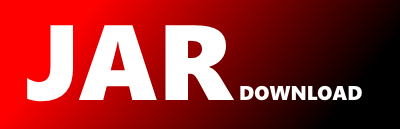
org.purejava.appindicator._GObjectClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libappindicator-gtk3-java-full Show documentation
Show all versions of libappindicator-gtk3-java-full Show documentation
Java bindings for libappindicator-gtk3 in 100% pure Java
// Generated by jextract
package org.purejava.appindicator;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.SegmentAllocator;
import java.lang.invoke.VarHandle;
/**
* {@snippet :
* struct _GObjectClass {
* struct _GTypeClass g_type_class;
* struct _GSList* construct_properties;
* struct _GObject* (*constructor)(unsigned long,unsigned int,struct _GObjectConstructParam*);
* void (*set_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* void (*get_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* void (*dispose)(struct _GObject*);
* void (*finalize)(struct _GObject*);
* void (*dispatch_properties_changed)(struct _GObject*,unsigned int,struct _GParamSpec**);
* void (*notify)(struct _GObject*,struct _GParamSpec*);
* void (*constructed)(struct _GObject*);
* unsigned long flags;
* unsigned long n_construct_properties;
* void* pspecs;
* unsigned long n_pspecs;
* void* pdummy[3];
* };
* }
*/
public class _GObjectClass {
public static MemoryLayout $LAYOUT() {
return constants$622.const$5;
}
public static MemorySegment g_type_class$slice(MemorySegment seg) {
return seg.asSlice(0, 8);
}
public static VarHandle construct_properties$VH() {
return constants$623.const$0;
}
/**
* Getter for field:
* {@snippet :
* struct _GSList* construct_properties;
* }
*/
public static MemorySegment construct_properties$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$623.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GSList* construct_properties;
* }
*/
public static void construct_properties$set(MemorySegment seg, MemorySegment x) {
constants$623.const$0.set(seg, x);
}
public static MemorySegment construct_properties$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$623.const$0.get(seg.asSlice(index*sizeof()));
}
public static void construct_properties$set(MemorySegment seg, long index, MemorySegment x) {
constants$623.const$0.set(seg.asSlice(index*sizeof()), x);
}
/**
* {@snippet :
* struct _GObject* (*constructor)(unsigned long,unsigned int,struct _GObjectConstructParam*);
* }
*/
public interface constructor {
java.lang.foreign.MemorySegment apply(long _x0, int _x1, java.lang.foreign.MemorySegment _x2);
static MemorySegment allocate(constructor fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$623.const$2, fi, constants$623.const$1, scope);
}
static constructor ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (long __x0, int __x1, java.lang.foreign.MemorySegment __x2) -> {
try {
return (java.lang.foreign.MemorySegment)constants$623.const$3.invokeExact(symbol, __x0, __x1, __x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle constructor$VH() {
return constants$623.const$4;
}
/**
* Getter for field:
* {@snippet :
* struct _GObject* (*constructor)(unsigned long,unsigned int,struct _GObjectConstructParam*);
* }
*/
public static MemorySegment constructor$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$623.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GObject* (*constructor)(unsigned long,unsigned int,struct _GObjectConstructParam*);
* }
*/
public static void constructor$set(MemorySegment seg, MemorySegment x) {
constants$623.const$4.set(seg, x);
}
public static MemorySegment constructor$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$623.const$4.get(seg.asSlice(index*sizeof()));
}
public static void constructor$set(MemorySegment seg, long index, MemorySegment x) {
constants$623.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static constructor constructor(MemorySegment segment, Arena scope) {
return constructor.ofAddress(constructor$get(segment), scope);
}
/**
* {@snippet :
* void (*set_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public interface set_property {
void apply(java.lang.foreign.MemorySegment object, int property_id, java.lang.foreign.MemorySegment value, java.lang.foreign.MemorySegment pspec);
static MemorySegment allocate(set_property fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$623.const$5, fi, constants$179.const$1, scope);
}
static set_property ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _object, int _property_id, java.lang.foreign.MemorySegment _value, java.lang.foreign.MemorySegment _pspec) -> {
try {
constants$372.const$3.invokeExact(symbol, _object, _property_id, _value, _pspec);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle set_property$VH() {
return constants$624.const$0;
}
/**
* Getter for field:
* {@snippet :
* void (*set_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public static MemorySegment set_property$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$624.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*set_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public static void set_property$set(MemorySegment seg, MemorySegment x) {
constants$624.const$0.set(seg, x);
}
public static MemorySegment set_property$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$624.const$0.get(seg.asSlice(index*sizeof()));
}
public static void set_property$set(MemorySegment seg, long index, MemorySegment x) {
constants$624.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static set_property set_property(MemorySegment segment, Arena scope) {
return set_property.ofAddress(set_property$get(segment), scope);
}
/**
* {@snippet :
* void (*get_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public interface get_property {
void apply(java.lang.foreign.MemorySegment object, int property_id, java.lang.foreign.MemorySegment value, java.lang.foreign.MemorySegment pspec);
static MemorySegment allocate(get_property fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$624.const$1, fi, constants$179.const$1, scope);
}
static get_property ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _object, int _property_id, java.lang.foreign.MemorySegment _value, java.lang.foreign.MemorySegment _pspec) -> {
try {
constants$372.const$3.invokeExact(symbol, _object, _property_id, _value, _pspec);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_property$VH() {
return constants$624.const$2;
}
/**
* Getter for field:
* {@snippet :
* void (*get_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public static MemorySegment get_property$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$624.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*get_property)(struct _GObject*,unsigned int,struct _GValue*,struct _GParamSpec*);
* }
*/
public static void get_property$set(MemorySegment seg, MemorySegment x) {
constants$624.const$2.set(seg, x);
}
public static MemorySegment get_property$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$624.const$2.get(seg.asSlice(index*sizeof()));
}
public static void get_property$set(MemorySegment seg, long index, MemorySegment x) {
constants$624.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static get_property get_property(MemorySegment segment, Arena scope) {
return get_property.ofAddress(get_property$get(segment), scope);
}
/**
* {@snippet :
* void (*dispose)(struct _GObject*);
* }
*/
public interface dispose {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(dispose fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$624.const$3, fi, constants$13.const$1, scope);
}
static dispose ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle dispose$VH() {
return constants$624.const$4;
}
/**
* Getter for field:
* {@snippet :
* void (*dispose)(struct _GObject*);
* }
*/
public static MemorySegment dispose$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$624.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*dispose)(struct _GObject*);
* }
*/
public static void dispose$set(MemorySegment seg, MemorySegment x) {
constants$624.const$4.set(seg, x);
}
public static MemorySegment dispose$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$624.const$4.get(seg.asSlice(index*sizeof()));
}
public static void dispose$set(MemorySegment seg, long index, MemorySegment x) {
constants$624.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static dispose dispose(MemorySegment segment, Arena scope) {
return dispose.ofAddress(dispose$get(segment), scope);
}
/**
* {@snippet :
* void (*finalize)(struct _GObject*);
* }
*/
public interface finalize {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(finalize fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$624.const$5, fi, constants$13.const$1, scope);
}
static finalize ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle finalize$VH() {
return constants$625.const$0;
}
/**
* Getter for field:
* {@snippet :
* void (*finalize)(struct _GObject*);
* }
*/
public static MemorySegment finalize$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$625.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*finalize)(struct _GObject*);
* }
*/
public static void finalize$set(MemorySegment seg, MemorySegment x) {
constants$625.const$0.set(seg, x);
}
public static MemorySegment finalize$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$625.const$0.get(seg.asSlice(index*sizeof()));
}
public static void finalize$set(MemorySegment seg, long index, MemorySegment x) {
constants$625.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static finalize finalize(MemorySegment segment, Arena scope) {
return finalize.ofAddress(finalize$get(segment), scope);
}
/**
* {@snippet :
* void (*dispatch_properties_changed)(struct _GObject*,unsigned int,struct _GParamSpec**);
* }
*/
public interface dispatch_properties_changed {
void apply(java.lang.foreign.MemorySegment _x0, int _x1, java.lang.foreign.MemorySegment _x2);
static MemorySegment allocate(dispatch_properties_changed fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$625.const$1, fi, constants$42.const$4, scope);
}
static dispatch_properties_changed ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, java.lang.foreign.MemorySegment __x2) -> {
try {
constants$625.const$2.invokeExact(symbol, __x0, __x1, __x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle dispatch_properties_changed$VH() {
return constants$625.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*dispatch_properties_changed)(struct _GObject*,unsigned int,struct _GParamSpec**);
* }
*/
public static MemorySegment dispatch_properties_changed$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$625.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*dispatch_properties_changed)(struct _GObject*,unsigned int,struct _GParamSpec**);
* }
*/
public static void dispatch_properties_changed$set(MemorySegment seg, MemorySegment x) {
constants$625.const$3.set(seg, x);
}
public static MemorySegment dispatch_properties_changed$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$625.const$3.get(seg.asSlice(index*sizeof()));
}
public static void dispatch_properties_changed$set(MemorySegment seg, long index, MemorySegment x) {
constants$625.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static dispatch_properties_changed dispatch_properties_changed(MemorySegment segment, Arena scope) {
return dispatch_properties_changed.ofAddress(dispatch_properties_changed$get(segment), scope);
}
/**
* {@snippet :
* void (*notify)(struct _GObject*,struct _GParamSpec*);
* }
*/
public interface notify {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(notify fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$625.const$4, fi, constants$13.const$4, scope);
}
static notify ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle notify$VH() {
return constants$625.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*notify)(struct _GObject*,struct _GParamSpec*);
* }
*/
public static MemorySegment notify$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$625.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*notify)(struct _GObject*,struct _GParamSpec*);
* }
*/
public static void notify$set(MemorySegment seg, MemorySegment x) {
constants$625.const$5.set(seg, x);
}
public static MemorySegment notify$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$625.const$5.get(seg.asSlice(index*sizeof()));
}
public static void notify$set(MemorySegment seg, long index, MemorySegment x) {
constants$625.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static notify notify(MemorySegment segment, Arena scope) {
return notify.ofAddress(notify$get(segment), scope);
}
/**
* {@snippet :
* void (*constructed)(struct _GObject*);
* }
*/
public interface constructed {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(constructed fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$626.const$0, fi, constants$13.const$1, scope);
}
static constructed ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle constructed$VH() {
return constants$626.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*constructed)(struct _GObject*);
* }
*/
public static MemorySegment constructed$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$626.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*constructed)(struct _GObject*);
* }
*/
public static void constructed$set(MemorySegment seg, MemorySegment x) {
constants$626.const$1.set(seg, x);
}
public static MemorySegment constructed$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$626.const$1.get(seg.asSlice(index*sizeof()));
}
public static void constructed$set(MemorySegment seg, long index, MemorySegment x) {
constants$626.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static constructed constructed(MemorySegment segment, Arena scope) {
return constructed.ofAddress(constructed$get(segment), scope);
}
public static VarHandle flags$VH() {
return constants$626.const$2;
}
/**
* Getter for field:
* {@snippet :
* unsigned long flags;
* }
*/
public static long flags$get(MemorySegment seg) {
return (long)constants$626.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* unsigned long flags;
* }
*/
public static void flags$set(MemorySegment seg, long x) {
constants$626.const$2.set(seg, x);
}
public static long flags$get(MemorySegment seg, long index) {
return (long)constants$626.const$2.get(seg.asSlice(index*sizeof()));
}
public static void flags$set(MemorySegment seg, long index, long x) {
constants$626.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static VarHandle n_construct_properties$VH() {
return constants$626.const$3;
}
/**
* Getter for field:
* {@snippet :
* unsigned long n_construct_properties;
* }
*/
public static long n_construct_properties$get(MemorySegment seg) {
return (long)constants$626.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* unsigned long n_construct_properties;
* }
*/
public static void n_construct_properties$set(MemorySegment seg, long x) {
constants$626.const$3.set(seg, x);
}
public static long n_construct_properties$get(MemorySegment seg, long index) {
return (long)constants$626.const$3.get(seg.asSlice(index*sizeof()));
}
public static void n_construct_properties$set(MemorySegment seg, long index, long x) {
constants$626.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static VarHandle pspecs$VH() {
return constants$626.const$4;
}
/**
* Getter for field:
* {@snippet :
* void* pspecs;
* }
*/
public static MemorySegment pspecs$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$626.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void* pspecs;
* }
*/
public static void pspecs$set(MemorySegment seg, MemorySegment x) {
constants$626.const$4.set(seg, x);
}
public static MemorySegment pspecs$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$626.const$4.get(seg.asSlice(index*sizeof()));
}
public static void pspecs$set(MemorySegment seg, long index, MemorySegment x) {
constants$626.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static VarHandle n_pspecs$VH() {
return constants$626.const$5;
}
/**
* Getter for field:
* {@snippet :
* unsigned long n_pspecs;
* }
*/
public static long n_pspecs$get(MemorySegment seg) {
return (long)constants$626.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* unsigned long n_pspecs;
* }
*/
public static void n_pspecs$set(MemorySegment seg, long x) {
constants$626.const$5.set(seg, x);
}
public static long n_pspecs$get(MemorySegment seg, long index) {
return (long)constants$626.const$5.get(seg.asSlice(index*sizeof()));
}
public static void n_pspecs$set(MemorySegment seg, long index, long x) {
constants$626.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static MemorySegment pdummy$slice(MemorySegment seg) {
return seg.asSlice(112, 24);
}
public static long sizeof() { return $LAYOUT().byteSize(); }
public static MemorySegment allocate(SegmentAllocator allocator) { return allocator.allocate($LAYOUT()); }
public static MemorySegment allocateArray(long len, SegmentAllocator allocator) {
return allocator.allocate(MemoryLayout.sequenceLayout(len, $LAYOUT()));
}
public static MemorySegment ofAddress(MemorySegment addr, Arena scope) { return RuntimeHelper.asArray(addr, $LAYOUT(), 1, scope); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy