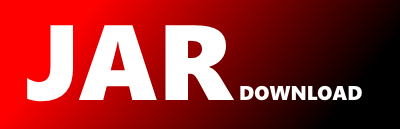
org.pure4j.collections.ToStringFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pure4j-core Show documentation
Show all versions of pure4j-core Show documentation
Pure Functional Programming Semantics For Java
package org.pure4j.collections;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import org.pure4j.annotations.pure.Pure;
public class ToStringFunctions {
/**
* Returns a string representation of this collection. The string
* representation consists of a list of the collection's elements in the
* order they are returned by its iterator, enclosed in square brackets
* ("[]"). Adjacent elements are separated by the characters
* ", " (comma and space). Elements are converted to strings as
* by {@link String#valueOf(Object)}.
*
* @param element type
* @param c the collection
* @return a string representation of this collection
*
*/
@Pure
public static String toString(Collection c) {
Iterator it = c.iterator();
if (! it.hasNext())
return "[]";
StringBuilder sb = new StringBuilder();
sb.append('[');
for (;;) {
E e = it.next();
sb.append(e == c ? "(this Collection)" : e);
if (! it.hasNext())
return sb.append(']').toString();
sb.append(',').append(' ');
}
}
/**
* Returns a string representation of this map. The string representation
* consists of a list of key-value mappings in the order returned by the
* map's entrySet view's iterator, enclosed in braces
* ("{}"). Adjacent mappings are separated by the characters
* ", " (comma and space). Each key-value mapping is rendered as
* the key followed by an equals sign ("=") followed by the
* associated value. Keys and values are converted to strings as by
* {@link String#valueOf(Object)}.
*
* @return a string representation of this map
* @param the key type
* @param the value type
* @param m the map to be rendered
*/
@Pure
public static String toString(Map m) {
Iterator> i = m.entrySet().iterator();
if (! i.hasNext())
return "{}";
StringBuilder sb = new StringBuilder();
sb.append('{');
for (;;) {
Entry e = i.next();
K key = e.getKey();
V value = e.getValue();
sb.append(key == m ? "(this Map)" : key);
sb.append('=');
sb.append(value == m ? "(this Map)" : value);
if (! i.hasNext())
return sb.append('}').toString();
sb.append(',').append(' ');
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy