0) {
return cmp;
}
return description.compareTo(arg0.getDescription());
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result
+ ((description == null) ? 0 : description.hashCode());
result = prime * result
+ ((language == null) ? 0 : language.hashCode());
result = prime * result
+ ((script == null) ? 0 : script.hashCode());
result = prime * result + ((variant == null) ? 0 : variant.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Dictionary other = (Dictionary) obj;
if (description == null) {
if (other.description != null)
return false;
} else if (!description.equals(other.description))
return false;
if (language == null) {
if (other.language != null)
return false;
} else if (!language.equals(other.language))
return false;
if (script == null) {
if (other.script != null)
return false;
} else if (!script.equals(other.script))
return false;
if (variant == null) {
if (other.variant != null)
return false;
} else if (!variant.equals(other.variant))
return false;
return true;
}
@Override
public String toString() {
return "<" + language + "," + script + "," + variant + "," + description + ">";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy