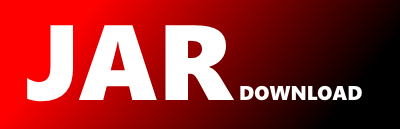
org.purejava.appindicator._GDriveIface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libappindicator-gtk3-java-full Show documentation
Show all versions of libappindicator-gtk3-java-full Show documentation
Java bindings for libappindicator-gtk3 in 100% pure Java
// Generated by jextract
package org.purejava.appindicator;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.SegmentAllocator;
import java.lang.invoke.VarHandle;
/**
* {@snippet :
* struct _GDriveIface {
* struct _GTypeInterface g_iface;
* void (*changed)(struct _GDrive*);
* void (*disconnected)(struct _GDrive*);
* void (*eject_button)(struct _GDrive*);
* char* (*get_name)(struct _GDrive*);
* struct _GIcon* (*get_icon)(struct _GDrive*);
* int (*has_volumes)(struct _GDrive*);
* struct _GList* (*get_volumes)(struct _GDrive*);
* int (*is_media_removable)(struct _GDrive*);
* int (*has_media)(struct _GDrive*);
* int (*is_media_check_automatic)(struct _GDrive*);
* int (*can_eject)(struct _GDrive*);
* int (*can_poll_for_media)(struct _GDrive*);
* void (*eject)(struct _GDrive*,enum GMountUnmountFlags,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* int (*eject_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* void (*poll_for_media)(struct _GDrive*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* int (*poll_for_media_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* char* (*get_identifier)(struct _GDrive*,char*);
* char** (*enumerate_identifiers)(struct _GDrive*);
* enum GDriveStartStopType (*get_start_stop_type)(struct _GDrive*);
* int (*can_start)(struct _GDrive*);
* int (*can_start_degraded)(struct _GDrive*);
* void (*start)(struct _GDrive*,enum GDriveStartFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* int (*start_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* int (*can_stop)(struct _GDrive*);
* void (*stop)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* int (*stop_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* void (*stop_button)(struct _GDrive*);
* void (*eject_with_operation)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* int (*eject_with_operation_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* char* (*get_sort_key)(struct _GDrive*);
* struct _GIcon* (*get_symbolic_icon)(struct _GDrive*);
* int (*is_removable)(struct _GDrive*);
* };
* }
*/
public class _GDriveIface {
public static MemoryLayout $LAYOUT() {
return constants$938.const$2;
}
public static MemorySegment g_iface$slice(MemorySegment seg) {
return seg.asSlice(0, 16);
}
/**
* {@snippet :
* void (*changed)(struct _GDrive*);
* }
*/
public interface changed {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(changed fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$938.const$3, fi, constants$13.const$1, scope);
}
static changed ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle changed$VH() {
return constants$938.const$4;
}
/**
* Getter for field:
* {@snippet :
* void (*changed)(struct _GDrive*);
* }
*/
public static MemorySegment changed$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$938.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*changed)(struct _GDrive*);
* }
*/
public static void changed$set(MemorySegment seg, MemorySegment x) {
constants$938.const$4.set(seg, x);
}
public static MemorySegment changed$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$938.const$4.get(seg.asSlice(index*sizeof()));
}
public static void changed$set(MemorySegment seg, long index, MemorySegment x) {
constants$938.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static changed changed(MemorySegment segment, Arena scope) {
return changed.ofAddress(changed$get(segment), scope);
}
/**
* {@snippet :
* void (*disconnected)(struct _GDrive*);
* }
*/
public interface disconnected {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(disconnected fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$938.const$5, fi, constants$13.const$1, scope);
}
static disconnected ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle disconnected$VH() {
return constants$939.const$0;
}
/**
* Getter for field:
* {@snippet :
* void (*disconnected)(struct _GDrive*);
* }
*/
public static MemorySegment disconnected$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$939.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*disconnected)(struct _GDrive*);
* }
*/
public static void disconnected$set(MemorySegment seg, MemorySegment x) {
constants$939.const$0.set(seg, x);
}
public static MemorySegment disconnected$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$939.const$0.get(seg.asSlice(index*sizeof()));
}
public static void disconnected$set(MemorySegment seg, long index, MemorySegment x) {
constants$939.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static disconnected disconnected(MemorySegment segment, Arena scope) {
return disconnected.ofAddress(disconnected$get(segment), scope);
}
/**
* {@snippet :
* void (*eject_button)(struct _GDrive*);
* }
*/
public interface eject_button {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(eject_button fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$939.const$1, fi, constants$13.const$1, scope);
}
static eject_button ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle eject_button$VH() {
return constants$939.const$2;
}
/**
* Getter for field:
* {@snippet :
* void (*eject_button)(struct _GDrive*);
* }
*/
public static MemorySegment eject_button$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$939.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*eject_button)(struct _GDrive*);
* }
*/
public static void eject_button$set(MemorySegment seg, MemorySegment x) {
constants$939.const$2.set(seg, x);
}
public static MemorySegment eject_button$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$939.const$2.get(seg.asSlice(index*sizeof()));
}
public static void eject_button$set(MemorySegment seg, long index, MemorySegment x) {
constants$939.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static eject_button eject_button(MemorySegment segment, Arena scope) {
return eject_button.ofAddress(eject_button$get(segment), scope);
}
/**
* {@snippet :
* char* (*get_name)(struct _GDrive*);
* }
*/
public interface get_name {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_name fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$939.const$3, fi, constants$5.const$2, scope);
}
static get_name ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_name$VH() {
return constants$939.const$4;
}
/**
* Getter for field:
* {@snippet :
* char* (*get_name)(struct _GDrive*);
* }
*/
public static MemorySegment get_name$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$939.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* char* (*get_name)(struct _GDrive*);
* }
*/
public static void get_name$set(MemorySegment seg, MemorySegment x) {
constants$939.const$4.set(seg, x);
}
public static MemorySegment get_name$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$939.const$4.get(seg.asSlice(index*sizeof()));
}
public static void get_name$set(MemorySegment seg, long index, MemorySegment x) {
constants$939.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static get_name get_name(MemorySegment segment, Arena scope) {
return get_name.ofAddress(get_name$get(segment), scope);
}
/**
* {@snippet :
* struct _GIcon* (*get_icon)(struct _GDrive*);
* }
*/
public interface get_icon {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_icon fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$939.const$5, fi, constants$5.const$2, scope);
}
static get_icon ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_icon$VH() {
return constants$940.const$0;
}
/**
* Getter for field:
* {@snippet :
* struct _GIcon* (*get_icon)(struct _GDrive*);
* }
*/
public static MemorySegment get_icon$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$940.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GIcon* (*get_icon)(struct _GDrive*);
* }
*/
public static void get_icon$set(MemorySegment seg, MemorySegment x) {
constants$940.const$0.set(seg, x);
}
public static MemorySegment get_icon$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$940.const$0.get(seg.asSlice(index*sizeof()));
}
public static void get_icon$set(MemorySegment seg, long index, MemorySegment x) {
constants$940.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static get_icon get_icon(MemorySegment segment, Arena scope) {
return get_icon.ofAddress(get_icon$get(segment), scope);
}
/**
* {@snippet :
* int (*has_volumes)(struct _GDrive*);
* }
*/
public interface has_volumes {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(has_volumes fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$940.const$1, fi, constants$10.const$5, scope);
}
static has_volumes ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle has_volumes$VH() {
return constants$940.const$2;
}
/**
* Getter for field:
* {@snippet :
* int (*has_volumes)(struct _GDrive*);
* }
*/
public static MemorySegment has_volumes$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$940.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*has_volumes)(struct _GDrive*);
* }
*/
public static void has_volumes$set(MemorySegment seg, MemorySegment x) {
constants$940.const$2.set(seg, x);
}
public static MemorySegment has_volumes$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$940.const$2.get(seg.asSlice(index*sizeof()));
}
public static void has_volumes$set(MemorySegment seg, long index, MemorySegment x) {
constants$940.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static has_volumes has_volumes(MemorySegment segment, Arena scope) {
return has_volumes.ofAddress(has_volumes$get(segment), scope);
}
/**
* {@snippet :
* struct _GList* (*get_volumes)(struct _GDrive*);
* }
*/
public interface get_volumes {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_volumes fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$940.const$3, fi, constants$5.const$2, scope);
}
static get_volumes ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_volumes$VH() {
return constants$940.const$4;
}
/**
* Getter for field:
* {@snippet :
* struct _GList* (*get_volumes)(struct _GDrive*);
* }
*/
public static MemorySegment get_volumes$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$940.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GList* (*get_volumes)(struct _GDrive*);
* }
*/
public static void get_volumes$set(MemorySegment seg, MemorySegment x) {
constants$940.const$4.set(seg, x);
}
public static MemorySegment get_volumes$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$940.const$4.get(seg.asSlice(index*sizeof()));
}
public static void get_volumes$set(MemorySegment seg, long index, MemorySegment x) {
constants$940.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static get_volumes get_volumes(MemorySegment segment, Arena scope) {
return get_volumes.ofAddress(get_volumes$get(segment), scope);
}
/**
* {@snippet :
* int (*is_media_removable)(struct _GDrive*);
* }
*/
public interface is_media_removable {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(is_media_removable fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$940.const$5, fi, constants$10.const$5, scope);
}
static is_media_removable ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle is_media_removable$VH() {
return constants$941.const$0;
}
/**
* Getter for field:
* {@snippet :
* int (*is_media_removable)(struct _GDrive*);
* }
*/
public static MemorySegment is_media_removable$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$941.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*is_media_removable)(struct _GDrive*);
* }
*/
public static void is_media_removable$set(MemorySegment seg, MemorySegment x) {
constants$941.const$0.set(seg, x);
}
public static MemorySegment is_media_removable$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$941.const$0.get(seg.asSlice(index*sizeof()));
}
public static void is_media_removable$set(MemorySegment seg, long index, MemorySegment x) {
constants$941.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static is_media_removable is_media_removable(MemorySegment segment, Arena scope) {
return is_media_removable.ofAddress(is_media_removable$get(segment), scope);
}
/**
* {@snippet :
* int (*has_media)(struct _GDrive*);
* }
*/
public interface has_media {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(has_media fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$941.const$1, fi, constants$10.const$5, scope);
}
static has_media ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle has_media$VH() {
return constants$941.const$2;
}
/**
* Getter for field:
* {@snippet :
* int (*has_media)(struct _GDrive*);
* }
*/
public static MemorySegment has_media$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$941.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*has_media)(struct _GDrive*);
* }
*/
public static void has_media$set(MemorySegment seg, MemorySegment x) {
constants$941.const$2.set(seg, x);
}
public static MemorySegment has_media$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$941.const$2.get(seg.asSlice(index*sizeof()));
}
public static void has_media$set(MemorySegment seg, long index, MemorySegment x) {
constants$941.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static has_media has_media(MemorySegment segment, Arena scope) {
return has_media.ofAddress(has_media$get(segment), scope);
}
/**
* {@snippet :
* int (*is_media_check_automatic)(struct _GDrive*);
* }
*/
public interface is_media_check_automatic {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(is_media_check_automatic fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$941.const$3, fi, constants$10.const$5, scope);
}
static is_media_check_automatic ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle is_media_check_automatic$VH() {
return constants$941.const$4;
}
/**
* Getter for field:
* {@snippet :
* int (*is_media_check_automatic)(struct _GDrive*);
* }
*/
public static MemorySegment is_media_check_automatic$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$941.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*is_media_check_automatic)(struct _GDrive*);
* }
*/
public static void is_media_check_automatic$set(MemorySegment seg, MemorySegment x) {
constants$941.const$4.set(seg, x);
}
public static MemorySegment is_media_check_automatic$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$941.const$4.get(seg.asSlice(index*sizeof()));
}
public static void is_media_check_automatic$set(MemorySegment seg, long index, MemorySegment x) {
constants$941.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static is_media_check_automatic is_media_check_automatic(MemorySegment segment, Arena scope) {
return is_media_check_automatic.ofAddress(is_media_check_automatic$get(segment), scope);
}
/**
* {@snippet :
* int (*can_eject)(struct _GDrive*);
* }
*/
public interface can_eject {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(can_eject fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$941.const$5, fi, constants$10.const$5, scope);
}
static can_eject ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle can_eject$VH() {
return constants$942.const$0;
}
/**
* Getter for field:
* {@snippet :
* int (*can_eject)(struct _GDrive*);
* }
*/
public static MemorySegment can_eject$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$942.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*can_eject)(struct _GDrive*);
* }
*/
public static void can_eject$set(MemorySegment seg, MemorySegment x) {
constants$942.const$0.set(seg, x);
}
public static MemorySegment can_eject$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$942.const$0.get(seg.asSlice(index*sizeof()));
}
public static void can_eject$set(MemorySegment seg, long index, MemorySegment x) {
constants$942.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static can_eject can_eject(MemorySegment segment, Arena scope) {
return can_eject.ofAddress(can_eject$get(segment), scope);
}
/**
* {@snippet :
* int (*can_poll_for_media)(struct _GDrive*);
* }
*/
public interface can_poll_for_media {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(can_poll_for_media fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$942.const$1, fi, constants$10.const$5, scope);
}
static can_poll_for_media ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle can_poll_for_media$VH() {
return constants$942.const$2;
}
/**
* Getter for field:
* {@snippet :
* int (*can_poll_for_media)(struct _GDrive*);
* }
*/
public static MemorySegment can_poll_for_media$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$942.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*can_poll_for_media)(struct _GDrive*);
* }
*/
public static void can_poll_for_media$set(MemorySegment seg, MemorySegment x) {
constants$942.const$2.set(seg, x);
}
public static MemorySegment can_poll_for_media$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$942.const$2.get(seg.asSlice(index*sizeof()));
}
public static void can_poll_for_media$set(MemorySegment seg, long index, MemorySegment x) {
constants$942.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static can_poll_for_media can_poll_for_media(MemorySegment segment, Arena scope) {
return can_poll_for_media.ofAddress(can_poll_for_media$get(segment), scope);
}
/**
* {@snippet :
* void (*eject)(struct _GDrive*,enum GMountUnmountFlags,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public interface eject {
void apply(java.lang.foreign.MemorySegment _x0, int _x1, java.lang.foreign.MemorySegment _x2, java.lang.foreign.MemorySegment _x3, java.lang.foreign.MemorySegment _x4);
static MemorySegment allocate(eject fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$942.const$3, fi, constants$281.const$5, scope);
}
static eject ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, java.lang.foreign.MemorySegment __x2, java.lang.foreign.MemorySegment __x3, java.lang.foreign.MemorySegment __x4) -> {
try {
constants$754.const$2.invokeExact(symbol, __x0, __x1, __x2, __x3, __x4);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle eject$VH() {
return constants$942.const$4;
}
/**
* Getter for field:
* {@snippet :
* void (*eject)(struct _GDrive*,enum GMountUnmountFlags,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static MemorySegment eject$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$942.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*eject)(struct _GDrive*,enum GMountUnmountFlags,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static void eject$set(MemorySegment seg, MemorySegment x) {
constants$942.const$4.set(seg, x);
}
public static MemorySegment eject$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$942.const$4.get(seg.asSlice(index*sizeof()));
}
public static void eject$set(MemorySegment seg, long index, MemorySegment x) {
constants$942.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static eject eject(MemorySegment segment, Arena scope) {
return eject.ofAddress(eject$get(segment), scope);
}
/**
* {@snippet :
* int (*eject_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public interface eject_finish {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(eject_finish fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$942.const$5, fi, constants$12.const$2, scope);
}
static eject_finish ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle eject_finish$VH() {
return constants$943.const$0;
}
/**
* Getter for field:
* {@snippet :
* int (*eject_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static MemorySegment eject_finish$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$943.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*eject_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static void eject_finish$set(MemorySegment seg, MemorySegment x) {
constants$943.const$0.set(seg, x);
}
public static MemorySegment eject_finish$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$943.const$0.get(seg.asSlice(index*sizeof()));
}
public static void eject_finish$set(MemorySegment seg, long index, MemorySegment x) {
constants$943.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static eject_finish eject_finish(MemorySegment segment, Arena scope) {
return eject_finish.ofAddress(eject_finish$get(segment), scope);
}
/**
* {@snippet :
* void (*poll_for_media)(struct _GDrive*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public interface poll_for_media {
void apply(java.lang.foreign.MemorySegment model, java.lang.foreign.MemorySegment path, java.lang.foreign.MemorySegment iter, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(poll_for_media fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$943.const$1, fi, constants$42.const$1, scope);
}
static poll_for_media ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _model, java.lang.foreign.MemorySegment _path, java.lang.foreign.MemorySegment _iter, java.lang.foreign.MemorySegment _data) -> {
try {
constants$259.const$4.invokeExact(symbol, _model, _path, _iter, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle poll_for_media$VH() {
return constants$943.const$2;
}
/**
* Getter for field:
* {@snippet :
* void (*poll_for_media)(struct _GDrive*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static MemorySegment poll_for_media$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$943.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*poll_for_media)(struct _GDrive*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static void poll_for_media$set(MemorySegment seg, MemorySegment x) {
constants$943.const$2.set(seg, x);
}
public static MemorySegment poll_for_media$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$943.const$2.get(seg.asSlice(index*sizeof()));
}
public static void poll_for_media$set(MemorySegment seg, long index, MemorySegment x) {
constants$943.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static poll_for_media poll_for_media(MemorySegment segment, Arena scope) {
return poll_for_media.ofAddress(poll_for_media$get(segment), scope);
}
/**
* {@snippet :
* int (*poll_for_media_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public interface poll_for_media_finish {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(poll_for_media_finish fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$943.const$3, fi, constants$12.const$2, scope);
}
static poll_for_media_finish ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle poll_for_media_finish$VH() {
return constants$943.const$4;
}
/**
* Getter for field:
* {@snippet :
* int (*poll_for_media_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static MemorySegment poll_for_media_finish$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$943.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*poll_for_media_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static void poll_for_media_finish$set(MemorySegment seg, MemorySegment x) {
constants$943.const$4.set(seg, x);
}
public static MemorySegment poll_for_media_finish$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$943.const$4.get(seg.asSlice(index*sizeof()));
}
public static void poll_for_media_finish$set(MemorySegment seg, long index, MemorySegment x) {
constants$943.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static poll_for_media_finish poll_for_media_finish(MemorySegment segment, Arena scope) {
return poll_for_media_finish.ofAddress(poll_for_media_finish$get(segment), scope);
}
/**
* {@snippet :
* char* (*get_identifier)(struct _GDrive*,char*);
* }
*/
public interface get_identifier {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment path, java.lang.foreign.MemorySegment func_data);
static MemorySegment allocate(get_identifier fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$943.const$5, fi, constants$5.const$5, scope);
}
static get_identifier ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _path, java.lang.foreign.MemorySegment _func_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$15.const$1.invokeExact(symbol, _path, _func_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_identifier$VH() {
return constants$944.const$0;
}
/**
* Getter for field:
* {@snippet :
* char* (*get_identifier)(struct _GDrive*,char*);
* }
*/
public static MemorySegment get_identifier$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$944.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* char* (*get_identifier)(struct _GDrive*,char*);
* }
*/
public static void get_identifier$set(MemorySegment seg, MemorySegment x) {
constants$944.const$0.set(seg, x);
}
public static MemorySegment get_identifier$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$944.const$0.get(seg.asSlice(index*sizeof()));
}
public static void get_identifier$set(MemorySegment seg, long index, MemorySegment x) {
constants$944.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static get_identifier get_identifier(MemorySegment segment, Arena scope) {
return get_identifier.ofAddress(get_identifier$get(segment), scope);
}
/**
* {@snippet :
* char** (*enumerate_identifiers)(struct _GDrive*);
* }
*/
public interface enumerate_identifiers {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(enumerate_identifiers fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$944.const$1, fi, constants$5.const$2, scope);
}
static enumerate_identifiers ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle enumerate_identifiers$VH() {
return constants$944.const$2;
}
/**
* Getter for field:
* {@snippet :
* char** (*enumerate_identifiers)(struct _GDrive*);
* }
*/
public static MemorySegment enumerate_identifiers$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$944.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* char** (*enumerate_identifiers)(struct _GDrive*);
* }
*/
public static void enumerate_identifiers$set(MemorySegment seg, MemorySegment x) {
constants$944.const$2.set(seg, x);
}
public static MemorySegment enumerate_identifiers$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$944.const$2.get(seg.asSlice(index*sizeof()));
}
public static void enumerate_identifiers$set(MemorySegment seg, long index, MemorySegment x) {
constants$944.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static enumerate_identifiers enumerate_identifiers(MemorySegment segment, Arena scope) {
return enumerate_identifiers.ofAddress(enumerate_identifiers$get(segment), scope);
}
/**
* {@snippet :
* enum GDriveStartStopType (*get_start_stop_type)(struct _GDrive*);
* }
*/
public interface get_start_stop_type {
int apply(java.lang.foreign.MemorySegment _x0);
static MemorySegment allocate(get_start_stop_type fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$944.const$3, fi, constants$10.const$5, scope);
}
static get_start_stop_type ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, __x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_start_stop_type$VH() {
return constants$944.const$4;
}
/**
* Getter for field:
* {@snippet :
* enum GDriveStartStopType (*get_start_stop_type)(struct _GDrive*);
* }
*/
public static MemorySegment get_start_stop_type$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$944.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* enum GDriveStartStopType (*get_start_stop_type)(struct _GDrive*);
* }
*/
public static void get_start_stop_type$set(MemorySegment seg, MemorySegment x) {
constants$944.const$4.set(seg, x);
}
public static MemorySegment get_start_stop_type$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$944.const$4.get(seg.asSlice(index*sizeof()));
}
public static void get_start_stop_type$set(MemorySegment seg, long index, MemorySegment x) {
constants$944.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static get_start_stop_type get_start_stop_type(MemorySegment segment, Arena scope) {
return get_start_stop_type.ofAddress(get_start_stop_type$get(segment), scope);
}
/**
* {@snippet :
* int (*can_start)(struct _GDrive*);
* }
*/
public interface can_start {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(can_start fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$944.const$5, fi, constants$10.const$5, scope);
}
static can_start ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle can_start$VH() {
return constants$945.const$0;
}
/**
* Getter for field:
* {@snippet :
* int (*can_start)(struct _GDrive*);
* }
*/
public static MemorySegment can_start$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$945.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*can_start)(struct _GDrive*);
* }
*/
public static void can_start$set(MemorySegment seg, MemorySegment x) {
constants$945.const$0.set(seg, x);
}
public static MemorySegment can_start$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$945.const$0.get(seg.asSlice(index*sizeof()));
}
public static void can_start$set(MemorySegment seg, long index, MemorySegment x) {
constants$945.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static can_start can_start(MemorySegment segment, Arena scope) {
return can_start.ofAddress(can_start$get(segment), scope);
}
/**
* {@snippet :
* int (*can_start_degraded)(struct _GDrive*);
* }
*/
public interface can_start_degraded {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(can_start_degraded fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$945.const$1, fi, constants$10.const$5, scope);
}
static can_start_degraded ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle can_start_degraded$VH() {
return constants$945.const$2;
}
/**
* Getter for field:
* {@snippet :
* int (*can_start_degraded)(struct _GDrive*);
* }
*/
public static MemorySegment can_start_degraded$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$945.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*can_start_degraded)(struct _GDrive*);
* }
*/
public static void can_start_degraded$set(MemorySegment seg, MemorySegment x) {
constants$945.const$2.set(seg, x);
}
public static MemorySegment can_start_degraded$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$945.const$2.get(seg.asSlice(index*sizeof()));
}
public static void can_start_degraded$set(MemorySegment seg, long index, MemorySegment x) {
constants$945.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static can_start_degraded can_start_degraded(MemorySegment segment, Arena scope) {
return can_start_degraded.ofAddress(can_start_degraded$get(segment), scope);
}
/**
* {@snippet :
* void (*start)(struct _GDrive*,enum GDriveStartFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public interface start {
void apply(java.lang.foreign.MemorySegment _x0, int _x1, java.lang.foreign.MemorySegment _x2, java.lang.foreign.MemorySegment _x3, java.lang.foreign.MemorySegment _x4, java.lang.foreign.MemorySegment _x5);
static MemorySegment allocate(start fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$945.const$3, fi, constants$380.const$0, scope);
}
static start ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, java.lang.foreign.MemorySegment __x2, java.lang.foreign.MemorySegment __x3, java.lang.foreign.MemorySegment __x4, java.lang.foreign.MemorySegment __x5) -> {
try {
constants$945.const$4.invokeExact(symbol, __x0, __x1, __x2, __x3, __x4, __x5);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle start$VH() {
return constants$945.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*start)(struct _GDrive*,enum GDriveStartFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static MemorySegment start$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$945.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*start)(struct _GDrive*,enum GDriveStartFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static void start$set(MemorySegment seg, MemorySegment x) {
constants$945.const$5.set(seg, x);
}
public static MemorySegment start$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$945.const$5.get(seg.asSlice(index*sizeof()));
}
public static void start$set(MemorySegment seg, long index, MemorySegment x) {
constants$945.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static start start(MemorySegment segment, Arena scope) {
return start.ofAddress(start$get(segment), scope);
}
/**
* {@snippet :
* int (*start_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public interface start_finish {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(start_finish fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$946.const$0, fi, constants$12.const$2, scope);
}
static start_finish ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle start_finish$VH() {
return constants$946.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*start_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static MemorySegment start_finish$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$946.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*start_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static void start_finish$set(MemorySegment seg, MemorySegment x) {
constants$946.const$1.set(seg, x);
}
public static MemorySegment start_finish$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$946.const$1.get(seg.asSlice(index*sizeof()));
}
public static void start_finish$set(MemorySegment seg, long index, MemorySegment x) {
constants$946.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static start_finish start_finish(MemorySegment segment, Arena scope) {
return start_finish.ofAddress(start_finish$get(segment), scope);
}
/**
* {@snippet :
* int (*can_stop)(struct _GDrive*);
* }
*/
public interface can_stop {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(can_stop fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$946.const$2, fi, constants$10.const$5, scope);
}
static can_stop ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle can_stop$VH() {
return constants$946.const$3;
}
/**
* Getter for field:
* {@snippet :
* int (*can_stop)(struct _GDrive*);
* }
*/
public static MemorySegment can_stop$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$946.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*can_stop)(struct _GDrive*);
* }
*/
public static void can_stop$set(MemorySegment seg, MemorySegment x) {
constants$946.const$3.set(seg, x);
}
public static MemorySegment can_stop$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$946.const$3.get(seg.asSlice(index*sizeof()));
}
public static void can_stop$set(MemorySegment seg, long index, MemorySegment x) {
constants$946.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static can_stop can_stop(MemorySegment segment, Arena scope) {
return can_stop.ofAddress(can_stop$get(segment), scope);
}
/**
* {@snippet :
* void (*stop)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public interface stop {
void apply(java.lang.foreign.MemorySegment _x0, int _x1, java.lang.foreign.MemorySegment _x2, java.lang.foreign.MemorySegment _x3, java.lang.foreign.MemorySegment _x4, java.lang.foreign.MemorySegment _x5);
static MemorySegment allocate(stop fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$946.const$4, fi, constants$380.const$0, scope);
}
static stop ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, java.lang.foreign.MemorySegment __x2, java.lang.foreign.MemorySegment __x3, java.lang.foreign.MemorySegment __x4, java.lang.foreign.MemorySegment __x5) -> {
try {
constants$945.const$4.invokeExact(symbol, __x0, __x1, __x2, __x3, __x4, __x5);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle stop$VH() {
return constants$946.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*stop)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static MemorySegment stop$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$946.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*stop)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static void stop$set(MemorySegment seg, MemorySegment x) {
constants$946.const$5.set(seg, x);
}
public static MemorySegment stop$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$946.const$5.get(seg.asSlice(index*sizeof()));
}
public static void stop$set(MemorySegment seg, long index, MemorySegment x) {
constants$946.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static stop stop(MemorySegment segment, Arena scope) {
return stop.ofAddress(stop$get(segment), scope);
}
/**
* {@snippet :
* int (*stop_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public interface stop_finish {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(stop_finish fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$947.const$0, fi, constants$12.const$2, scope);
}
static stop_finish ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle stop_finish$VH() {
return constants$947.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*stop_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static MemorySegment stop_finish$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$947.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*stop_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static void stop_finish$set(MemorySegment seg, MemorySegment x) {
constants$947.const$1.set(seg, x);
}
public static MemorySegment stop_finish$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$947.const$1.get(seg.asSlice(index*sizeof()));
}
public static void stop_finish$set(MemorySegment seg, long index, MemorySegment x) {
constants$947.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static stop_finish stop_finish(MemorySegment segment, Arena scope) {
return stop_finish.ofAddress(stop_finish$get(segment), scope);
}
/**
* {@snippet :
* void (*stop_button)(struct _GDrive*);
* }
*/
public interface stop_button {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(stop_button fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$947.const$2, fi, constants$13.const$1, scope);
}
static stop_button ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle stop_button$VH() {
return constants$947.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*stop_button)(struct _GDrive*);
* }
*/
public static MemorySegment stop_button$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$947.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*stop_button)(struct _GDrive*);
* }
*/
public static void stop_button$set(MemorySegment seg, MemorySegment x) {
constants$947.const$3.set(seg, x);
}
public static MemorySegment stop_button$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$947.const$3.get(seg.asSlice(index*sizeof()));
}
public static void stop_button$set(MemorySegment seg, long index, MemorySegment x) {
constants$947.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static stop_button stop_button(MemorySegment segment, Arena scope) {
return stop_button.ofAddress(stop_button$get(segment), scope);
}
/**
* {@snippet :
* void (*eject_with_operation)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public interface eject_with_operation {
void apply(java.lang.foreign.MemorySegment _x0, int _x1, java.lang.foreign.MemorySegment _x2, java.lang.foreign.MemorySegment _x3, java.lang.foreign.MemorySegment _x4, java.lang.foreign.MemorySegment _x5);
static MemorySegment allocate(eject_with_operation fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$947.const$4, fi, constants$380.const$0, scope);
}
static eject_with_operation ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, java.lang.foreign.MemorySegment __x2, java.lang.foreign.MemorySegment __x3, java.lang.foreign.MemorySegment __x4, java.lang.foreign.MemorySegment __x5) -> {
try {
constants$945.const$4.invokeExact(symbol, __x0, __x1, __x2, __x3, __x4, __x5);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle eject_with_operation$VH() {
return constants$947.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*eject_with_operation)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static MemorySegment eject_with_operation$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$947.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*eject_with_operation)(struct _GDrive*,enum GMountUnmountFlags,struct _GMountOperation*,struct _GCancellable*,void (*)(struct _GObject*,struct _GAsyncResult*,void*),void*);
* }
*/
public static void eject_with_operation$set(MemorySegment seg, MemorySegment x) {
constants$947.const$5.set(seg, x);
}
public static MemorySegment eject_with_operation$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$947.const$5.get(seg.asSlice(index*sizeof()));
}
public static void eject_with_operation$set(MemorySegment seg, long index, MemorySegment x) {
constants$947.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static eject_with_operation eject_with_operation(MemorySegment segment, Arena scope) {
return eject_with_operation.ofAddress(eject_with_operation$get(segment), scope);
}
/**
* {@snippet :
* int (*eject_with_operation_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public interface eject_with_operation_finish {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(eject_with_operation_finish fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$948.const$0, fi, constants$12.const$2, scope);
}
static eject_with_operation_finish ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle eject_with_operation_finish$VH() {
return constants$948.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*eject_with_operation_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static MemorySegment eject_with_operation_finish$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$948.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*eject_with_operation_finish)(struct _GDrive*,struct _GAsyncResult*,struct _GError**);
* }
*/
public static void eject_with_operation_finish$set(MemorySegment seg, MemorySegment x) {
constants$948.const$1.set(seg, x);
}
public static MemorySegment eject_with_operation_finish$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$948.const$1.get(seg.asSlice(index*sizeof()));
}
public static void eject_with_operation_finish$set(MemorySegment seg, long index, MemorySegment x) {
constants$948.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static eject_with_operation_finish eject_with_operation_finish(MemorySegment segment, Arena scope) {
return eject_with_operation_finish.ofAddress(eject_with_operation_finish$get(segment), scope);
}
/**
* {@snippet :
* char* (*get_sort_key)(struct _GDrive*);
* }
*/
public interface get_sort_key {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_sort_key fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$948.const$2, fi, constants$5.const$2, scope);
}
static get_sort_key ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_sort_key$VH() {
return constants$948.const$3;
}
/**
* Getter for field:
* {@snippet :
* char* (*get_sort_key)(struct _GDrive*);
* }
*/
public static MemorySegment get_sort_key$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$948.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* char* (*get_sort_key)(struct _GDrive*);
* }
*/
public static void get_sort_key$set(MemorySegment seg, MemorySegment x) {
constants$948.const$3.set(seg, x);
}
public static MemorySegment get_sort_key$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$948.const$3.get(seg.asSlice(index*sizeof()));
}
public static void get_sort_key$set(MemorySegment seg, long index, MemorySegment x) {
constants$948.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static get_sort_key get_sort_key(MemorySegment segment, Arena scope) {
return get_sort_key.ofAddress(get_sort_key$get(segment), scope);
}
/**
* {@snippet :
* struct _GIcon* (*get_symbolic_icon)(struct _GDrive*);
* }
*/
public interface get_symbolic_icon {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_symbolic_icon fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$948.const$4, fi, constants$5.const$2, scope);
}
static get_symbolic_icon ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_symbolic_icon$VH() {
return constants$948.const$5;
}
/**
* Getter for field:
* {@snippet :
* struct _GIcon* (*get_symbolic_icon)(struct _GDrive*);
* }
*/
public static MemorySegment get_symbolic_icon$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$948.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GIcon* (*get_symbolic_icon)(struct _GDrive*);
* }
*/
public static void get_symbolic_icon$set(MemorySegment seg, MemorySegment x) {
constants$948.const$5.set(seg, x);
}
public static MemorySegment get_symbolic_icon$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$948.const$5.get(seg.asSlice(index*sizeof()));
}
public static void get_symbolic_icon$set(MemorySegment seg, long index, MemorySegment x) {
constants$948.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static get_symbolic_icon get_symbolic_icon(MemorySegment segment, Arena scope) {
return get_symbolic_icon.ofAddress(get_symbolic_icon$get(segment), scope);
}
/**
* {@snippet :
* int (*is_removable)(struct _GDrive*);
* }
*/
public interface is_removable {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(is_removable fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$949.const$0, fi, constants$10.const$5, scope);
}
static is_removable ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle is_removable$VH() {
return constants$949.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*is_removable)(struct _GDrive*);
* }
*/
public static MemorySegment is_removable$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$949.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*is_removable)(struct _GDrive*);
* }
*/
public static void is_removable$set(MemorySegment seg, MemorySegment x) {
constants$949.const$1.set(seg, x);
}
public static MemorySegment is_removable$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$949.const$1.get(seg.asSlice(index*sizeof()));
}
public static void is_removable$set(MemorySegment seg, long index, MemorySegment x) {
constants$949.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static is_removable is_removable(MemorySegment segment, Arena scope) {
return is_removable.ofAddress(is_removable$get(segment), scope);
}
public static long sizeof() { return $LAYOUT().byteSize(); }
public static MemorySegment allocate(SegmentAllocator allocator) { return allocator.allocate($LAYOUT()); }
public static MemorySegment allocateArray(long len, SegmentAllocator allocator) {
return allocator.allocate(MemoryLayout.sequenceLayout(len, $LAYOUT()));
}
public static MemorySegment ofAddress(MemorySegment addr, Arena scope) { return RuntimeHelper.asArray(addr, $LAYOUT(), 1, scope); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy