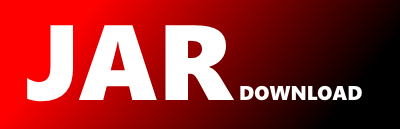
org.pure4j.collections.ASeq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pure4j-core Show documentation
Show all versions of pure4j-core Show documentation
Pure Functional Programming Semantics For Java
/**
* Copyright (c) Rich Hickey. All rights reserved.
* The use and distribution terms for this software are covered by the
* Eclipse Public License 1.0 (http://opensource.org/licenses/eclipse-1.0.php)
* which can be found in the file epl-v10.html at the root of this distribution.
* By using this software in any fashion, you are agreeing to be bound by
* the terms of this license.
* You must not remove this notice, or any other, from this software.
**/
package org.pure4j.collections;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.pure4j.Pure4J;
import org.pure4j.annotations.immutable.IgnoreImmutableTypeCheck;
import org.pure4j.annotations.pure.Enforcement;
import org.pure4j.annotations.pure.Pure;
public abstract class ASeq implements ISeq, List, Serializable {
private static final long serialVersionUID = 220865945544862915L;
@IgnoreImmutableTypeCheck
protected transient int _hasheq = -1;
public String toString() {
return ToStringFunctions.toString(this);
}
@SuppressWarnings("unchecked")
public IPersistentCollection empty() {
return (IPersistentCollection) PersistentList.emptyList();
}
protected ASeq() {
}
public boolean equals(Object obj) {
if (this == obj)
return true;
if (!(obj instanceof Seqable))
return false;
ISeq> ms = ((Seqable>)obj).seq();
for (ISeq s = seq(); s != null; s = s.next(), ms = ms.next()) {
if (ms == null || !Util.equals(s.first(), ms.first()))
return false;
}
return ms == null;
}
public int hashCode() {
if (_hasheq == -1) {
_hasheq = Hasher.hashOrdered(this);
}
return _hasheq;
}
public int count() {
int i = 1;
for (ISeq s = next(); s != null; s = s.next(), i++)
if (s instanceof Counted)
return i + s.count();
return i;
}
final public ISeq seq() {
return this;
}
public ISeq cons(K o) {
Pure4J.immutable(o);
return new Cons(o, this);
}
public ISeq more() {
ISeq s = next();
if (s == null)
return PersistentList.emptySeq();
return s;
}
public Object[] toArray() {
return PureCollections.seqToArray(seq());
}
@SuppressWarnings("unchecked")
@Pure(Enforcement.FORCE)
public T[] toArray(T[] a) {
return (T[]) PureCollections.seqToNewArray(seq(), a);
}
public boolean add(K o) {
Pure4J.unsupported();
return true;
}
public boolean remove(Object o) {
Pure4J.unsupported();
return true;
}
public boolean addAll(Collection extends K> c) {
Pure4J.unsupported();
return true;
}
public void clear() {
Pure4J.unsupported();
}
public boolean retainAll(Collection> c) {
Pure4J.unsupported();
return true;
}
public boolean removeAll(Collection> c) {
Pure4J.unsupported();
return true;
}
public boolean containsAll(Collection> c) {
Pure4J.immutable(c);
for (Object o : c) {
if (!contains(o))
return false;
}
return true;
}
public int size() {
return count();
}
public boolean isEmpty() {
return seq() == null;
}
public boolean contains(Object o) {
Pure4J.immutable(o);
for (ISeq s = seq(); s != null; s = s.next()) {
if (Util.equals(s.first(), o))
return true;
}
return false;
}
public IPureIterator iterator() {
return new SeqIterator(this);
}
// ////////// List stuff /////////////////
@Pure(Enforcement.FORCE) // no side-effects
private List reify() {
return Collections.unmodifiableList(new ArrayList(this));
}
public List subList(int fromIndex, int toIndex) {
return reify().subList(fromIndex, toIndex);
}
public K set(int index, Object element) {
Pure4J.unsupported();
return null;
}
public K remove(int index) {
Pure4J.unsupported();
return null;
}
public int indexOf(Object o) {
Pure4J.immutable(o);
ISeq s = seq();
for (int i = 0; s != null; s = s.next(), i++) {
if (Util.equals(s.first(), o))
return i;
}
return -1;
}
public int lastIndexOf(Object o) {
Pure4J.immutable(o);
return reify().lastIndexOf(o);
}
public IPureListIterator listIterator() {
return new PureListIterator(reify().listIterator());
}
public IPureListIterator listIterator(int index) {
return new PureListIterator(reify().listIterator(index));
}
@SuppressWarnings("unchecked")
public K get(int index) {
return (K) PureCollections.nth(this, index);
}
public void add(int index, Object element) {
Pure4J.unsupported();
}
public boolean addAll(int index, Collection extends K> c) {
Pure4J.unsupported();
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy