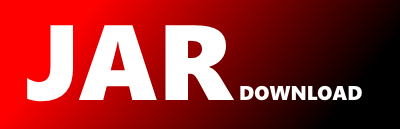
org.pure4j.collections.PersistentQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pure4j-core Show documentation
Show all versions of pure4j-core Show documentation
Pure Functional Programming Semantics For Java
/**
* Copyright (c) Rich Hickey. All rights reserved.
* The use and distribution terms for this software are covered by the
* Eclipse Public License 1.0 (http://opensource.org/licenses/eclipse-1.0.php)
* which can be found in the file epl-v10.html at the root of this distribution.
* By using this software in any fashion, you are agreeing to be bound by
* the terms of this license.
* You must not remove this notice, or any other, from this software.
**/
package org.pure4j.collections;
import java.util.Collection;
import java.util.Iterator;
import java.util.NoSuchElementException;
import org.pure4j.Pure4J;
import org.pure4j.annotations.immutable.IgnoreImmutableTypeCheck;
import org.pure4j.annotations.pure.Enforcement;
import org.pure4j.annotations.pure.Pure;
//import java.util.concurrent.ConcurrentLinkedQueue;
/**
* conses onto rear, peeks/pops from front See Okasaki's Batched Queues This
* differs in that it uses a PersistentVector as the rear, which is in-order, so
* no reversing or suspensions required for persistent use
*/
public class PersistentQueue implements IPersistentStack {
final private static PersistentQueue
© 2015 - 2024 Weber Informatics LLC | Privacy Policy